3 Steps to Integrate Sensors in Your Arduino IoT Project
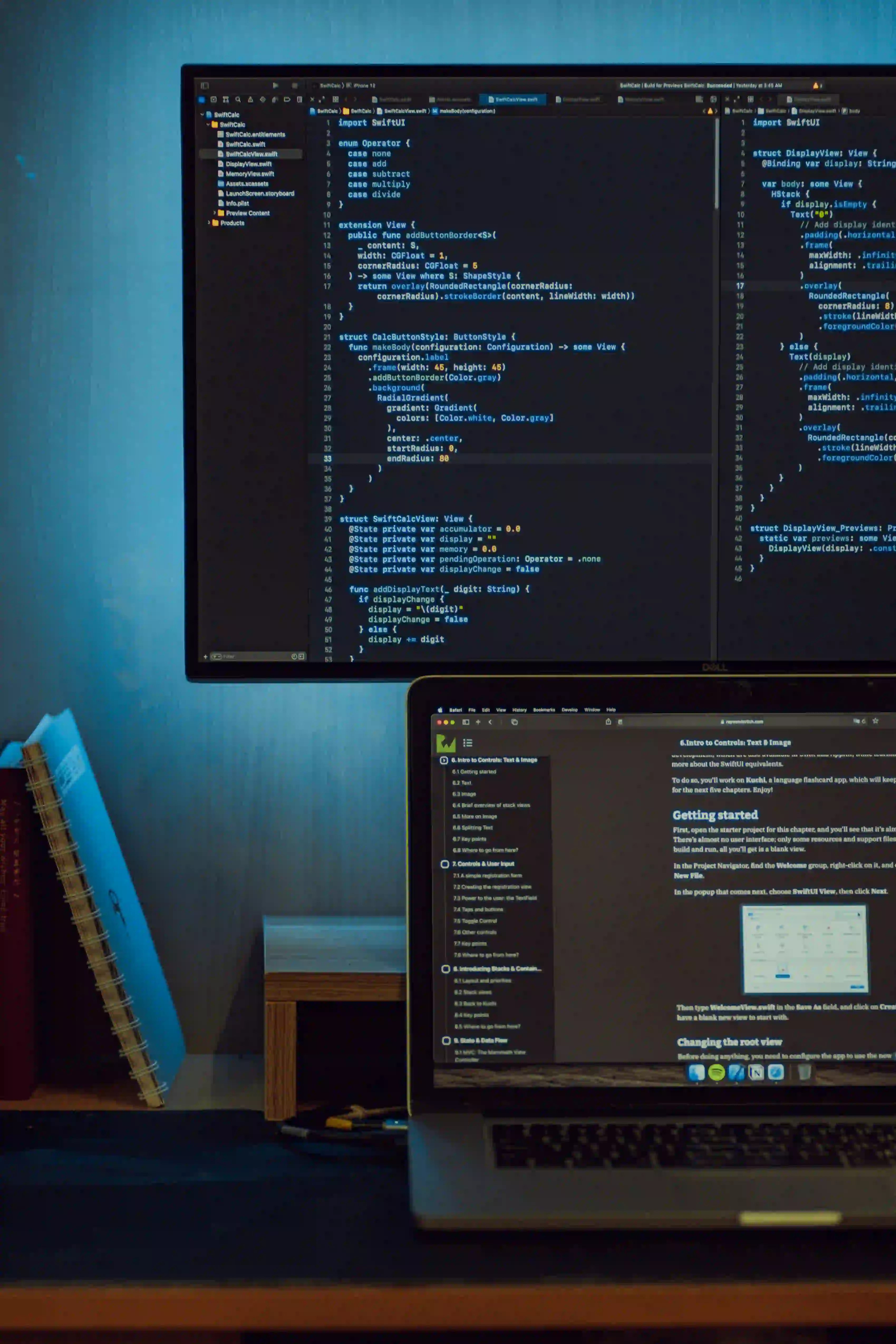
3 Steps to Integrate Sensors in Your Arduino IoT Project
The Internet of Things (IoT) has opened a gateway for hobbyists and tech enthusiasts to explore and innovate with interconnected devices. Arduino, with its simplicity and vast community support, stands as a pivotal platform for bringing IoT projects to life. In this comprehensive guide, we'll dive into the process of integrating sensors into your Arduino IoT projects. Whether you're monitoring temperature, detecting motion, or measuring light intensity, incorporating sensors not only breathes life into your projects but also introduces you to real-world applications of IoT.
