Boost Your Android App with Stroboscopic Torch Features!
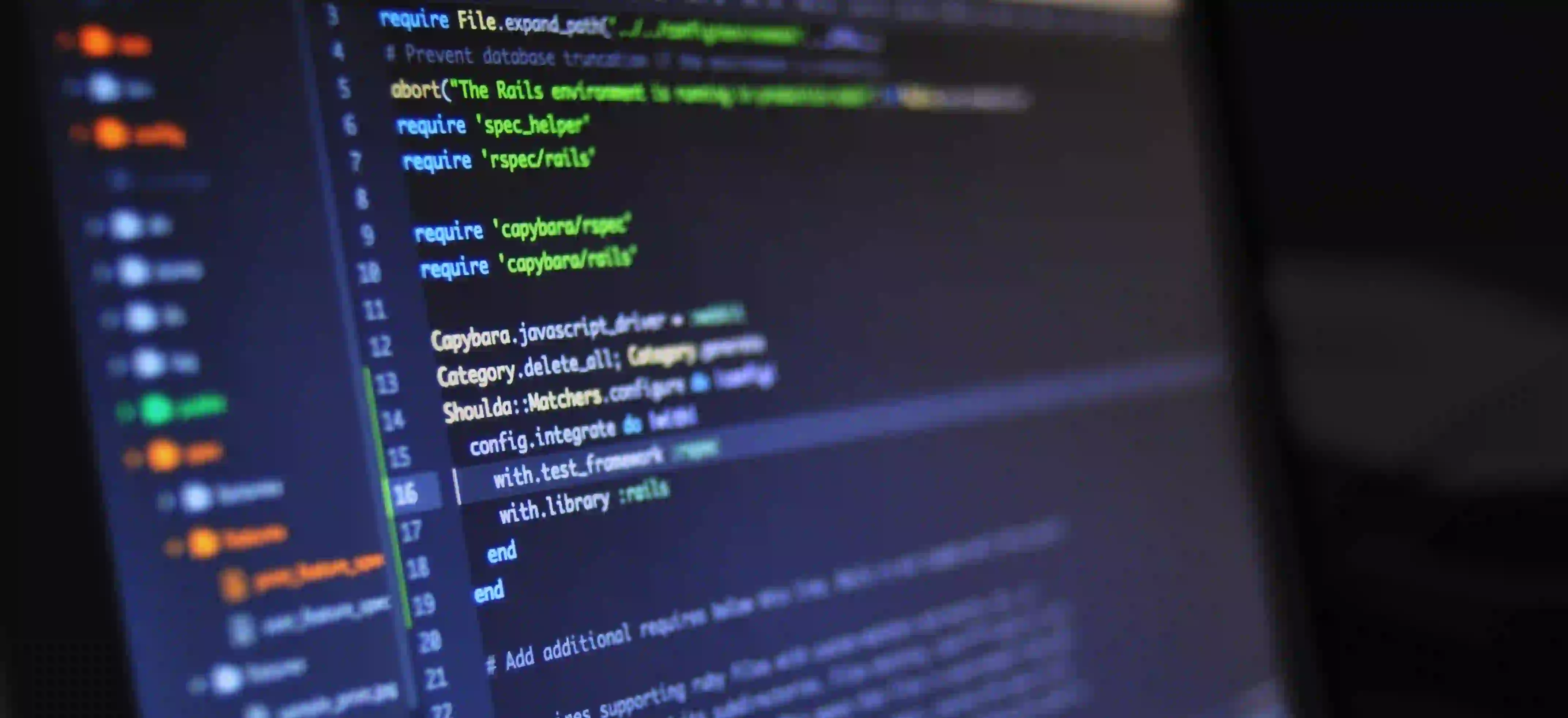
Illuminate Your Android App with Stroboscopic Torch
As a Java developer, you're always on the lookout for ways to enhance the functionality and appeal of your Android applications. One way to do this is by incorporating stroboscopic torch features into your app. Not only does this add a fun and useful element to your app, but it also showcases your expertise in handling hardware-related functionalities. In this blog post, we'll explore how to integrate stroboscopic torch features into your Android app using Java.
Understanding Stroboscopic Torch
Before diving into the implementation, let's take a moment to understand what a stroboscopic torch feature entails. Essentially, it enables the torch (or flash) of the device to flicker at a rapid pace, creating a stroboscopic effect. This functionality can be utilized in various scenarios such as creating a visual alert, adding an element of fun to apps, or incorporating it into a specific feature within your app.
Setting Up the Project
To begin, make sure you have Android Studio installed on your system. Create a new Android project or open an existing one where you want to integrate the stroboscopic torch feature.
