Netty 4: How to Fix HTTP Content Compression Issues
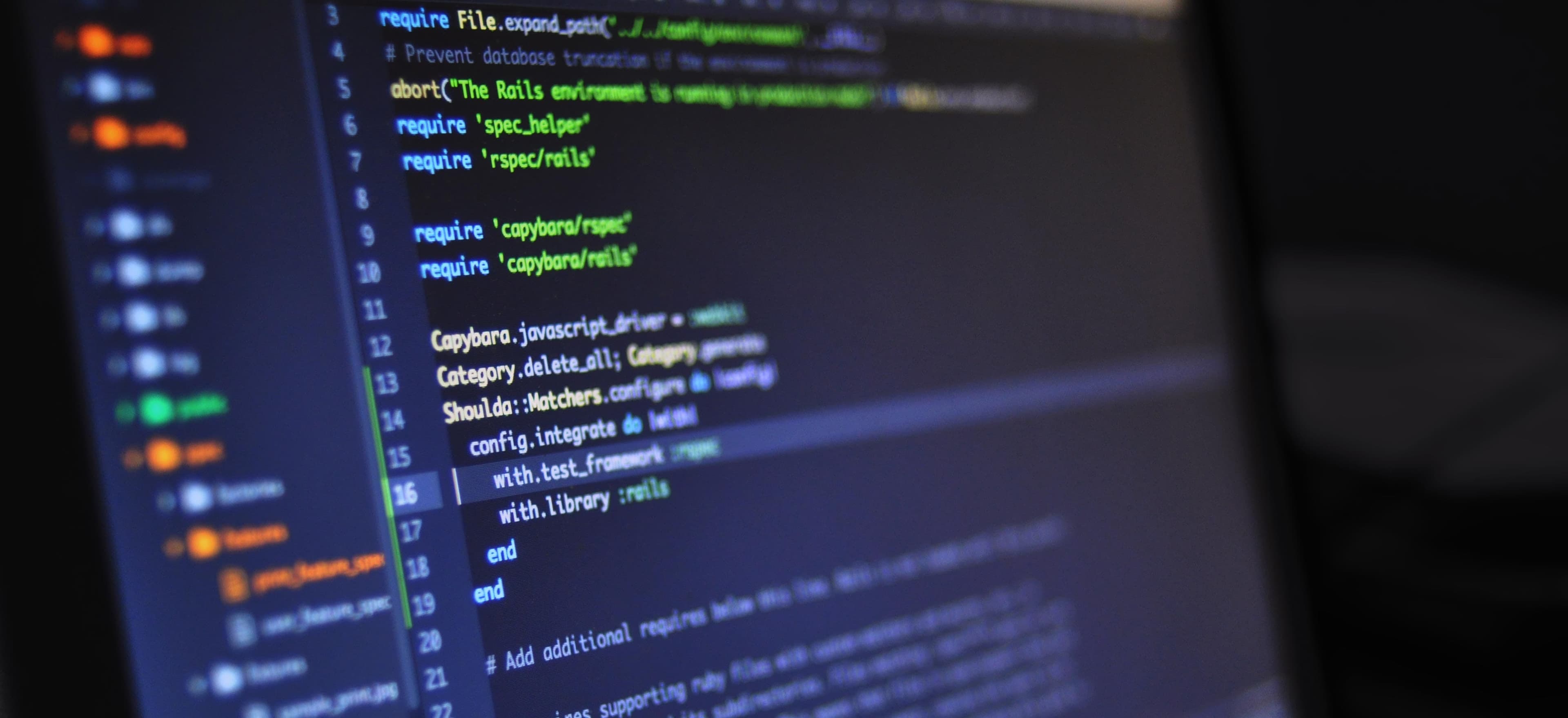
- Published on
Optimizing HTTP Content Compression with Netty 4
In today's digital landscape, optimizing web content delivery is crucial to ensure a seamless user experience. One of the key techniques for improving web performance is HTTP content compression. By reducing the size of resources sent over the network, content compression can significantly enhance page load times and decrease bandwidth usage.
