Solving Common MapHandler Issues in OpenMap Apps
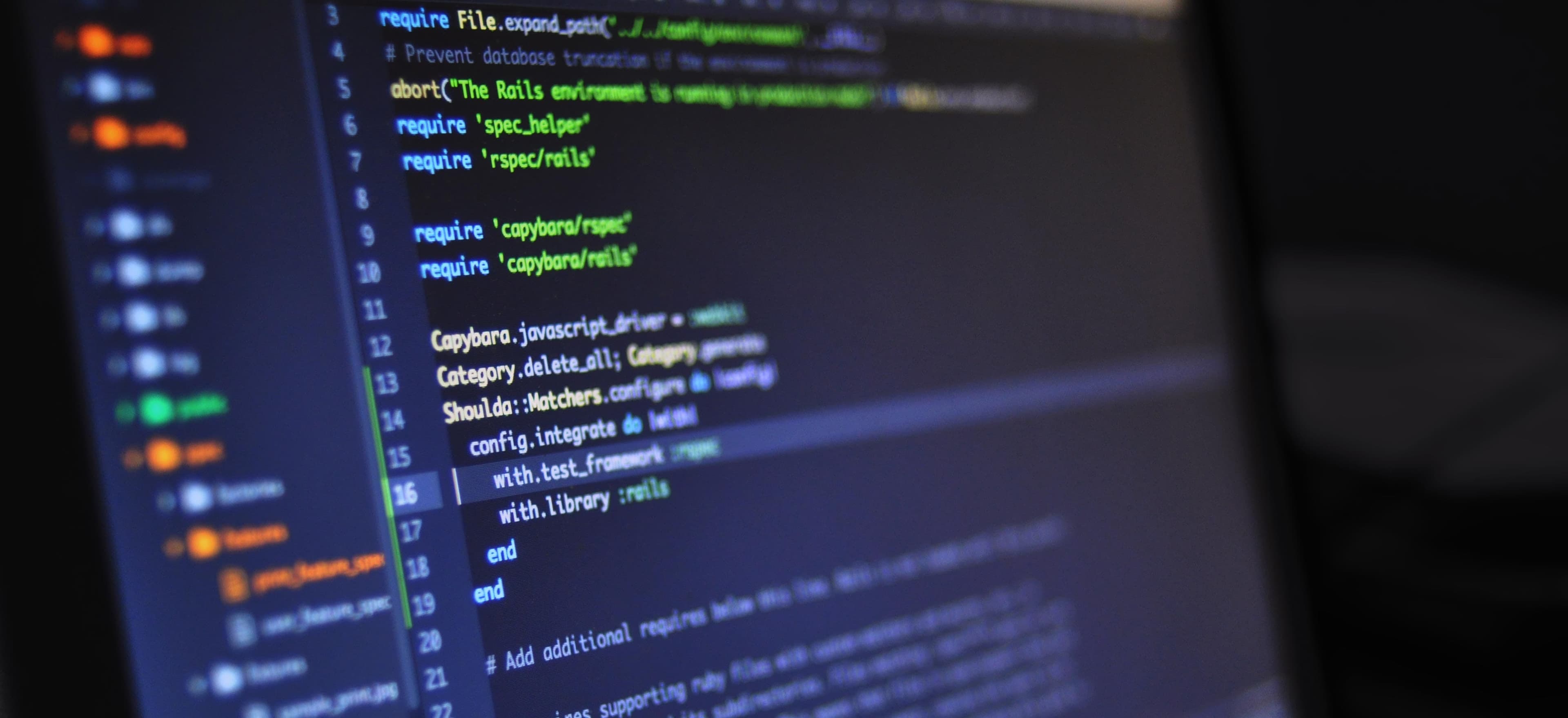
- Published on
Solving Common MapHandler Issues in OpenMap Apps
As Java developers, we often encounter challenges when working with geospatial data and mapping applications. OpenMap, a powerful and flexible Java toolkit for building mapping applications, is no exception. In this blog post, we will explore some common issues with MapHandler in OpenMap apps and discuss how to solve them effectively.
