Unlocking Seamless Migration: From Monolith to Microservice
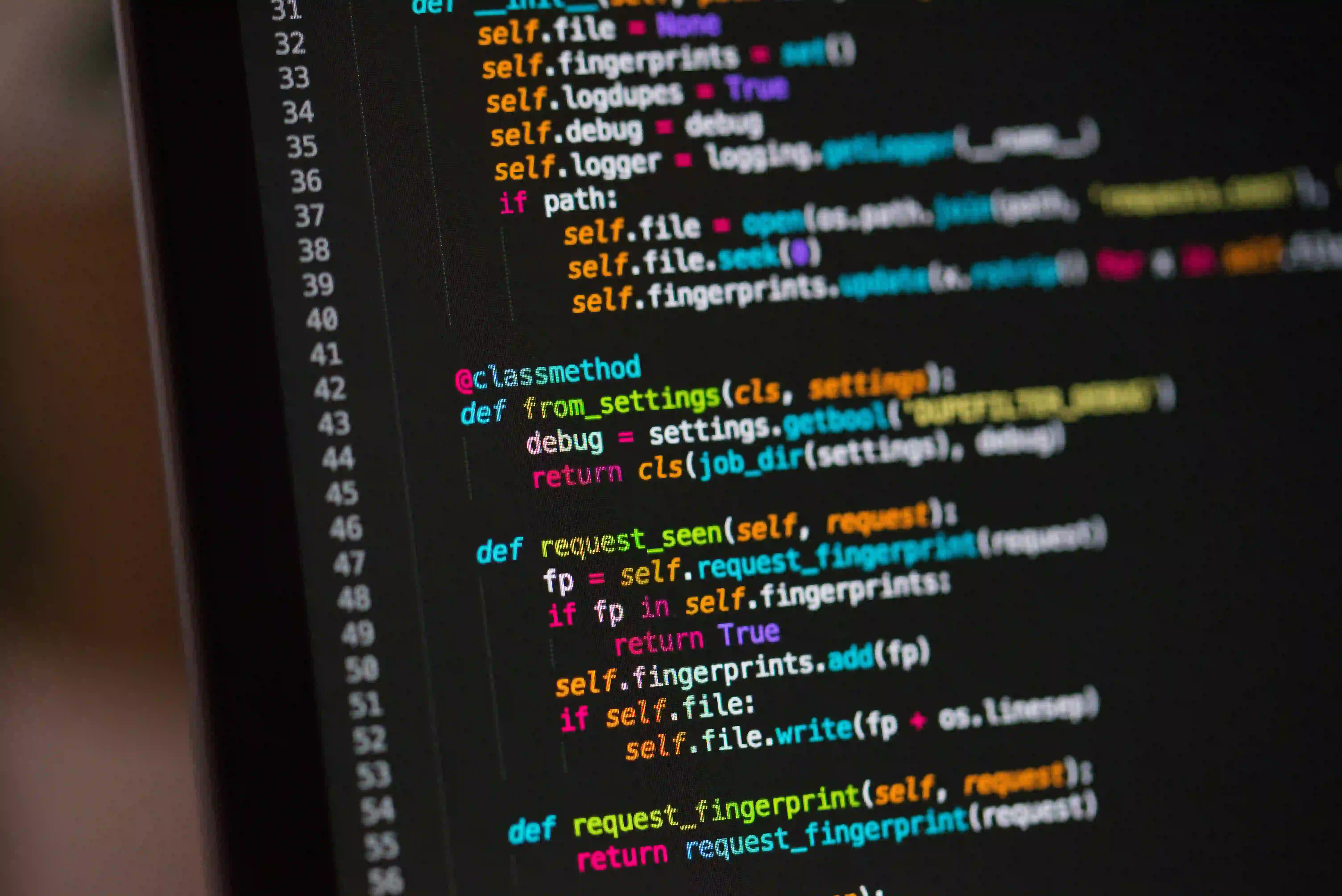
Unlocking Seamless Migration: From Monolith to Microservice
In the evolving landscape of software architecture, the shift from monolithic to microservices has become increasingly popular for organizations seeking to improve scalability, maintainability, and agility. Java, with its robust features and wide adoption, plays a pivotal role in this transition.
