Why Fluent Interfaces Fail at Long-term Code Maintenance
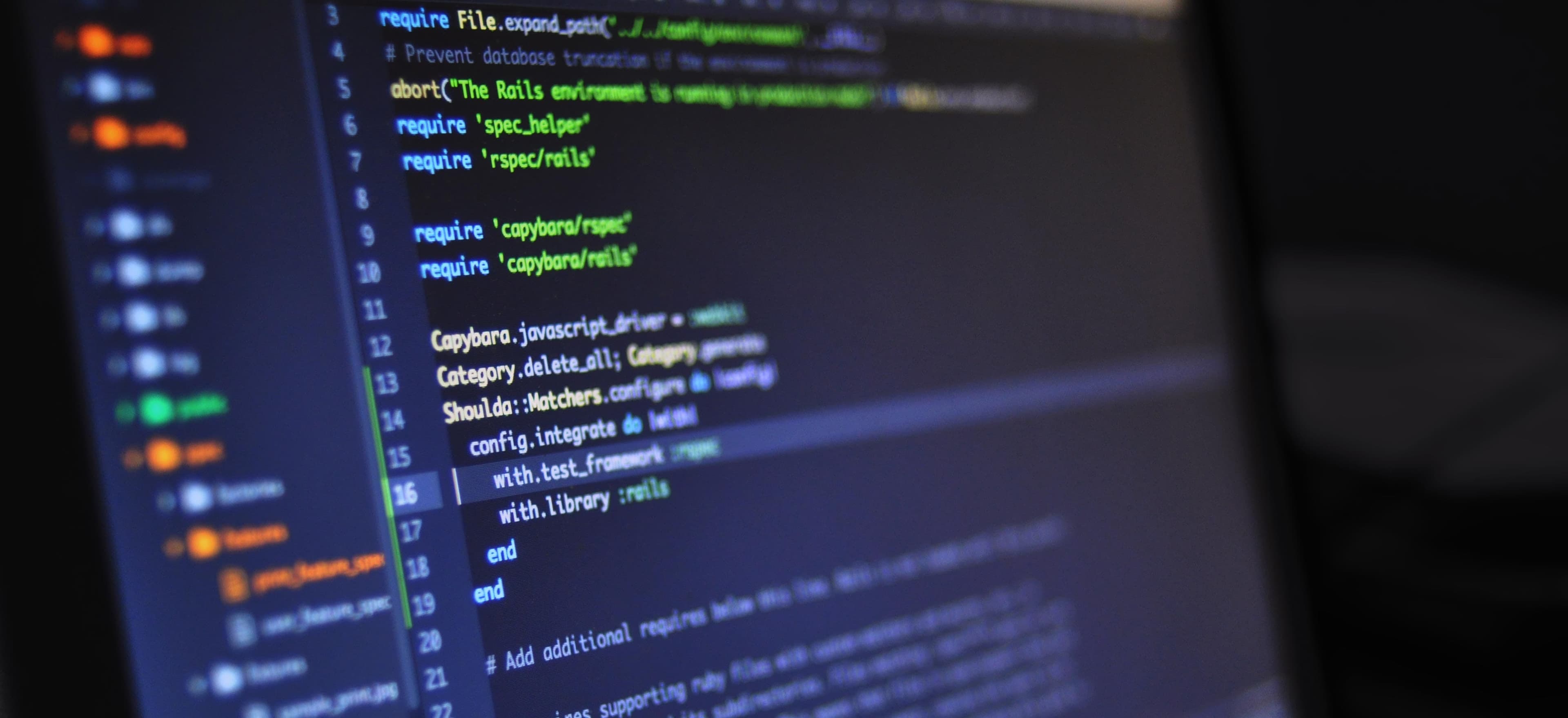
- Published on
The Pitfalls of Fluent Interfaces in Java
When developing software applications, developers often seek to improve the readability and maintainability of their code. One common approach to achieving this goal is through the use of fluent interfaces. Fluent interfaces allow developers to write code that reads like natural language, making it easy to understand the intent of the code. However, while fluent interfaces can initially appear elegant and intuitive, they often fail to live up to their promises of long-term code maintainability. In this article, we'll explore the pitfalls of fluent interfaces in Java, and why they may not be the best choice for long-term code maintenance.
What are Fluent Interfaces?
Fluent interfaces, also known as method chaining, are a design pattern that allows the developer to chain method calls together in a way that creates a more readable, fluent syntax. This can be achieved by designing methods to return the object itself, enabling method calls to be chained together in a single statement.
public class FluentExample {
private String name;
private int age;
public FluentExample withName(String name) {
this.name = name;
return this;
}
public FluentExample withAge(int age) {
this.age = age;
return this;
}
}
FluentExample example = new FluentExample()
.withName("John")
.withAge(30);
The code snippet above illustrates a simple fluent interface that allows method calls to be chained together in a readable and fluid manner.
