Simplify Java: How to Create Zero-Dependency Native Apps
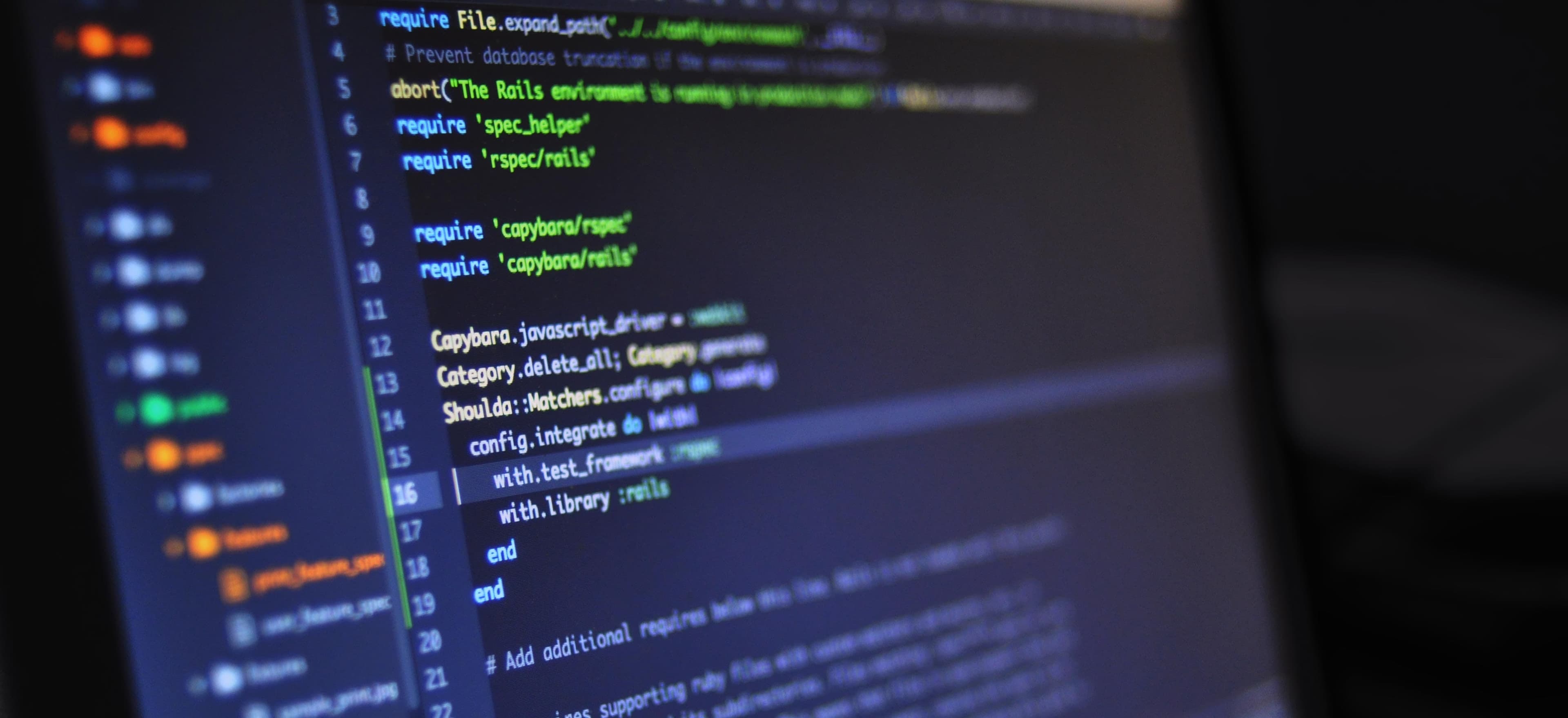
- Published on
Simplify Java: How to Create Zero-Dependency Native Apps
When it comes to creating native applications in Java, developers often face the challenge of managing dependencies. However, by employing certain techniques and tools, it's possible to streamline the process and build native apps that are free from external dependencies. In this article, we'll explore how to achieve this goal using Java.