Step 1: Choosing the Right Sensor
Before diving into the coding and connections, the first step is understanding and selecting the appropriate sensor for your project. Sensors come in various shapes and sizes, each designed for specific tasks. For instance, the DHT11 or DHT22 sensors are perfect for measuring temperature and humidity, while the PIR sensor is ideal for motion detection.
When choosing a sensor, consider the following aspects:
- Project Requirements: Identify what physical quantities you need to measure.
- Accuracy and Range: Ensure the sensor meets the accuracy and range suitable for your application.
- Interface: Check whether the sensor is analog or digital, and verify its compatibility with your Arduino board.
For further insights into selecting the right sensor, you might find this comprehensive guide helpful: Choosing the Right Sensor for Your Arduino Project.
Step 2: Wiring and Connecting the Sensor to Arduino
Once you've selected the suitable sensor, the next step involves physically connecting it to your Arduino board. As an example, we'll focus on integrating a DHT11 temperature and humidity sensor into an Arduino Uno.
Required Components:
- Arduino Uno
- DHT11 Sensor
- Breadboard
- Jumper Wires
Wiring Diagram:
- Connect the VCC pin of the DHT11 sensor to the 5V pin on the Arduino.
- Connect the GND pin to one of the GND pins on the Arduino.
- Connect the Data pin of the DHT11 to a digital pin on the Arduino (for example, pin 2).
Why These Connections?
- The VCC and GND provide power to the sensor, enabling it to operate.
- The Data pin is responsible for sending the temperature and humidity data to the Arduino.
Step 3: Programming the Arduino
With the sensor properly connected, you're now ready to dive into the code. The objective is to write a program that reads data from the sensor and prints it to the serial monitor.
Required Libraries:
First, you'll need to include the DHT sensor library in your Arduino IDE. You can install it through the Library Manager or download it from Adafruit's GitHub repository.
Sample Code:
#include <DHT.h>
#define DHTPIN 2 // Digital pin connected to the DHT sensor
#define DHTTYPE DHT11 // Define the type of DHT sensor
DHT dht(DHTPIN, DHTTYPE);
void setup() {
Serial.begin(9600);
dht.begin();
}
void loop() {
// Wait a few seconds between measurements.
delay(2000);
// Reading temperature and humidity
float humidity = dht.readHumidity();
float temperature = dht.readTemperature();
// Check if the reading failed and exit early (to try again).
if (isnan(humidity) || isnan(temperature)) {
Serial.println("Failed to read from the DHT sensor!");
return;
}
// Print the results to the Serial Monitor
Serial.print("Humidity: ");
Serial.print(humidity);
Serial.print("% Temperature: ");
Serial.print(temperature);
Serial.println("°C");
}
Code Commentary:
- The
DHT.h
library abstracts the complex part of reading data from the sensor, allowing you to focus on what's important — the data. - The
#define
preprocessor commands specify the pin number and sensor type, simplifying code reuse and modifications. - Initializing the DHT object and calling
dht.begin()
in thesetup()
function prepares the sensor for data transmission. - The
loop()
function contains the logic to read humidity and temperature, check for unsuccessful readings, and print the data to the Serial Monitor.
Tips for Successful Sensor Integration
- Always Test Your Sensor: Before incorporating a sensor into your project, connect it independently to ensure it's working as expected.
- Power Considerations: Some sensors may require more power than what the Arduino can provide through its 5V or 3.3V pins. In such cases, consider using an external power source.
- Debugging: If the readings from the sensor seem off, double-check your connections, review the sensor's datasheet, and ensure your code reflects the proper configuration.
Integrating sensors into your Arduino projects can be an enriching experience that not only enhances your programming skills but also provides a deeper understanding of the physical world around us. Whether you’re building a weather station, a smart home device, or any other IoT project, following these steps ensures a seamless integration process, paving the way for endless possibilities in the realm of electronics and IoT.
Embrace the process, experiment with different sensors, and most importantly, enjoy the journey of bringing your innovative ideas to life.