In this article, we'll delve into the realm of HTTP content compression with Netty 4, a high-performance networking library for building low-level, high-throughput network applications in Java. We'll explore common issues related to HTTP content compression and present effective solutions to fix them, ensuring smooth and efficient compression of resources in your Netty-powered applications.
Understanding the Importance of HTTP Content Compression
HTTP content compression serves as a fundamental optimization technique for web servers and clients. By compressing resources such as HTML, CSS, JavaScript, and other content types before transmitting them over the network, the payload size is reduced, resulting in faster page load times and decreased bandwidth consumption. This is especially crucial for mobile devices and users with limited network connectivity.
Netty 4 provides robust support for handling HTTP requests and responses, making it an ideal platform for implementing HTTP content compression strategies. However, there are common pitfalls and issues that developers may encounter when configuring and utilizing HTTP content compression in Netty-based applications. Let's address these issues and explore effective remedies to ensure seamless content compression in your Netty 4 projects.
Common Issues with HTTP Content Compression in Netty 4
Incorrect Configuration of Compression Handler
One prevalent issue in Netty 4 applications is the incorrect configuration of the compression handler for HTTP content. This can lead to ineffective compression or even failure to compress resources, resulting in suboptimal performance.
Improper Handling of Content Types
Another common issue is the improper handling of content types for compression. If certain content types are not appropriately configured for compression, the resources of those types will bypass compression, impacting overall optimization efforts.
Gzip Compression Compatibility
Gzip is one of the most widely used compression algorithms for HTTP content compression. However, ensuring compatibility and proper handling of Gzip-compressed content in Netty 4 applications can be challenging and may lead to compatibility issues with various clients and browsers.
Fixing HTTP Content Compression Issues in Netty 4
Now, let's delve into actionable solutions to rectify the aforementioned issues and optimize HTTP content compression in Netty 4.
Proper Configuration of Compression Handler
To address the issue of incorrect configuration of the compression handler, it is crucial to ensure that the compression handler is appropriately configured in the Netty pipeline for HTTP requests and responses. The following code snippet illustrates how to configure the HTTP content compression handler in Netty 4:
ChannelPipeline pipeline = Channels.pipeline();
// Other handlers in the pipeline
pipeline.addLast("codec", new HttpServerCodec());
pipeline.addLast("compressor", new HttpContentCompressor());
// Other handlers in the pipeline
In this example, the HttpContentCompressor
is added to the pipeline to enable compression of HTTP content. By properly configuring the compression handler in the pipeline, Netty 4 can effectively compress outgoing HTTP responses and decompress incoming requests, enhancing overall network performance.
Handling Content Types for Compression
To address the improper handling of content types for compression, developers must explicitly specify the content types that should be compressed. This can be achieved by configuring the HttpContentCompressor
with appropriate parameters to include specific content types for compression. Below is an example of how to configure the HttpContentCompressor
to handle specific content types for compression:
HttpContentCompressor compressor = new HttpContentCompressor();
compressor.setIncludedContentTypes(
Arrays.asList("text/plain", "text/html", "text/css", "application/javascript")
);
pipeline.addLast("compressor", compressor);
By specifying the content types to be included for compression, developers can ensure that relevant resources are effectively compressed, thereby optimizing web performance and user experience.
Gzip Compression Compatibility
Ensuring Gzip compression compatibility in Netty 4 applications involves addressing potential issues related to Gzip-compressed content. Developers should validate Gzip compatibility across various clients and browsers to ensure seamless decompression of Gzip-compressed content. Additionally, considering the use of alternative compression algorithms such as Brotli can improve compression efficiency and compatibility.
Key Takeaways
In conclusion, optimizing HTTP content compression in Netty 4 is critical for enhancing web performance and delivering a superior user experience. By addressing common issues such as incorrect configuration of compression handlers, improper handling of content types, and Gzip compression compatibility, developers can ensure effective content compression in their Netty-powered applications, resulting in faster page load times and reduced network bandwidth usage.
By implementing the recommended solutions and best practices discussed in this article, developers can elevate the performance of their Netty 4 applications, solidifying their position in the realm of high-performance and efficient network application development.
For further exploration of Netty 4 and HTTP content compression, refer to the Netty documentation. Happy coding!