Master Bean Validation with PrimeFaces: A Guide
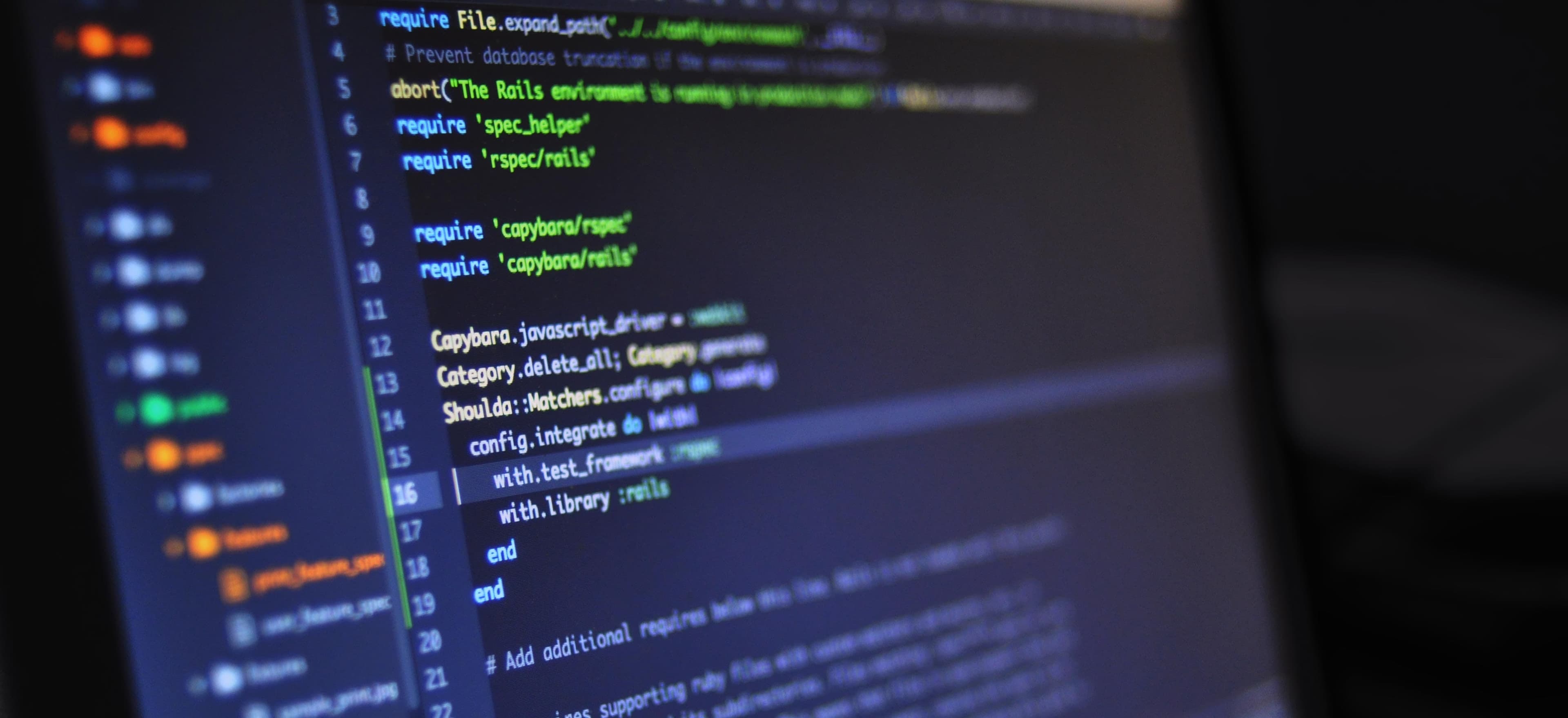
- Published on
Master Bean Validation with PrimeFaces: A Guide
Bean Validation is a powerful tool in Java for checking the validity of data and enforcing constraints on JavaBeans. When combined with PrimeFaces, a popular open-source user interface (UI) framework for JSF (JavaServer Faces), developers can create seamless and efficient web applications with robust data validation.
In this guide, we will explore the integration of Bean Validation with PrimeFaces to create a comprehensive and user-friendly data validation mechanism in Java web applications.
Why Bean Validation with PrimeFaces
Bean Validation, specified by the JSR 380, provides a framework for declarative validation constraints. PrimeFaces, on the other hand, offers a rich set of UI components for building web interfaces. By combining these two technologies, developers can ensure that the data entered by users through the PrimeFaces UI components adheres to specific validation rules as defined by the Bean Validation annotations.
