Unlocking UI Wonders: Mastering JacpFX for Rich Clients
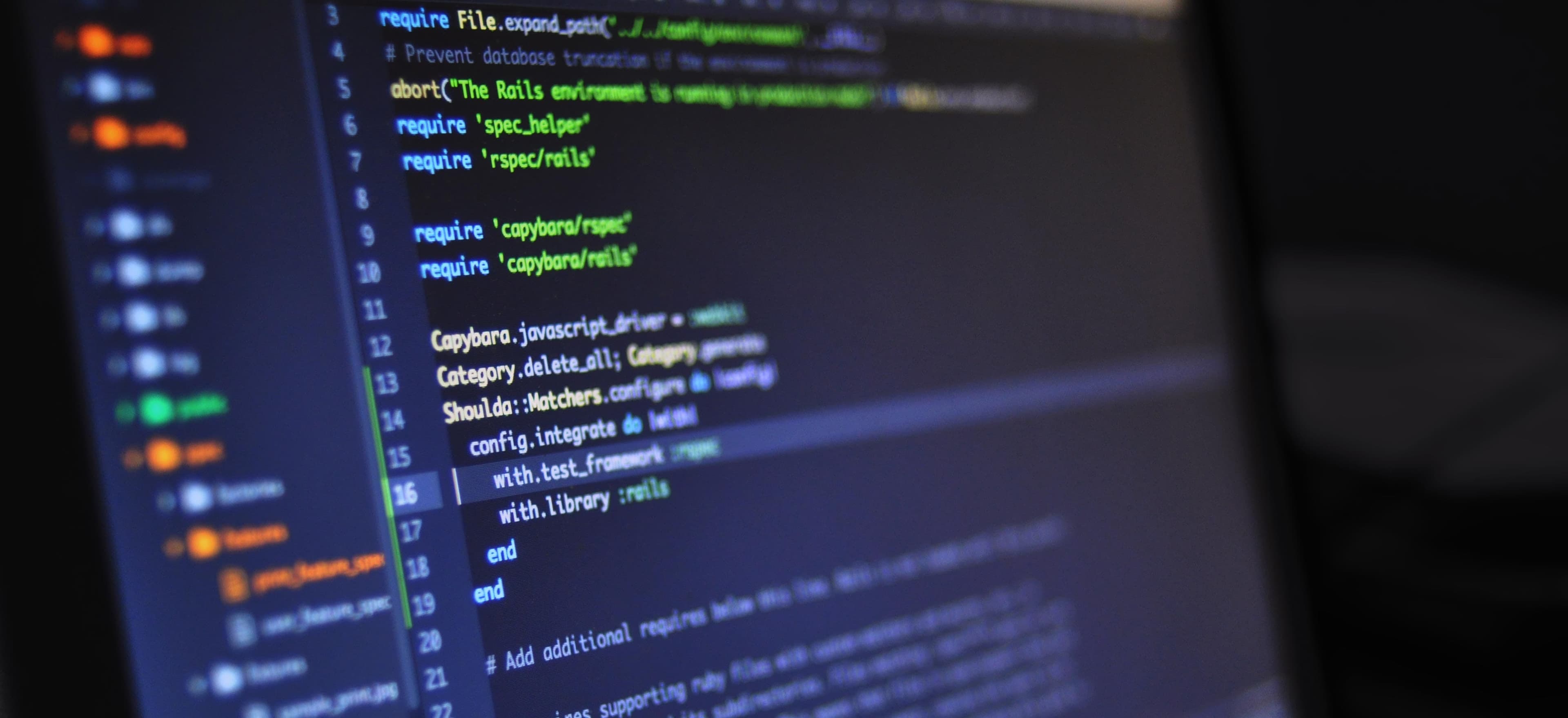
- Published on
Unlocking UI Wonders: Mastering JacpFX for Rich Clients
In the world of software development, creating a visually stunning, responsive, and user-friendly UI is crucial for capturing and retaining users. This is especially true for rich client applications, where user experience can make or break the success of a product. Java offers a powerful solution for building rich client applications in the form of JavaFX, a modern, feature-rich GUI toolkit. When it comes to mastering the art of building rich client applications, Java developers can leverage the JacpFX framework to unlock a world of UI wonders.
What is JacpFX?
JacpFX, short for Java Asynchronous Client Platform for JavaFX, is a framework designed to simplify the development of JavaFX applications by providing a structured, modular, and event-driven architecture. It promotes the creation of reusable UI components, encourages clean separation of concerns, and facilitates asynchronous communication between different parts of the application. With JacpFX, developers can build scalable, maintainable, and responsive rich client applications with ease.
