Master SOAP Message Logging in Spring: A JAX-WS Guide
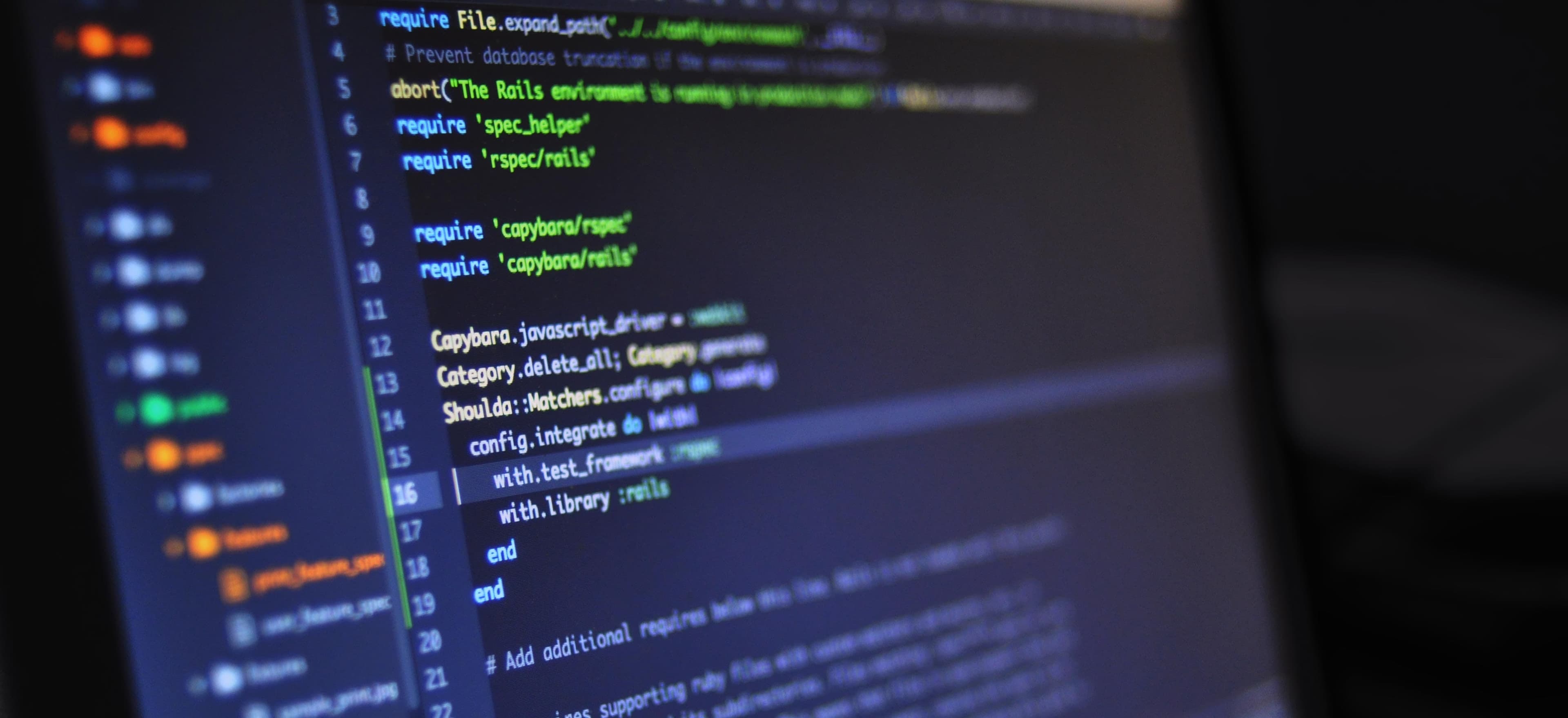
- Published on
Master SOAP Message Logging in Spring: A JAX-WS Guide
When working with web services, especially SOAP-based ones, logging the incoming and outgoing messages is crucial for debugging, auditing, and compliance purposes. In this guide, we'll delve into how to efficiently set up SOAP message logging in a Spring-based JAX-WS application.