The Allure of Fluent Interfaces
Fluent interfaces have gained popularity due to their ability to create expressive and easily readable code. When well-designed, fluent interfaces can lead to more maintainable code by clearly expressing the intent of the operations being performed. Additionally, fluent interfaces often result in more concise and expressive code, reducing the need for temporary variables and making the code easier to reason about.
The Pitfalls of Fluent Interfaces for Long-term Maintenance
1. Hidden Complexity
While fluent interfaces can make the code appear more readable and elegant, they often hide underlying complexity. Chained method calls can lead to unexpected side effects and make it challenging to analyze the flow of the code. This hidden complexity can make it difficult for new developers joining the project to understand the logic and follow the code flow, ultimately hindering long-term maintenance efforts.
Example:
FluentExample example = new FluentExample()
.withName("John")
.withAge(30);
// It's not immediately obvious if the following operation modifies the 'example' object
String modifiedName = example.withName("Jane").getName();
In the example above, the fluent interface allows the withName
method to be called again, potentially modifying the state of the example
object in an unexpected manner. This hidden behavior can lead to confusion and introduce bugs during maintenance or refactoring.
2. Breaks Method Chaining
In certain scenarios, fluent interfaces can break the method chaining pattern, making the code less readable and harder to follow. When methods return a different type than the original object, it disrupts the fluent interface and forces the developer to handle the unexpected type, making the code less elegant and harder to maintain.
Example:
public class FluentExample {
// ...
public AnotherObject getAnotherObject() {
return new AnotherObject(this.name, this.age);
}
}
// Method chaining is broken as the return type is not FluentExample
FluentExample example = new FluentExample()
.withName("John")
.withAge(30)
.getAnotherObject();
Here, the getAnotherObject
method returns a different type, breaking the fluent interface and potentially causing confusion for developers trying to chain method calls.
3. Testing Complications
Fluent interfaces can pose challenges when writing tests, particularly when dealing with mocking frameworks. When writing unit tests, the fluent interface's method chaining can lead to complex setups for stubbing or mocking behavior, often resulting in tests that are difficult to understand and maintain.
Example:
FluentExample example = new FluentExample()
.withName("John")
.withAge(30);
// Testing becomes challenging due to the need to mock multiple method calls
Testing the behavior of the FluentExample
class becomes complicated when trying to stub or mock method chaining, potentially leading to fragile tests and increased maintenance overhead.
The Alternatives to Fluent Interfaces
While fluent interfaces offer an appealing syntax, there are alternative approaches that provide clearer and more maintainable code in the long term.
1. Builder Pattern
The Builder pattern allows for the construction of complex objects step by step. By separating the construction of an object from its representation, the Builder pattern offers a more explicit and readable way to create objects. This pattern is especially useful when dealing with a large number of optional parameters in a class.
Example:
public class BuilderExample {
private String name;
private int age;
public BuilderExample() {
// Some default initialization
}
public BuilderExample withName(String name) {
this.name = name;
return this;
}
public BuilderExample withAge(int age) {
this.age = age;
return this;
}
public BuilderExample build() {
return new BuilderExample(this);
}
}
BuilderExample example = new BuilderExample()
.withName("John")
.withAge(30)
.build();
The Builder pattern allows for a clear separation of concerns and ensures that object construction is explicit and easy to follow.
2. Immutability
Immutability can also provide an alternative to fluent interfaces by promoting code that is easier to reason about and less error-prone. By designing classes to be immutable, the state of an object cannot be altered after its creation, leading to more predictable and maintainable code.
Lessons Learned
While fluent interfaces may seem appealing for their readability and expressive syntax, they introduce hidden complexities, break method chaining, and complicate testing efforts. In the long term, these pitfalls can hinder code maintainability and lead to challenges for developers joining the project. By considering alternative patterns such as the Builder pattern or promoting immutability, developers can create code that is more straightforward and maintainable in the long run.
In conclusion, while fluent interfaces may offer short-term benefits, it's essential to carefully weigh their long-term implications and consider alternative approaches that prioritize code maintainability and readability in the face of evolving software requirements.
By understanding the drawbacks of fluent interfaces and exploring alternative patterns, developers can make informed decisions about the design of their code, ultimately contributing to more maintainable and robust software systems.
For a deeper understanding of Java design patterns and best practices, refer to Oracle's Java Tutorials and Effective Java by Joshua Bloch.
Checkout our other articles