Understanding Zero-Dependency Native Apps
Zero-dependency native apps refer to applications that can run independently without relying on external libraries or frameworks. This approach offers several advantages, including improved portability, reduced risk of compatibility issues, and enhanced security.
In the Java ecosystem, achieving zero-dependency involves minimizing the reliance on third-party libraries and ensuring that the application can be executed on any system with a compatible Java Runtime Environment (JRE) installed.
Leveraging JLink for Modularization
One of the key tools for creating zero-dependency native apps in Java is JLink. JLink, introduced in Java 9, allows developers to create custom runtime images that contain only the platform modules required by the application. This enables the generation of a standalone runtime that includes the necessary modules and no additional dependencies.
Let's consider a simple example where we have a Java application consisting of multiple modules. We can use JLink to create a custom runtime image containing only the modules necessary for our application.
// module-info.java
module com.example.app {
requires javafx.controls;
exports com.example.app;
}
In this example, we define a module com.example.app
that requires the javafx.controls
module. When it comes to creating the runtime image, JLink will include the required modules along with the modules they depend on, effectively creating a self-contained executable with zero external dependencies.
Using JPackage for Packaging
Java 14 introduced JPackage, a tool that complements JLink by allowing developers to package their Java applications as native installers. This means that developers can distribute their zero-dependency native apps in the form of platform-specific installation packages, such as MSI for Windows, PKG for macOS, and DEB/RPM for Linux.
By leveraging JPackage, developers can simplify the deployment of their applications while ensuring that end-users can easily install and run the apps without worrying about missing dependencies.
Simplifying Building and Deployment with Gradle
To streamline the process of building and deploying zero-dependency native apps, Gradle provides excellent support through plugins such as application
and jlink
. By using these plugins, developers can automate the creation of custom runtime images and native installers as part of the build process.
Let's see how Gradle can be used to achieve this:
plugins {
id 'application'
id 'org.openjfx.javafxplugin' version '0.0.9'
}
application {
mainModule = 'com.example.app'
mainClass = 'com.example.app.Main'
}
javafx {
version = "15"
modules = [ 'javafx.controls' ]
}
jlink {
launcher {
name = 'myapp'
}
}
In this Gradle build file, we define the application plugin for creating a Java application, specify the main module and class, configure the JavaFX plugin for the required modules, and set up the JLink plugin for generating the custom runtime image with a designated launcher.
By integrating Gradle with JLink and JPackage, developers can effectively automate the entire process of building, modularizing, and packaging zero-dependency native apps, thereby simplifying the development workflow.
Embracing Lightweight Libraries and Modules
In addition to leveraging JLink and JPackage, another approach to creating zero-dependency native apps involves utilizing lightweight libraries and modules that minimize external dependencies. For instance, by choosing lightweight alternatives to commonly used libraries, developers can reduce the overall reliance on external components.
Furthermore, modularizing the application architecture and adopting a modular programming approach can help isolate dependencies and create a more cohesive and maintainable codebase. This approach aligns with the principles of modularity introduced in Java 9, enabling developers to build zero-dependency native apps with improved encapsulation and flexibility.
Closing the Chapter
In conclusion, creating zero-dependency native apps in Java is achievable through the strategic use of tools like JLink and JPackage, the adoption of modularized architecture, and the integration of lightweight libraries. By embracing these techniques, developers can simplify the development, deployment, and maintenance of native applications while ensuring a lean and self-contained deployment package.
In the ever-evolving landscape of application development, the ability to create zero-dependency native apps represents a significant advancement, offering enhanced portability, security, and ease of use. As Java continues to evolve, developers can expect further improvements and tools to support the seamless creation of native applications with minimal external dependencies.
Now that you understand how to create zero-dependency native apps in Java, you can explore further by visiting OpenJDK and Gradle to delve deeper into the tools and techniques discussed in this article.