Safeguarding Your Site: Top Web App Authentication Pitfalls
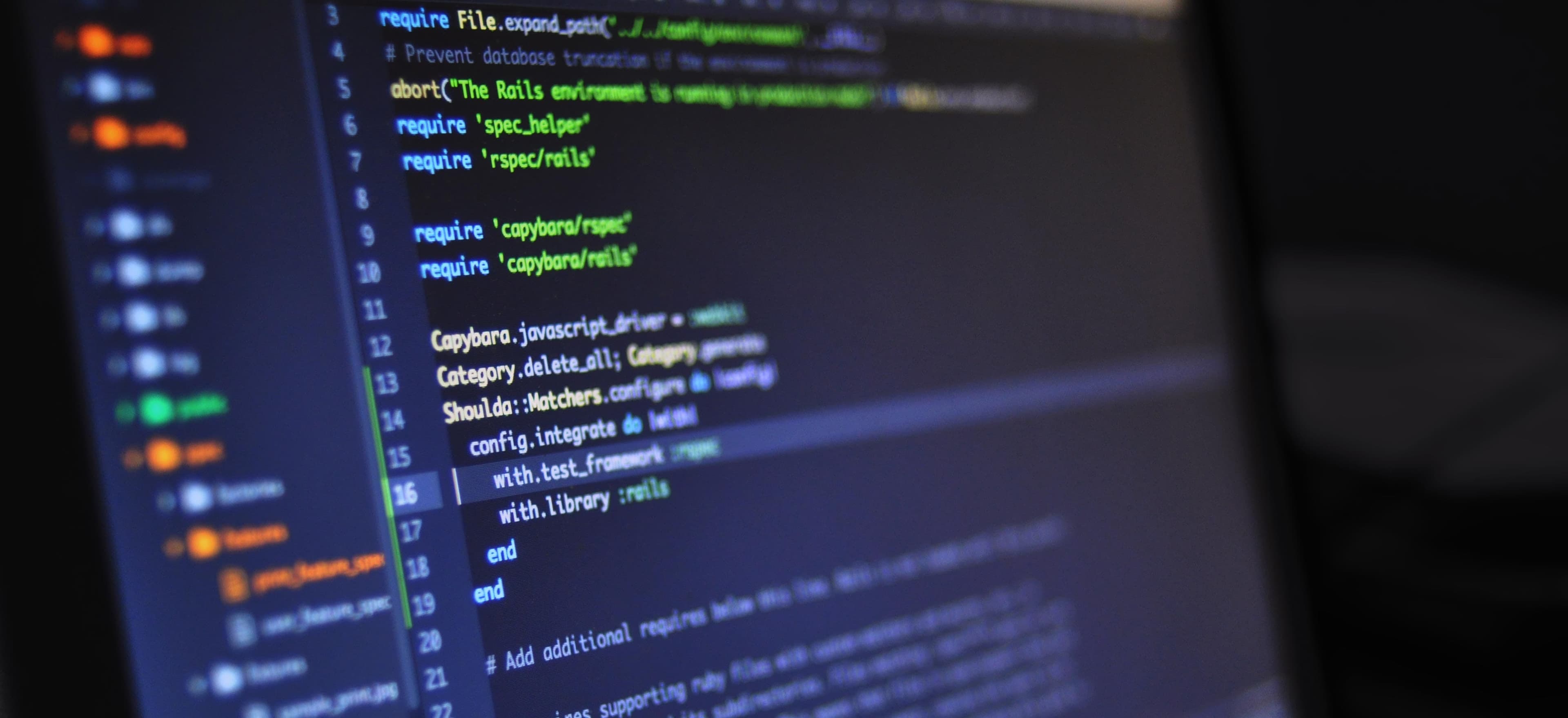
- Published on
Safeguarding Your Site: Top Web App Authentication Pitfalls
Website security is a paramount concern for any developer, especially when it comes to authentication. Java is a popular choice for web application development, and understanding the common pitfalls in web app authentication is crucial. In this post, we'll explore some of the top authentication pitfalls and discuss how Java can help mitigate these risks.
