Solving Retail Woes with Store Integration Architecture
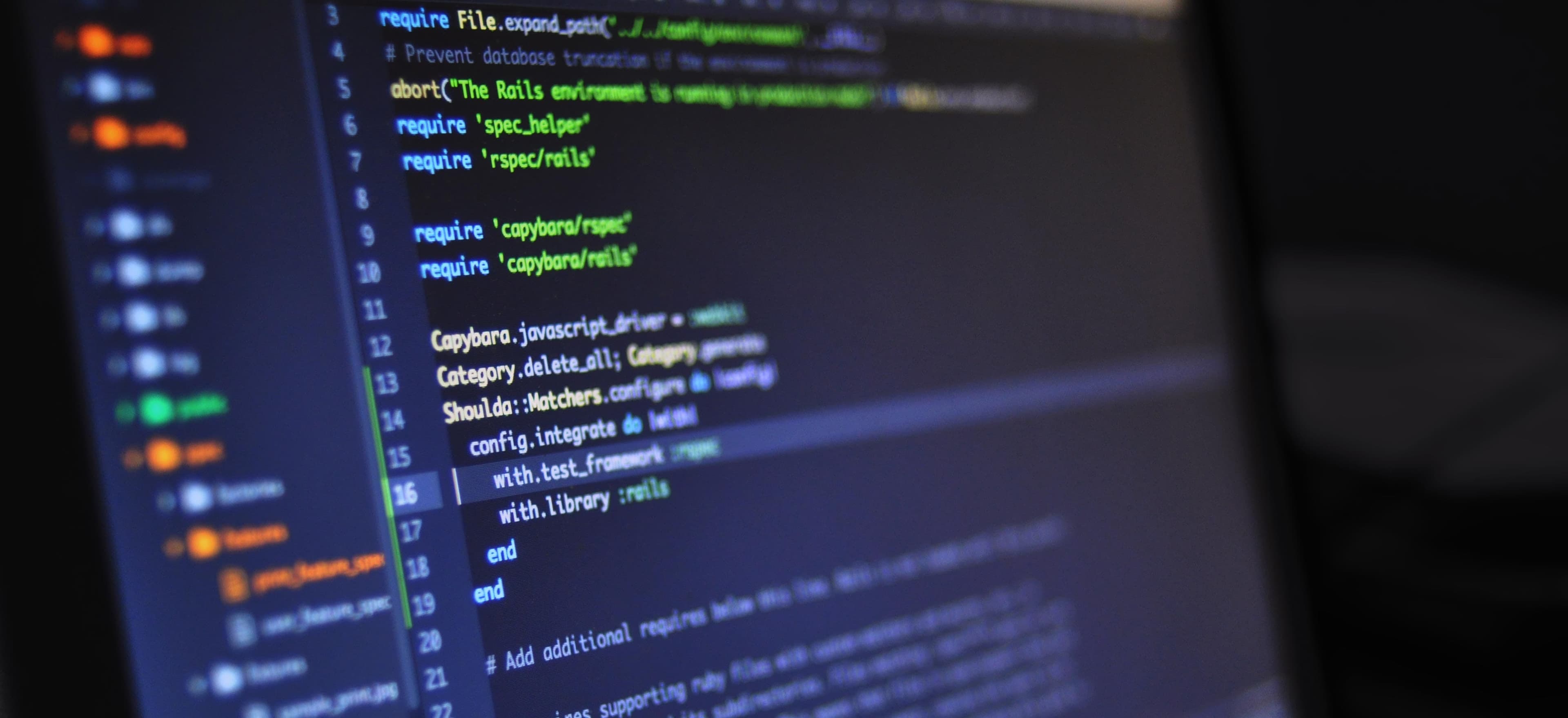
- Published on
Solving Retail Woes with Store Integration Architecture
In today's retail landscape, delivering a seamless shopping experience is no longer a competitive advantage, but a necessity. To meet customer demands, retailers need to integrate their online and offline stores effectively. This is where Store Integration Architecture comes into play. In this article, we will explore how Java can be leveraged to build a robust and scalable store integration architecture that addresses the retail woes and enhances the overall shopping experience.
