REST vs. Messaging in Microservices: Which Wins?
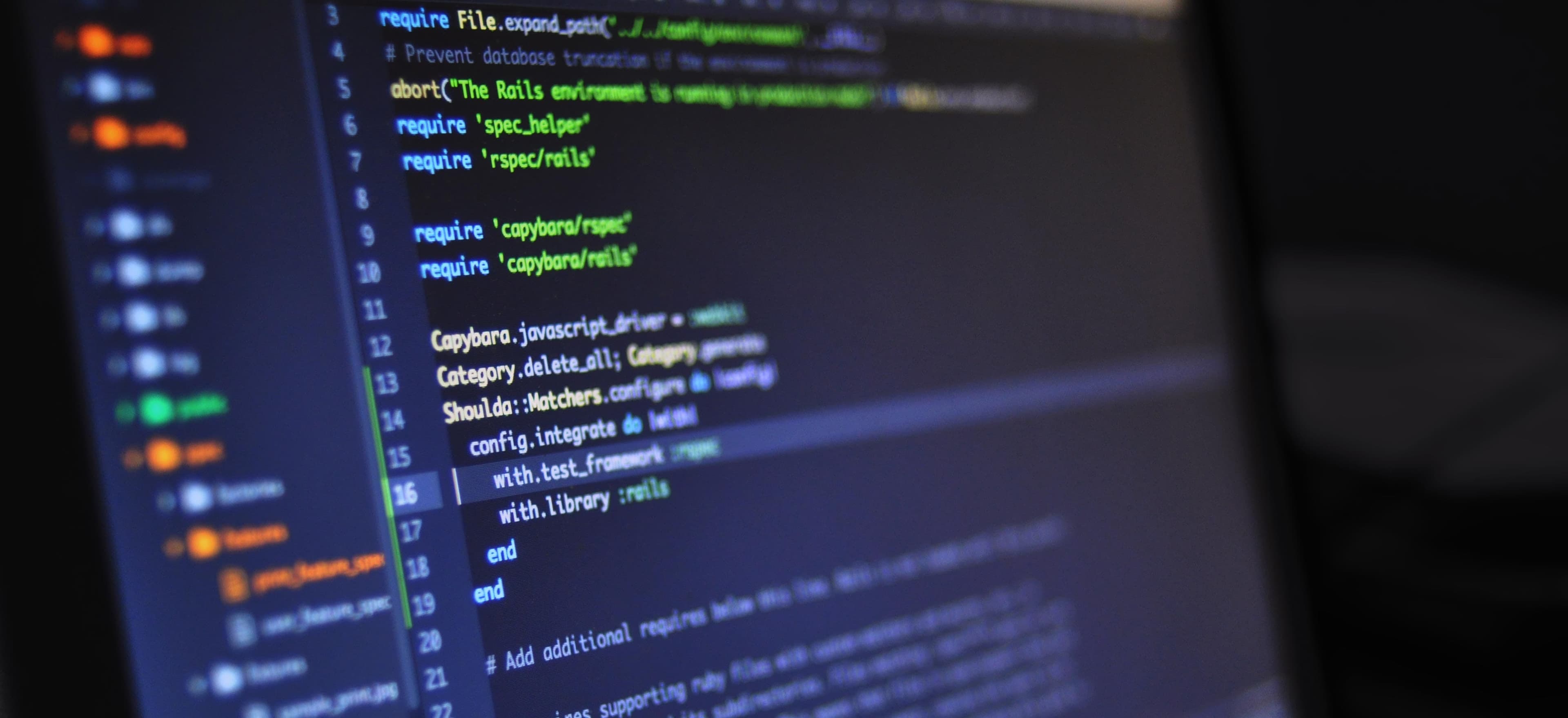
- Published on
REST vs. Messaging in Microservices: Which Wins?
When it comes to building and designing microservices, one of the key decisions developers face is choosing between REST (Representational State Transfer) and messaging protocols. Each approach has its own set of benefits and trade-offs, and the decision ultimately depends on the specific requirements of the application. In this article, we'll delve into the intricacies of both REST and messaging in the context of microservices, highlighting their strengths and weaknesses to determine which one comes out on top.
Understanding REST in Microservices
REST, an architectural style for building networked applications, leverages the HTTP protocol and is known for its simplicity and scalability. In the context of microservices, RESTful APIs serve as a means for communication between services. The key characteristics of REST include stateless communication, uniform interfaces, and the concept of resources.