The Monolith vs. Microservice Conundrum
Monolithic Architecture
In traditional monolithic architecture, the entire application is developed, deployed, and managed as a single unified unit. While this approach initially offers simplicity, it often leads to challenges in scaling, deploying updates, and maintaining the entire codebase.
Microservices Architecture
On the other hand, the microservices architecture advocates breaking down the application into smaller, independent, and loosely coupled services. Each service is responsible for a specific functionality and can be developed, deployed, and scaled independently.
Leveraging Java for Microservices
Strong Ecosystem
Java boasts a vast ecosystem of libraries, frameworks, and tools that perfectly align with the microservices paradigm. Popular frameworks like Spring Boot, Micronaut, and Quarkus provide robust support for building microservices in Java.
Platform Independence
With the "write once, run anywhere" principle, Java ensures the portability of microservices across different environments, making it an ideal choice for modern, distributed architectures.
Mature Tooling
Java comes equipped with mature and battle-tested tools for monitoring, logging, testing, and managing microservices. Tools like Docker, Kubernetes, and Prometheus seamlessly integrate with Java-based microservices, facilitating efficient management and monitoring capabilities.
Strategies for Migration
Strangler Pattern
The Strangler Pattern involves gradually replacing parts of the monolith with microservices, thereby "strangling" the monolith over time. This incremental approach allows for a smoother transition and reduces the risk associated with a wholesale migration.
Domain-Driven Design (DDD)
Adopting DDD principles helps to identify bounded contexts within the monolith, paving the way for the extraction of independently deployable microservices. By focusing on domain logic and boundaries, DDD facilitates a more granular and strategic approach to migration.
API Gateway
Introducing an API gateway acts as a mediator between clients and microservices, enabling gradual migration without disrupting the existing client interfaces. This approach also offers opportunities for implementing cross-cutting concerns such as security, logging, and monitoring.
Code Examples: Transforming Monolithic Java Code to Microservices
Monolithic Code Snippet
public class MonolithicService {
public void processOrder(Order order) {
// Business logic for processing orders
}
}
Refactored Microservice Code
@RestController
public class OrderService {
@PostMapping("/orders")
public ResponseEntity<String> processOrder(@RequestBody Order order) {
// Business logic for processing orders
return ResponseEntity.ok("Order processed successfully");
}
}
In the refactored code, the monolithic service has been transformed into a microservice using Spring Boot. The @RestController
annotation enables the service to handle HTTP requests, while the @PostMapping
annotation specifies the endpoint for processing orders.
Commentary:
- The transformation illustrates the shift from a monolithic, internally invoked method to a microservice with a defined HTTP endpoint.
- This refactoring enables decoupling and independent scalability of the order processing functionality.
Key Considerations for Successful Migration
Testing Strategy
Implementing comprehensive unit, integration, and end-to-end tests becomes imperative during migration. Tools like JUnit, Mockito, and WireMock assist in validating the behavior and interactions of individual microservices.
Continuous Integration/Continuous Deployment (CI/CD)
Leveraging CI/CD pipelines aids in automating the build, test, and deployment processes, ensuring rapid feedback and deployment of microservices.
Monitoring and Observability
Adopting tools such as Jaeger, Zipkin, and Grafana for distributed tracing, along with centralized logging and metrics systems, helps in maintaining visibility and diagnosing issues across microservices.
Team Skills and Culture
Ensuring that the development teams are equipped with the necessary skills for microservices, and fostering a culture of autonomy, accountability, and collaboration are essential for a successful transition.
The Last Word
The migration from monolithic to microservices architecture is a significant undertaking that requires careful planning, strategic refactoring, and the right technological choices. Java, with its adaptability, strong ecosystem, and robust tooling, provides an ideal foundation for organizations embarking on this journey.
While the transition poses its challenges, the rewards in terms of scalability, agility, and maintainability can be substantial. By employing best practices, leveraging suitable frameworks, and nurturing a culture of continuous improvement, organizations can effectively unlock the potential of microservices while preserving the strengths of Java development.
Embracing this evolution in software architecture, organizations can position themselves to meet the dynamic demands of modern applications and pave the way for future innovations.
References:
In summary, the journey from monolith to microservices is a transformative process that demands careful planning, the choice of appropriate tools and frameworks, and the application of best practices. With Java as the cornerstone, this transition can unlock an organization's potential for scalability, flexibility, and efficiency in software development.