The Retail Challenge
Retailers often face challenges such as inventory discrepancies between online and offline stores, fragmented customer data, and inconsistent pricing and promotions. These challenges can lead to a disjointed customer experience, resulting in dissatisfied customers and lost sales opportunities. To tackle these issues, retailers are turning to Store Integration Architecture.
What is Store Integration Architecture?
Store Integration Architecture is a set of practices, patterns, and technologies that enable seamless integration between online and offline retail channels. It allows retailers to synchronize inventory, customer data, pricing, and promotions across different touchpoints, providing a unified shopping experience for customers.
Java's Role in Store Integration Architecture
Java, with its strong emphasis on scalability, flexibility, and robustness, is well-suited for building the core components of Store Integration Architecture. Let's delve into some key aspects of how Java can be leveraged to address the challenges faced by retailers.
Handling Asynchronous Communication
One of the fundamental requirements of Store Integration Architecture is handling asynchronous communication between various systems. Java provides robust support for asynchronous programming through features like CompletableFuture and Executor framework. By using Java's CompletableFuture, retailers can manage concurrent tasks more efficiently, leading to improved performance and responsiveness in handling real-time inventory updates and order processing.
CompletableFuture<Void> updateInventoryTask = CompletableFuture.runAsync(() -> {
// Code to update inventory
});
CompletableFuture<Void> processOrderTask = CompletableFuture.runAsync(() -> {
// Code to process order
});
CompletableFuture<Void> promotionUpdateTask = CompletableFuture.runAsync(() -> {
// Code to update promotions
});
CompletableFuture<Void> allTasks = CompletableFuture.allOf(updateInventoryTask, processOrderTask, promotionUpdateTask);
allTasks.join();
In the above example, CompletableFuture is used to perform three independent tasks concurrently, and then they are combined using allOf
to ensure that all tasks are completed before proceeding.
Building Robust APIs
Java's mature ecosystem, coupled with frameworks like Spring and JAX-RS, empowers retailers to build robust and scalable APIs for seamless data exchange between online and offline stores. By implementing RESTful APIs in Java, retailers can expose endpoints for inventory synchronization, customer data retrieval, and promotion updates, enabling smooth communication between disparate systems.
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import javax.ws.rs.core.MediaType;
@Path("/inventory")
public class InventoryResource {
@GET
@Produces(MediaType.APPLICATION_JSON)
public List<Item> getInventory() {
// Code to retrieve inventory data
}
}
The code snippet demonstrates a simple InventoryResource class using JAX-RS to expose an endpoint for retrieving inventory data in a JSON format.
Event-Driven Architecture
Java's support for event-driven architecture, combined with frameworks like Apache Kafka or RabbitMQ, facilitates real-time data synchronization and event processing. By leveraging Java's event-driven capabilities, retailers can ensure that inventory updates, order placements, and promotional changes trigger appropriate actions across all channels, maintaining consistency and accuracy in the shopping experience.
public void onInventoryUpdate(Item item) {
// Code to process inventory update event
}
public void onOrderPlacement(Order order) {
// Code to process order placement event
}
public void onPromotionChange(Promotion promotion) {
// Code to process promotion change event
}
In the above example, event handler methods are defined to react to specific events, ensuring that the respective actions are taken in response to inventory updates, order placements, and promotional changes.
The Last Word
In conclusion, Java serves as a potent tool for addressing the complex challenges faced by retailers in integrating their online and offline stores. By leveraging Java's strengths in handling asynchronous communication, building robust APIs, and implementing event-driven architecture, retailers can establish a Store Integration Architecture that fosters a cohesive shopping experience for customers. As retail continues to evolve, the role of Java in shaping seamless store integration architecture is set to become even more pivotal.
Implementing Store Integration Architecture is not without its challenges and complexities, but the benefits of unifying the retail experience cannot be overstated. By harnessing the power of Java, retailers can position themselves at the forefront of delivering unparalleled shopping experiences in today's omnichannel retail landscape.
By adopting a well-architected Store Integration Architecture, retailers can address the pressing needs of their customers, establish a competitive edge, and lay the foundation for sustained success in the dynamic realm of retail.
Read more about Store Integration Architecture