Mastering Spring MVC Test Framework: Common Pitfalls
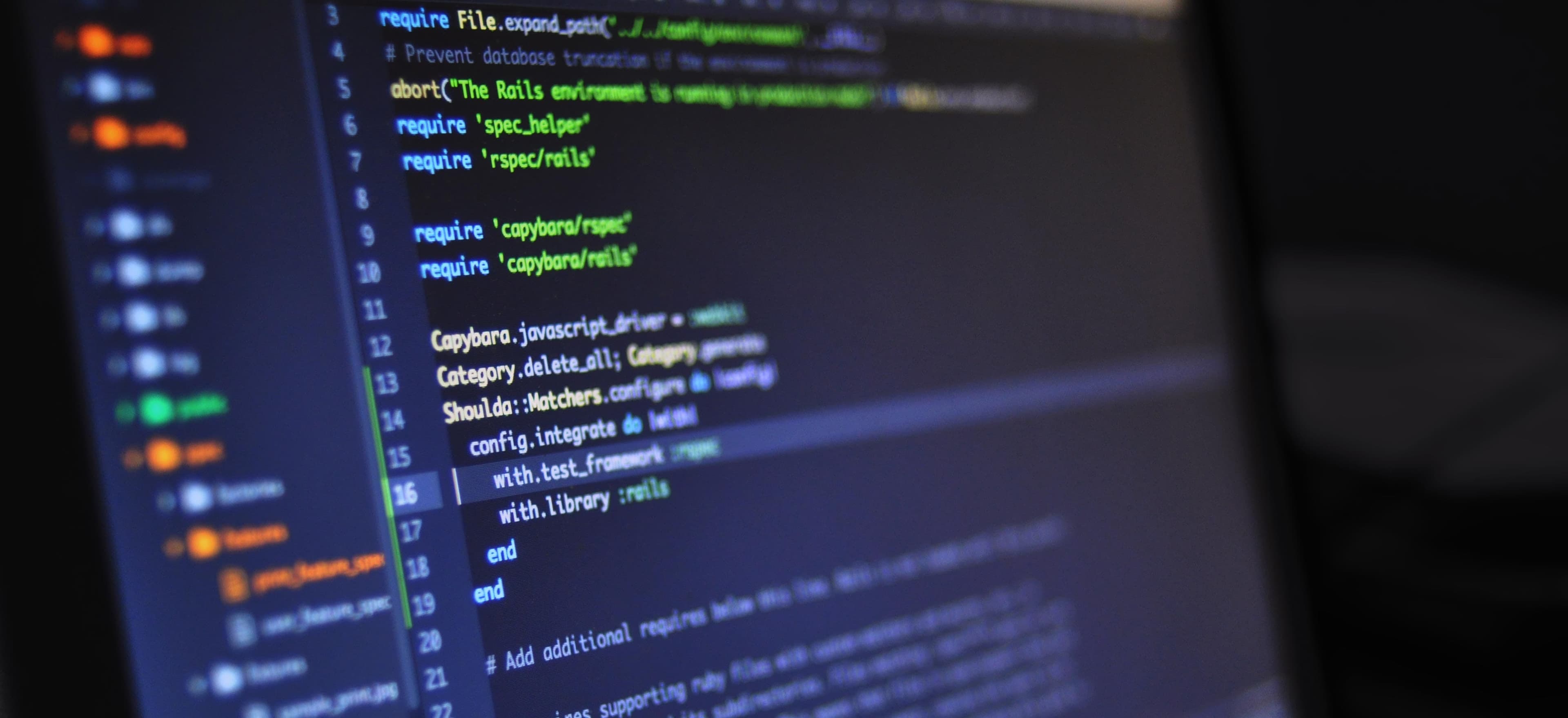
- Published on
Mastering Spring MVC Test Framework: Navigating Common Pitfalls with Ease
Spring MVC Test has revolutionized the way developers test their web applications. By simulating real HTTP requests and responses, it provides an accurate reflection of your app's behavior in a production-like environment. However, navigating this powerful framework isn't without its challenges. In this guide, we'll delve into common pitfalls associated with Spring MVC Test, offering strategies and code examples to help you master this framework and ensure your testing suite is both robust and efficient.