Understanding SOAP Message Logging
SOAP (Simple Object Access Protocol) messages are XML-based and are used to facilitate communication between web services. Logging these messages allows developers to inspect the actual requests and responses being exchanged between client and server, which is indispensable for troubleshooting and understanding the system's behavior.
Setting Up the Project
Before we begin, let's ensure we have a Spring-based JAX-WS project set up. If you're new to this, you can refer to Spring's official documentation for guidance. Once your project is ready, we can proceed with configuring SOAP message logging.
Configuring Message Logging in Spring
In Spring, logging SOAP messages for a JAX-WS service is primarily achieved through the use of interceptors. Interceptors allow us to capture the incoming and outgoing messages and perform custom processing on them.
Let's create a class CustomLoggingInterceptor
that extends EndpointInterceptor
to capture and log the SOAP messages.
import org.springframework.ws.context.MessageContext;
import org.springframework.ws.server.EndpointInterceptor;
public class CustomLoggingInterceptor implements EndpointInterceptor {
@Override
public boolean handleRequest(MessageContext messageContext, Object endpoint) throws Exception {
// Log incoming request message
return true;
}
@Override
public boolean handleResponse(MessageContext messageContext, Object endpoint) throws Exception {
// Log outgoing response message
return true;
}
// Implement other methods if necessary
}
In the handleRequest
and handleResponse
methods, we can access the messageContext
to retrieve and log the inbound and outbound SOAP messages, respectively.
Registering the Interceptor
Once the CustomLoggingInterceptor
is in place, it needs to be registered with the JAX-WS configuration. In a Spring-based JAX-WS application, this can be achieved using the PayloadRootAnnotationMethodEndpointMapping
bean.
import org.springframework.ws.server.EndpointInterceptor;
import org.springframework.ws.server.endpoint.mapping.PayloadRootAnnotationMethodEndpointMapping;
@Configuration
public class WebServiceConfig {
@Bean
public PayloadRootAnnotationMethodEndpointMapping endpointMapping() {
PayloadRootAnnotationMethodEndpointMapping endpointMapping = new PayloadRootAnnotationMethodEndpointMapping();
endpointMapping.setInterceptors(new EndpointInterceptor[]{new CustomLoggingInterceptor()});
return endpointMapping;
}
// Other bean configurations for web services
}
By injecting the CustomLoggingInterceptor
into the PayloadRootAnnotationMethodEndpointMapping
, we ensure that the interceptor is invoked for each incoming request and outgoing response.
Logging the Messages
With the interceptor in place and registered, we can now implement the logging logic within the handleRequest
and handleResponse
methods.
@Override
public boolean handleRequest(MessageContext messageContext, Object endpoint) throws Exception {
String requestMessage = messageContext.getRequest().toString();
log.info("Incoming SOAP request: " + requestMessage);
return true;
}
@Override
public boolean handleResponse(MessageContext messageContext, Object endpoint) throws Exception {
String responseMessage = messageContext.getResponse().toString();
log.info("Outgoing SOAP response: " + responseMessage);
return true;
}
In this example, we simply retrieve the request and response messages as strings and log them using a logging framework like Log4j or SLF4J. Depending on your specific requirements, you can parse and format the XML messages for better readability.
Testing the Setup
To ensure that the SOAP message logging is functioning as expected, deploy your JAX-WS application and initiate some web service requests. On the server side, you should see the incoming request and outgoing response logged as per the configured interceptor.
The Closing Argument
In this guide, we've explored the importance of SOAP message logging and demonstrated how to set up logging for a Spring-based JAX-WS application using interceptors. Effective message logging not only aids in debugging and issue resolution but also provides valuable insights into the actual data being transmitted between web services.
By following these steps, you can master SOAP message logging in your Spring-based JAX-WS application, empowering you to efficiently monitor and troubleshoot your web service interactions. Start implementing SOAP message logging today and elevate the robustness of your web service infrastructure.
Happy coding!
Checkout our other articles