Getting Started with JacpFX
To kick off our journey into the world of JacpFX, let's take a look at how to get started with setting up a basic JacpFX project. We will use Maven to manage our project and dependencies.
Setting up a Maven Project
Create a new Maven project in your favorite IDE, or if you prefer the command line, use the following Maven command:
mvn archetype:generate -DgroupId=com.example -DartifactId=jacpfx-demo -DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false
Once the project is created, navigate to the project directory and open the pom.xml
file. Add the JacpFX dependencies:
<dependencies>
<dependency>
<groupId>org.jacpfx</groupId>
<artifactId>jacpfx</artifactId>
<version>11.0.0</version>
</dependency>
<!-- Add other dependencies as needed -->
</dependencies>
Creating a Simple JacpFX Application
Next, let's create a simple JacpFX application to understand the basic structure and concepts. JacpFX follows the concept of perspectives, which are modular components that represent different views or parts of the application. Each perspective can contain its own UI components and logic.
Here's an example of a simple perspective class:
import javafx.scene.layout.VBox;
import org.jacpfx.api.annotations.Resource;
import org.jacpfx.api.component.Perspective;
public class MyPerspective implements Perspective<VBox> {
@Resource
private String message;
@Override
public VBox prepareNode() {
// Create UI components and return the root node
}
// Other methods and event handlers can be added here
}
In this example, MyPerspective
is a simple perspective that extends the Perspective
interface provided by JacpFX. It defines a method to prepare the UI node and can handle resources injected using the @Resource
annotation.
The Power of Asynchronous UI
One of the key features of JacpFX is its support for asynchronous UI operations. Asynchronous programming allows the UI to remain responsive while performing potentially long-running tasks such as network requests or heavy computations. JacpFX provides mechanisms for executing code asynchronously and updating the UI upon completion.
Let's take a look at an example of asynchronous UI operations in JacpFX:
import org.jacpfx.api.annotations.OnAsyncFXThread;
import org.jacpfx.api.annotations.OnAsyncUIThread;
import org.jacpfx.api.annotations.Resource;
import org.jacpfx.api.component.Perspective;
public class AsyncPerspective implements Perspective<VBox> {
@Resource
private DataService dataService;
@OnAsyncFXThread
public void fetchDataAsync() {
// Perform asynchronous data retrieval
// This method will execute on a background FX thread
}
@OnAsyncUIThread
public void updateUIWithData() {
// Update the UI with the retrieved data
// This method will execute on the UI thread
}
}
In this example, the fetchDataAsync
method is annotated with @OnAsyncFXThread
, indicating that it will run on a background FX thread. Once the data retrieval is complete, the updateUIWithData
method, annotated with @OnAsyncUIThread
, will be invoked to update the UI on the main UI thread.
Modular Architecture and Reusability
JacpFX promotes a modular architecture that encourages the creation of reusable UI components and perspectives. This modular approach facilitates code organization, maintenance, and extension. Perspectives can be composed and arranged to form complex UI layouts, promoting code reuse and separation of concerns.
Here's an example of composing perspectives in JacpFX:
import org.jacpfx.api.annotations.Resource;
import org.jacpfx.api.component.Perspective;
public class MainPerspective implements Perspective<BorderPane> {
@Resource
private MyPerspective myPerspective;
@Resource
private OtherPerspective otherPerspective;
@Override
public BorderPane prepareNode() {
// Create a layout and add the sub-perspectives to it
// Return the root node
}
}
In this example, the MainPerspective
composes the MyPerspective
and OtherPerspective
to form a complex UI layout. By leveraging the modular architecture, developers can create highly customizable and maintainable UI components.
Expanding Horizons with JacpFX
JacpFX empowers Java developers to craft rich client applications with elegant and responsive user interfaces. The framework's support for asynchronous UI, modular architecture, and event-driven programming makes it a formidable tool for building modern JavaFX applications. By mastering JacpFX, developers can unleash the full potential of Java for creating stunning and interactive rich client experiences.
In Conclusion, Here is What Matters
In conclusion, mastering JacpFX opens the door to a world of possibilities for building rich client applications. With its focus on asynchronous UI, modular architecture, and reusability, JacpFX provides the building blocks for creating visually captivating and highly responsive user interfaces. By incorporating JacpFX into their toolkit, Java developers can elevate their UI development skills and deliver rich client applications that leave a lasting impression on users.
So, if you're looking to take your JavaFX development to the next level, dive into the world of JacpFX and unlock the UI wonders that await.
Are you ready to embark on the journey of mastering JacpFX for rich clients? Let's dive in and create stunning UI experiences with Java!
Checkout our other articles