Understanding Spring MVC Test
Before we dive into the pitfalls, let's establish a basic understanding of what Spring MVC Test is. At its core, it is a module of the broader Spring Framework that enables testing of Spring MVC components without the need of deploying them to a real servlet container. This is achieved through the MockMvc
class, which comes bundled with methods to send HTTP requests and assert responses.
For further reading on Spring MVC Test's architecture and capabilities, the official Spring documentation is an excellent resource.
Pitfall 1: Incorrect Context Configuration
One common mistake is not correctly specifying the application context configuration. This can lead to tests that are either slow, due to loading unnecessary configurations, or fail because of missing beans.
Solution:
Ensure that you're only loading the relevant configuration for your tests. Use the @WebAppConfiguration
and @ContextConfiguration
annotations judiciously.
@WebAppConfiguration
@ContextConfiguration(classes = {WebAppConfig.class})
public class MyControllerTest {
@Autowired
private WebApplicationContext wac;
private MockMvc mockMvc;
@Before
public void setup() {
this.mockMvc = MockMvcBuilders.webAppContextSetup(wac).build();
}
// Test methods...
}
In the example above, WebAppConfig.class
should contain only the necessary configuration for the tests to run. This keeps your test context lean and mean.
Pitfall 2: Not Mocking External Services
When testing a controller that depends on external services or beans, forgetting to mock these dependencies is a frequent oversight. This can result in tests that are unreliable and difficult to maintain.
Solution:
Leverage the @MockBean
or @Mock
annotations to replace the actual beans with mocks in your test context.
public class UserControllerTest {
@Autowired
private MockMvc mockMvc;
@MockBean
private UserService userService; // Mocking the external UserService
@Before
public void setup() {
// Setup your MockMvc and any necessary stubbing for userService
}
// Your test methods go here...
}
By mocking the UserService
, we ensure that our controller tests are focused solely on the controller's behavior and not on the underlying service logic.
Pitfall 3: Inadequate Request and Response Assertions
Another pitfall is not sufficiently asserting the HTTP request and response, leading to tests that may pass despite unwanted behavior in the application.
Solution:
Use MockMvc
's fluent API to construct precise request and response assertions.
@Test
public void whenValidInput_thenReturns200() throws Exception {
mockMvc.perform(get("/users/1"))
.andExpect(status().isOk())
.andExpect(jsonPath("$.name").value("John Doe"));
}
In this snippet, the test explicitly checks not just for a 200 OK status but also validates the response body.
Pitfall 4: Overlooking Security
Ignoring security configurations in tests can lead to a false sense of confidence. Just because your tests pass, doesn't mean your application is secure.
Solution:
Integrate Spring Security Test by adding the @WithMockUser
or @WithSecurityContext
annotations to test methods that require authentication.
@Test
@WithMockUser(username="admin", roles={"USER","ADMIN"})
public void givenAdminUser_whenGetProtectedResource_thenOk() throws Exception {
mockMvc.perform(get("/admin/resource"))
.andExpect(status().isOk());
}
This ensures your tests respect your security configurations, providing a more accurate picture of your application's behavior.
Avoiding Pitfalls: Best Practices
Mastering Spring MVC Test requires more than avoiding pitfalls; it's about adopting best practices that strengthen and streamline your testing suite:
- Leverage
@WebMvcTest
for Controller Tests: This slices the context to only load components relevant for MVC testing, making your tests faster and more focused. - Use
@DataJpaTest
,@RestClientTest
, and other slice tests: When testing specific layers of your application, use these annotations to load only the necessary context. - Integrate Continuous Integration (CI): Ensure your tests run on a CI server. This catches errors early and improves code quality.
- Keep Tests Focused: Avoid testing more than one thing. If you're testing a controller, don't test the service logic again.
A Final Look
While Spring MVC Test is a powerful toolkit in a developer's arsenal, it comes with its own set of challenges. By understanding and avoiding common pitfalls – from context configuration errors to inadequate security testing – you can harness the full power of this framework.
For applications where user experience hinges on flawless web interactions, adopting these practices isn't just advisable; it's essential. As you refine your testing strategies and embrace the best practices mentioned, you'll find your prowess with Spring MVC Test growing, allowing you to craft more reliable, robust, and user-friendly web applications.
For a more in-depth exploration of Spring MVC Test and its capabilities, visit the Spring MVC Test documentation.
Remember, exceptional software is not just written; it's tested. Equip yourself with the knowledge to avoid these common pitfalls, and you're on your way to mastering Spring MVC Test.