Fixing Declarative Linking Issues in Jersey 2.9+
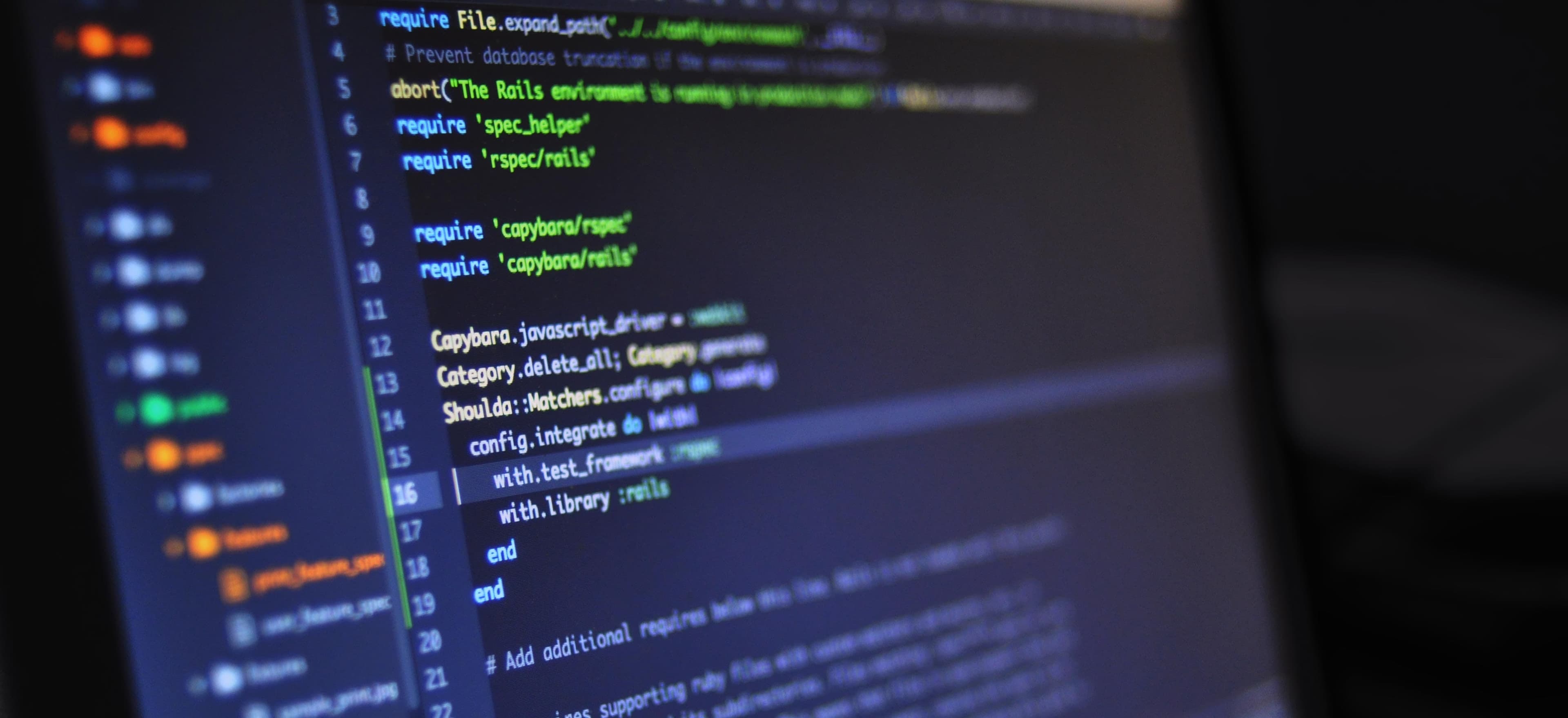
- Published on
Fixing Declarative Linking Issues in Jersey 2.9+
When working with Jersey, the declarative linking issue can often become a roadblock. However, with the release of Jersey 2.9 and above, resolving these issues has become easier. In this post, we will delve into the core of this problem and discuss how to tackle it effectively.
Understanding Declarative Linking
Declarative linking is a powerful concept within the RESTful paradigm. It enables developers to define resource relationships and allows for seamless navigation between different parts of the API. However, when it comes to implementation, issues related to generating and resolving these links can arise.
The Problem
In Jersey 2.9+, developers faced challenges in generating and resolving declarative links due to changes in the underlying framework. This led to issues such as broken links or incorrect URI generation, impacting the overall functionality and user experience of the API.
The Solution: UriBuilder
To address declarative linking issues in Jersey 2.9+, the UriBuilder
class comes to the rescue. By leveraging UriBuilder
, we can enhance link generation and resolve any existing issues.
Generating Links
Let's first look at an example of how to use UriBuilder
to generate links:
UriBuilder builder = UriBuilder.fromResource(ResourceClass.class);
URI uri = builder.path(ResourceClass.class, "getResource").build();
In the above example, we create a UriBuilder
instance using the fromResource
method, which takes the resource class as a parameter. We then append the path using the path
method and build the URI. This allows for dynamic and accurate link generation.
Resolving Links
Now, let's explore how UriBuilder
can help in resolving links:
UriBuilder builder = UriBuilder.fromUri("http://example.com/base");
URI uri = builder.path("/resource/{id}").resolveTemplate("id", 123).build();
In this snippet, we start with a base URI and then use resolveTemplate
to replace the placeholders with actual values, resulting in the resolved URI. This ensures that the links are accurately resolved, eliminating any discrepancies.
By utilizing UriBuilder
for both link generation and resolution, we can effectively mitigate declarative linking issues in Jersey 2.9+.
Leveraging HATEOAS
Hypermedia as the Engine of Application State (HATEOAS) is a fundamental principle in RESTful API design. It allows clients to navigate the API dynamically by following links provided by the server. In the context of declarative linking, HATEOAS plays a pivotal role in ensuring that the links are consistent and actionable.
Implementing HATEOAS
To implement HATEOAS in Jersey 2.9+, we can utilize libraries such as Spring HATEOAS or implement custom link generation logic. These libraries offer solutions for building and serving hypermedia-driven REST APIs, empowering developers to incorporate HATEOAS principles seamlessly.
Moreover, by incorporating HATEOAS, we can enhance the discoverability of resources and improve the overall navigational experience for API consumers.
Key Takeaways
In conclusion, declarative linking issues in Jersey 2.9+ can be effectively addressed by leveraging UriBuilder
for link generation and resolution. Furthermore, embracing HATEOAS principles can elevate the functionality and usability of the API, providing a more seamless experience for clients.
By understanding the core concepts and employing the right tools and principles, developers can overcome declarative linking challenges and build robust, navigable APIs in Jersey 2.9+.
For more in-depth understanding of Jersey 2.9+ and RESTful API best practices, refer to the official Jersey documentation.
So, go ahead, harness the power of UriBuilder
and HATEOAS to conquer declarative linking issues in Jersey 2.9+ and elevate your API development game!