Setting up the Project
Let's start by setting up a Maven project using your favorite IDE. Ensure that you have the following dependencies in your pom.xml
file:
<dependency>
<groupId>javax</groupId>
<artifactId>javaee-api</artifactId>
<version>8.0</version>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>org.primefaces</groupId>
<artifactId>primefaces</artifactId>
<version>{latest_version}</version>
</dependency>
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-validator</artifactId>
<version>{latest_version}</version>
</dependency>
Make sure to replace {latest_version}
with the current versions of PrimeFaces and Hibernate Validator.
Creating a Managed Bean
Now, let's create a managed bean that will contain the data to be validated. Below is a simple example of a managed bean with Bean Validation annotations:
import javax.inject.Named;
import javax.validation.constraints.NotNull;
import javax.validation.constraints.Size;
@Named
public class UserBean {
@NotNull
@Size(min = 3, max = 50)
private String username;
@NotNull
@Size(min = 6, max = 20)
private String password;
// Getters and setters
}
In this example, we have used @NotNull
to ensure that the fields are not null, and @Size
to define the size constraints for the username
and password
fields.
Integrating Bean Validation with PrimeFaces
Next, we'll create a form using PrimeFaces UI components and integrate Bean Validation for input fields. Here's an example of a PrimeFaces form with Bean Validation:
<h:form id="userForm">
<p:outputLabel for="username" value="Username:" />
<p:inputText id="username" value="#{userBean.username}" required="true" />
<p:outputLabel for="password" value="Password:" />
<p:password id="password" value="#{userBean.password}" required="true" />
<p:commandButton value="Submit" action="#{userBean.submit}" />
</h:form>
In the above code, we are using PrimeFaces p:outputLabel
and p:inputText
components for the username, and p:password
component for the password. The required="true"
attribute enforces client-side validation. When combined with Bean Validation annotations in the managed bean, this provides comprehensive validation for the user input.
Handling Validation Errors
To handle validation errors and display error messages to the user, we can utilize PrimeFaces' p:message
component. Here's an example of how to display validation errors next to the input fields:
<h:form id="userForm">
<p:outputLabel for="username" value="Username:" />
<p:inputText id="username" value="#{userBean.username}" required="true" />
<p:message for="username" />
<p:outputLabel for="password" value="Password:" />
<p:password id="password" value="#{userBean.password}" required="true" />
<p:message for="password" />
<p:commandButton value="Submit" action="#{userBean.submit}" />
</h:form>
By adding the p:message
component for each input field, we can display error messages generated by Bean Validation next to the relevant fields in the form.
Creating Custom Validation Messages
Bean Validation allows you to define custom error messages for each validation constraint. This can be done by specifying the message
attribute within the annotation. Let's customize the validation messages for the UserBean
class:
import javax.validation.constraints.NotNull;
import javax.validation.constraints.Size;
@Named
public class UserBean {
@NotNull(message = "Username is required")
@Size(min = 3, max = 50, message = "Username must be between 3 and 50 characters")
private String username;
@NotNull(message = "Password is required")
@Size(min = 6, max = 20, message = "Password must be between 6 and 20 characters")
private String password;
// Getters and setters
}
By customizing the error messages, we can provide specific and user-friendly feedback to the users when validation constraints are not met.
Internationalization and Localization
Bean Validation supports internationalized error messages by utilizing resource bundles. This allows developers to display validation messages in different languages based on the user's locale. To enable internationalization for Bean Validation messages, create a ValidationMessages.properties
file and provide key-value pairs for the error messages in the desired language.
The Closing Argument
In conclusion, integrating Bean Validation with PrimeFaces provides a robust solution for data validation in Java web applications. By leveraging the declarative constraints of Bean Validation along with the rich UI components of PrimeFaces, developers can create a seamless and user-friendly experience for input validation.
By following this guide, you've learned the essential steps to master Bean Validation with PrimeFaces. You've set up a Maven project, created a managed bean with Bean Validation annotations, integrated it with PrimeFaces UI components, handled validation errors, and customized validation messages. Additionally, you've gained insight into internationalizing validation messages for a global audience.
With this knowledge, you are well-equipped to build secure and reliable web applications with comprehensive data validation using Bean Validation and PrimeFaces.
Now it's time to put this into practice and start mastering Bean Validation with PrimeFaces in your own projects.
Happy coding!
To further explore the capabilities of PrimeFaces and Bean Validation, you can refer to the official documentation for PrimeFaces and Bean Validation.