Understanding the MapHandler in OpenMap
Before addressing the common issues, let's first understand the role of MapHandler in OpenMap. The MapHandler is a crucial component that facilitates user interaction with the map, including panning, zooming, and selecting map objects. It interprets user input and transforms it into map operations, playing a pivotal role in delivering a smooth and intuitive mapping experience.
Common MapHandler Issues
1. Unresponsive Map Interaction
One of the most prevalent issues is unresponsive map interaction, where user input (e.g., dragging to pan the map or using the scroll wheel to zoom) does not produce the expected map changes. This can be frustrating for users and indicates a flaw in the MapHandler configuration.
2. Inconsistent Event Handling
In some cases, the MapHandler may exhibit inconsistent event handling, leading to unexpected behavior when users interact with the map. This could be caused by improper event listener setup or conflicts between different event handlers within the application.
3. Custom Gesture Support
Integrating custom gestures, such as double-tap or pinch-to-zoom, into the MapHandler's functionality can be challenging. OpenMap provides standard map interaction gestures, but extending these to support custom gestures requires careful implementation to ensure seamless integration.
Resolving the Issues
1. Checking MapHandler Configuration
When encountering unresponsive map interaction, start by reviewing the MapHandler configuration. Ensure that the MapHandler instance is properly initialized and connected to the map component. Verify that the event listeners are correctly registered to capture user input, and that the handler has been set up to process these events effectively.
Example:
MapHandler mapHandler = new BasicMapHandler();
mapHandler.setMap(mapBean.getMap());
mapBean.addMouseListener(mapHandler);
mapBean.addMouseMotionListener(mapHandler);
mapBean.addMouseWheelListener(mapHandler);
In this example, we create a BasicMapHandler
instance, connect it to the map component, and register the necessary mouse listeners to enable interactive map manipulation.
2. Event Listener Consolidation
To address inconsistent event handling, consolidate event listeners to prevent conflicts and ensure that all user input is directed to the MapHandler appropriately. Avoid registering multiple listeners that may interfere with each other, and centralize event processing within the MapHandler to maintain a consistent behavior across different user interactions.
Example:
mapBean.removeMouseListener(previousMouseListener);
mapBean.removeMouseMotionListener(previousMouseMotionListener);
mapBean.removeMouseWheelListener(previousMouseWheelListener);
MapHandler mapHandler = new BasicMapHandler();
// Add the mapHandler as the single listener for all mouse events
mapBean.addMouseListener(mapHandler);
mapBean.addMouseMotionListener(mapHandler);
mapBean.addMouseWheelListener(mapHandler);
In this example, we remove any previous mouse listeners and consolidate event handling under the BasicMapHandler
instance to ensure uniform processing of user input.
3. Implementing Custom Gesture Support
When incorporating custom gestures into the MapHandler, it is essential to extend the functionality of the handler to recognize and respond to these gestures accordingly. This involves capturing the specific input patterns associated with custom gestures and mapping them to appropriate map operations, such as zooming or tilting the map view.
Example:
public class CustomMapHandler extends BasicMapHandler {
public void handleCustomGesture(GestureEvent gestureEvent) {
if (gestureEvent.isDoubleTap()) {
// Perform custom double-tap action
} else if (gestureEvent.isPinchToZoom()) {
// Handle pinch-to-zoom gesture
}
// Additional custom gesture handling
}
}
In this example, we extend the BasicMapHandler
to create a CustomMapHandler
that includes specific methods for handling custom gestures, such as double-tap and pinch-to-zoom, enhancing the MapHandler's capabilities.
Key Takeaways
In conclusion, the MapHandler in OpenMap plays a pivotal role in delivering a seamless and interactive mapping experience. By understanding common issues related to MapHandler functionality and implementing effective solutions, developers can ensure that their OpenMap applications provide smooth and intuitive map interactions for end users.
Remember to thoroughly review the MapHandler configuration, consolidate event listeners, and extend the handler to support custom gestures where necessary. These practices will contribute to the robustness and usability of mapping applications built with OpenMap.
With these strategies in place, developers can overcome MapHandler challenges and create compelling mapping solutions that meet user expectations and deliver an exceptional user experience.
For further information on OpenMap and Java mapping applications, check out the official OpenMap documentation and the Java GIS community.
Happy mapping!