Decoding Fowler: Tackling Microservices Complexity
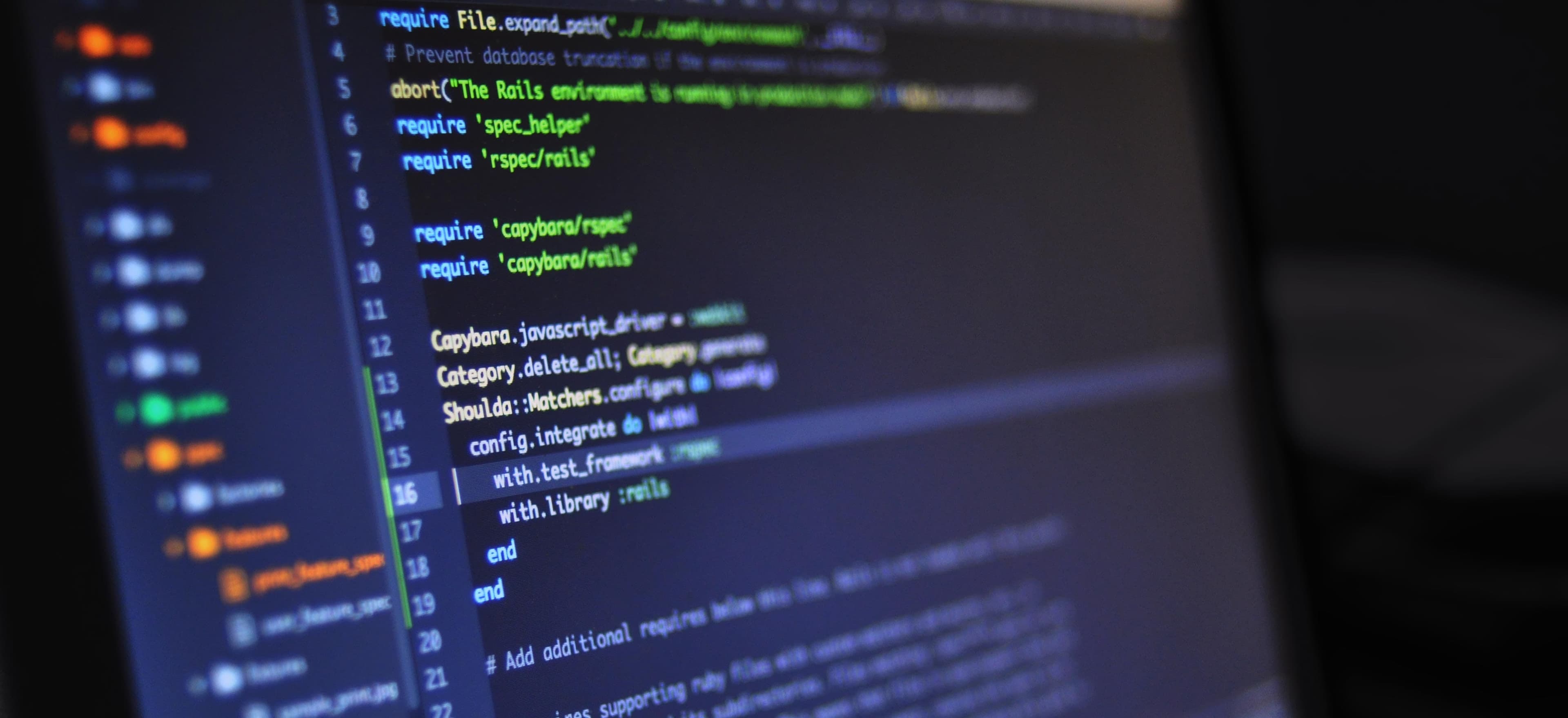
- Published on
Decoding Fowler: Tackling Microservices Complexity
In the world of software development, the concept of microservices has gained significant traction in recent years. Coined by Martin Fowler, microservices architecture has become a popular choice for building applications due to its scalability, resilience, and flexibility. However, with these benefits come complexities that developers must address in order to successfully implement and maintain microservices-based systems. In this blog post, we will delve into the challenges associated with microservices complexity and explore strategies for addressing them using Java.
Understanding Microservices Complexity
Service Interactions
One of the key complexities in a microservices architecture is managing the interactions between services. Unlike monolithic applications, where components communicate within the same process and memory space, microservices operate as independent units. As a result, developers must carefully handle communication protocols, data serialization, and network latency to ensure seamless interactions between services.