Advantages of REST in Microservices
Simplicity and Ease of Use: RESTful APIs are intuitive and straightforward, making them easy to understand and use, which is beneficial in a microservices architecture where multiple services need to communicate effectively.
Interoperability: RESTful APIs can be accessed from any platform or programming language, promoting interoperability across different services and systems.
Caching: The use of HTTP caching mechanisms allows for improved performance and scalability, as responses can be cached at various levels within the infrastructure.
Drawbacks of REST in Microservices
Overfetching and Underfetching: One of the common criticisms of REST in microservices is the potential for overfetching or underfetching of data, where clients may receive more or less information than they actually need, leading to inefficiencies.
Chattiness: RESTful APIs can be chatty, resulting in multiple network requests to accomplish a single task, which can impact performance, especially in a microservices environment with numerous inter-service communications.
Exploring Messaging in Microservices
Messaging, on the other hand, involves asynchronous communication between services through message brokers such as RabbitMQ, Apache Kafka, or Amazon SQS. This approach facilitates decoupling between services, allowing them to communicate indirectly through the exchange of messages.
Advantages of Messaging in Microservices
Asynchronous Communication: Messaging enables asynchronous communication, which can improve system resilience, as services aren't directly dependent on each other's availability.
Scalability and Flexibility: Message queues allow for greater flexibility and scalability, as they can easily handle varying message loads and accommodate future service changes.
Event-Driven Architecture: Messaging supports event-driven architecture, enabling real-time processing and responsiveness to events and changes in the system.
Drawbacks of Messaging in Microservices
Complexity: Implementing and managing message brokers introduces complexity to the system, requiring additional infrastructure and maintenance.
Consistency and Transaction Management: Ensuring consistency and managing transactions in an asynchronous messaging environment can be challenging, especially when dealing with distributed transactions.
Making the Choice: REST vs. Messaging
So, which communication approach prevails in the realm of microservices? The answer lies in the characteristics and requirements of the specific application.
Use REST When:
-
Simple Request-Response Interactions: For straightforward interactions and when direct request-response communication suffices, RESTful APIs are a suitable choice due to their simplicity and ease of use.
-
Caching Requirements: If the application demands extensive caching of responses at various levels, REST can offer performance benefits through its built-in caching mechanisms.
-
Interoperability and Integration: When interoperability and integration with diverse platforms and technologies are crucial, RESTful APIs provide a standardized and accessible communication method.
// Example of a simple RESTful endpoint in a microservice
@RestController
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/users/{id}")
public ResponseEntity<User> getUserById(@PathVariable Long id) {
User user = userService.getUserById(id);
return ResponseEntity.ok(user);
}
}
The above code snippet showcases a simple RESTful endpoint for fetching a user by ID, utilizing the standard HTTP GET method.
Use Messaging When:
-
Event-Driven Architecture: In scenarios where event-driven processing and real-time responsiveness are crucial, messaging and asynchronous communication excel in handling event-driven workflows.
-
Loose Coupling: When aiming for loose coupling between services and the ability to scale and evolve independently, messaging promotes decoupling and flexibility in communication.
-
Handling Background Tasks: For dealing with background jobs, distributed workflows, and long-running processes, messaging can effectively manage asynchronous tasks.
// Example of publishing a message to a RabbitMQ exchange in a microservice
@Service
public class EventPublisherService {
@Autowired
private RabbitTemplate rabbitTemplate;
public void publishEvent(Object event) {
rabbitTemplate.convertAndSend("exchangeName", "routingKey", event);
}
}
In the provided code snippet, an event publisher service demonstrates the publication of messages to a RabbitMQ exchange for asynchronous processing.
Final Thoughts
In the dynamic landscape of microservices, both REST and messaging play integral roles in enabling communication and interaction between services. While REST excels in simplicity, ease of use, and interoperability, messaging offers advantages in terms of asynchronous communication, scalability, and event-driven processing.
Ultimately, the decision between REST and messaging in microservices hinges on the specific needs of the application, considering factors such as communication patterns, data exchange requirements, and system scalability. It's worth noting that hybrid approaches, which combine both RESTful APIs and messaging, can also be employed to harness the strengths of each method based on the context of the use case.
In conclusion, there is no one-size-fits-all answer to the "REST vs. Messaging" debate. Instead, understanding the nuances of each approach and aligning them with the unique demands of the microservices architecture is pivotal in making an informed decision that best serves the functionality, performance, and scalability of the application.
For further exploration of microservices communication patterns and best practices, refer to Martin Fowler's article on Microservices and InfoQ's in-depth analysis of microservices communication strategies.