Accessing Torch Functionality
In order to control the torch of the device, we need to access the camera feature. This can be achieved using the CameraManager class introduced in Android API level 23 (Android 6.0, Marshmallow) which provides the ability to interact with camera devices.
Let's start by gaining access to the camera manager within our app. Below is an example of how to do this by obtaining an instance of the CameraManager:
CameraManager cameraManager = (CameraManager) getSystemService(Context.CAMERA_SERVICE);
The getSystemService(Context.CAMERA_SERVICE)
call retrieves the CameraManager instance, which we can then use to manipulate the torch on the device.
Toggling the Torch
We can control the torch by accessing the camera's characteristics and setting the torch mode accordingly. The code snippet below demonstrates how to toggle the torch on and off:
String cameraId = cameraManager.getCameraIdList()[0]; // Obtain the camera ID
boolean isTorchOn = false; // Assume torch is initially off
try {
cameraManager.setTorchMode(cameraId, true); // Turn on the torch
isTorchOn = true;
} catch (CameraAccessException e) {
e.printStackTrace();
// Handle exceptions
}
// Later in the code, to turn off the torch
try {
cameraManager.setTorchMode(cameraId, false); // Turn off the torch
isTorchOn = false;
} catch (CameraAccessException e) {
e.printStackTrace();
// Handle exceptions
}
In the code above, we obtain the camera ID, then proceed to toggle the torch on and off as required. It's important to handle any potential exceptions that may occur during this process to ensure the app's stability.
Implementing Stroboscopic Effect
Now that we can control the torch of the device, we can create the stroboscopic effect by toggling the torch rapidly at predefined intervals. This can be accomplished using a Handler to schedule repeated actions. The following code showcases how to achieve this effect:
final Handler handler = new Handler();
final Runnable runnable = new Runnable() {
@Override
public void run() {
if (isTorchOn) {
// Turn off the torch if currently on
try {
cameraManager.setTorchMode(cameraId, false);
isTorchOn = false;
} catch (CameraAccessException e) {
e.printStackTrace();
// Handle exceptions
}
} else {
// Turn on the torch if currently off
try {
cameraManager.setTorchMode(cameraId, true);
isTorchOn = true;
} catch (CameraAccessException e) {
e.printStackTrace();
// Handle exceptions
}
}
// Schedule the next toggle after a specified interval
handler.postDelayed(this, 100); // Adjust the interval as desired
}
};
// Start the stroboscopic effect
handler.post(runnable);
In the above code, we define a Handler to schedule the toggling of the torch at regular intervals. The run()
method within the Runnable
implementation encapsulates the logic to switch the torch on and off rapidly, thus creating the stroboscopic effect. Adjust the interval (in milliseconds) to achieve the desired flickering rate.
Integrating User Controls
To enhance the user experience, consider incorporating controls to enable and disable the stroboscopic effect based on user input. This can be achieved through buttons or switches within the app's interface. Here's an example of how to integrate a toggle button for controlling the stroboscopic effect:
ToggleButton toggleButton = findViewById(R.id.toggleButton);
toggleButton.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) {
if (isChecked) {
// Start the stroboscopic effect
handler.post(runnable);
} else {
// Stop the stroboscopic effect
handler.removeCallbacksAndMessages(null);
// Ensure the torch is turned off when the effect is stopped
if (isTorchOn) {
try {
cameraManager.setTorchMode(cameraId, false);
isTorchOn = false;
} catch (CameraAccessException e) {
e.printStackTrace();
// Handle exceptions
}
}
}
}
});
In this example, a ToggleButton
is used to allow the user to control the activation and deactivation of the stroboscopic effect. The onCheckedChanged
listener ensures that the stroboscopic effect starts and stops accordingly, while also handling the cleanup process when the effect is disabled.
Additional Considerations
When implementing the stroboscopic torch feature, it's important to consider the potential impact on device battery life and the user's visual comfort. Ensure the flickering rate is reasonable and provide an option to disable the feature if it's not essential for the app's functionality.
Testing is crucial to validate the functionality on a variety of devices to ensure consistent behavior across different hardware and Android versions.
The Bottom Line
Incorporating a stroboscopic torch feature into your Android app can add a unique and engaging element that sets your app apart. By leveraging the camera functionality and scheduling rapid torch toggling, you can create an exciting visual effect that enhances user interaction. Remember to handle exceptions, consider user controls, and test your implementation thoroughly to deliver a polished and reliable feature.
Now that you've gained insights into integrating stroboscopic torch features using Java, it's time to illuminate your Android app with this captivating functionality!
So, go ahead, implement the stroboscopic torch feature, and watch your app shine in a new light!
Start integrating the stroboscopic torch features today and see your Android app glow bright!