Understanding Authentication Pitfalls
Authentication is the process of verifying the identity of a user, typically through a username and password combination. However, implementing authentication is not as straightforward as it may seem. There are several common pitfalls that developers must be aware of to ensure the security of their web applications.
Common Pitfalls
-
Weak Password Policies: Allowing weak passwords, such as those that are too short or easily guessable, can compromise the security of the entire application.
-
Insecure Storage of Credentials: Storing user credentials in plain text or using weak hashing algorithms can lead to unauthorized access if the database is compromised.
-
Insufficient Session Management: Inadequate session management can result in session hijacking or fixation, allowing attackers to impersonate legitimate users.
-
Inadequate Account Lockout: Without proper account lockout mechanisms, attackers can launch brute force attacks to guess user passwords.
-
Improper Error Handling: Revealing specific details about why authentication failed can aid attackers in finding vulnerabilities.
Mitigating Pitfalls with Java
Java provides robust tools and libraries to address these authentication pitfalls effectively. Let's delve into some best practices and code snippets to demonstrate how Java can help mitigate these risks.
Enforcing Strong Password Policies
Code Snippet
public class PasswordPolicyValidator {
public boolean isValidPassword(String password) {
// Implement logic to enforce password policy, e.g., minimum length, complexity requirements
}
}
Commentary
In the code snippet above, the PasswordPolicyValidator
class can enforce password policies by implementing logic to check for minimum password length, complexity requirements (e.g., upper and lower case letters, special characters), and denying commonly used passwords. By validating passwords against these criteria, developers can ensure that only strong passwords are accepted.
Secure Credential Storage
Code Snippet
public class PasswordHasher {
public String hashPassword(String password) {
// Implement strong hashing algorithm (e.g., bcrypt) to securely hash the password
}
}
Commentary
In this snippet, the PasswordHasher
class demonstrates the use of a strong hashing algorithm, such as bcrypt, to securely hash user passwords before storing them in the database. Using strong cryptographic hashing algorithms is crucial in protecting user credentials, even if the database is compromised.
Robust Session Management
Code Snippet
public class SessionManager {
public void createSession(User user) {
// Implement secure session creation and management, including token generation and expiration
}
}
Commentary
The SessionManager
class showcases how Java can be used to implement secure session management, including the generation of unique session tokens and proper expiration handling. By employing secure session management practices, developers can prevent session hijacking and fixation attacks.
Implementing Account Lockout
Code Snippet
public class LoginAttemptsManager {
public void handleFailedLoginAttempts(User user) {
// Implement logic to track failed login attempts and lock the account if the threshold is exceeded
}
}
Commentary
In the above code, the LoginAttemptsManager
class illustrates how to track and handle failed login attempts, eventually locking the user account if a certain threshold is exceeded. Implementing account lockout mechanisms can thwart brute force attacks, thereby enhancing the security of the authentication process.
Secure Error Handling
Code Snippet
try {
// Perform authentication
} catch (AuthenticationException e) {
// Handle authentication errors without revealing sensitive information
}
Commentary
In this snippet, the try-catch
block demonstrates how to handle authentication errors without divulging specific details that could aid attackers. Proper error handling is essential in preventing attackers from exploiting vulnerabilities through error messages.
Key Takeaways
Web app authentication is a critical aspect of security, and understanding and mitigating its pitfalls is paramount. Java offers a wealth of tools, libraries, and best practices to address common authentication pitfalls effectively. By enforcing strong password policies, securely storing credentials, implementing robust session management, enforcing account lockout, and ensuring secure error handling, developers can bolster the security of their web applications.
By utilizing Java's features and best practices, developers can build and maintain secure web applications, thus safeguarding their sites and the sensitive data of their users.
Remember, secure authentication is not a one-time task; it requires ongoing monitoring and updates to address evolving security threats. Stay vigilant and keep your web applications secure!