Distributed Data Management
In a microservices environment, each service manages its own data store. This distributed data management introduces challenges related to consistency, transactionality, and data integrity. Developers must carefully design data access patterns and implement mechanisms for cross-service data consistency to avoid data corruption and ensure atomic operations across multiple services.
Service Discovery and Load Balancing
Dynamic service discovery and load balancing are essential for maintaining the resilience and scalability of microservices. With services being frequently deployed and scaled independently, developers need to implement robust service discovery mechanisms and load balancing strategies to efficiently route and distribute traffic across instances of services.
Tackling Microservices Complexity with Java
Reactive Programming
Reactive programming has emerged as a powerful paradigm for addressing the complexities of asynchronous and event-driven communication in microservices. Libraries such as Project Reactor and RxJava provide developers with the tools to write composable, non-blocking, and event-driven code that seamlessly handles asynchronous service interactions.
Flux<User> users = userRepository.findAll().filter(user -> user.getAge() > 18);
users.subscribe(user -> System.out.println("Adult user: " + user.getName()));
In this example, we use Project Reactor's Flux
to asynchronously query a user repository and filter the results based on age. The reactive stream allows us to handle the asynchronous nature of the operation while maintaining composability and non-blocking behavior.
Circuit Breaker Pattern
The circuit breaker pattern is crucial for building resilient microservices that can gracefully handle failures and prevent cascading system-wide outages. Libraries like Resilience4j and Hystrix offer Java developers the ability to implement circuit breaker patterns, enabling services to gracefully degrade in the face of failures and rapidly recover when the underlying issues are resolved.
CircuitBreaker circuitBreaker = CircuitBreaker.of("backendService", CircuitBreakerConfig.custom()
.failureRateThreshold(50)
.waitDurationInOpenState(Duration.ofMillis(1000))
.permittedNumberOfCallsInHalfOpenState(3)
.slidingWindow(10, 100, SlidingWindowType.COUNT_BASED)
.build());
CompletableFuture<String> result = CircuitBreaker.decorateFuture(circuitBreaker, () -> backendService.doSomethingAsynchronously());
In this code snippet, we configure a circuit breaker with Resilience4j, defining thresholds for failure rates, wait durations, and permitted calls in the half-open state. We then decorate a CompletableFuture
with the circuit breaker, ensuring that the asynchronous operation is protected by the circuit breaker's resilience mechanisms.
Domain-Driven Design (DDD)
Domain-driven design provides a comprehensive approach to modeling complex business domains within microservices architectures. By leveraging DDD principles such as bounded contexts, aggregate roots, and domain events, Java developers can effectively delineate domain boundaries, enforce consistency boundaries, and orchestrate domain interactions within microservices.
@DomainEvent
public class OrderPlacedEvent {
private final String orderId;
private final LocalDateTime timestamp;
public OrderPlacedEvent(String orderId, LocalDateTime timestamp) {
this.orderId = orderId;
this.timestamp = timestamp;
}
// Getters and additional logic
}
In this example, we define a domain event OrderPlacedEvent
using DDD principles, encapsulating the essential information about an order being placed within the domain. This event can then be propagated across relevant microservices to trigger domain-specific actions and maintain consistency within the overall system.
Asynchronous Communication
Asynchronous communication patterns, such as messaging queues and event-driven architectures, play a vital role in decoupling services and mitigating the complexities of synchronous interactions in microservices. Frameworks like Spring Cloud Stream and Apache Kafka provide Java developers with the necessary abstractions and tools to implement asynchronous communication channels and event-driven workflows seamlessly.
@StreamListener(OrderPlacedEvent.INPUT)
public void handleOrderPlacedEvent(OrderPlacedEvent event) {
// Perform actions based on the order placed event
orderService.processOrder(event.getOrderId());
}
In this snippet, we utilize Spring Cloud Stream to define a listener for the OrderPlacedEvent
, enabling the service to asynchronously handle incoming order placement events and initiate the corresponding order processing workflow.
A Final Look
Microservices complexity presents a formidable challenge for developers aiming to build scalable, resilient, and maintainable systems. However, by leveraging the right tools and applying effective strategies, Java developers can navigate and mitigate the complexities inherent in microservices architectures. Reactive programming, circuit breaker patterns, domain-driven design, and asynchronous communication are just a few of the many powerful techniques available to tame the complexities of microservices and steer them towards success.
As you delve deeper into the realm of microservices complexity, consider exploring the comprehensive resources provided by Martin Fowler and The Reactive Manifesto to gain a deeper understanding of the principles and patterns that underpin modern microservices architecture.
Are you ready to embrace the challenges and triumphs of microservices complexity as a Java developer? Let us know your thoughts and experiences in the comments section below.