Overcoming Type Erasure in Java Generics Polymorphism
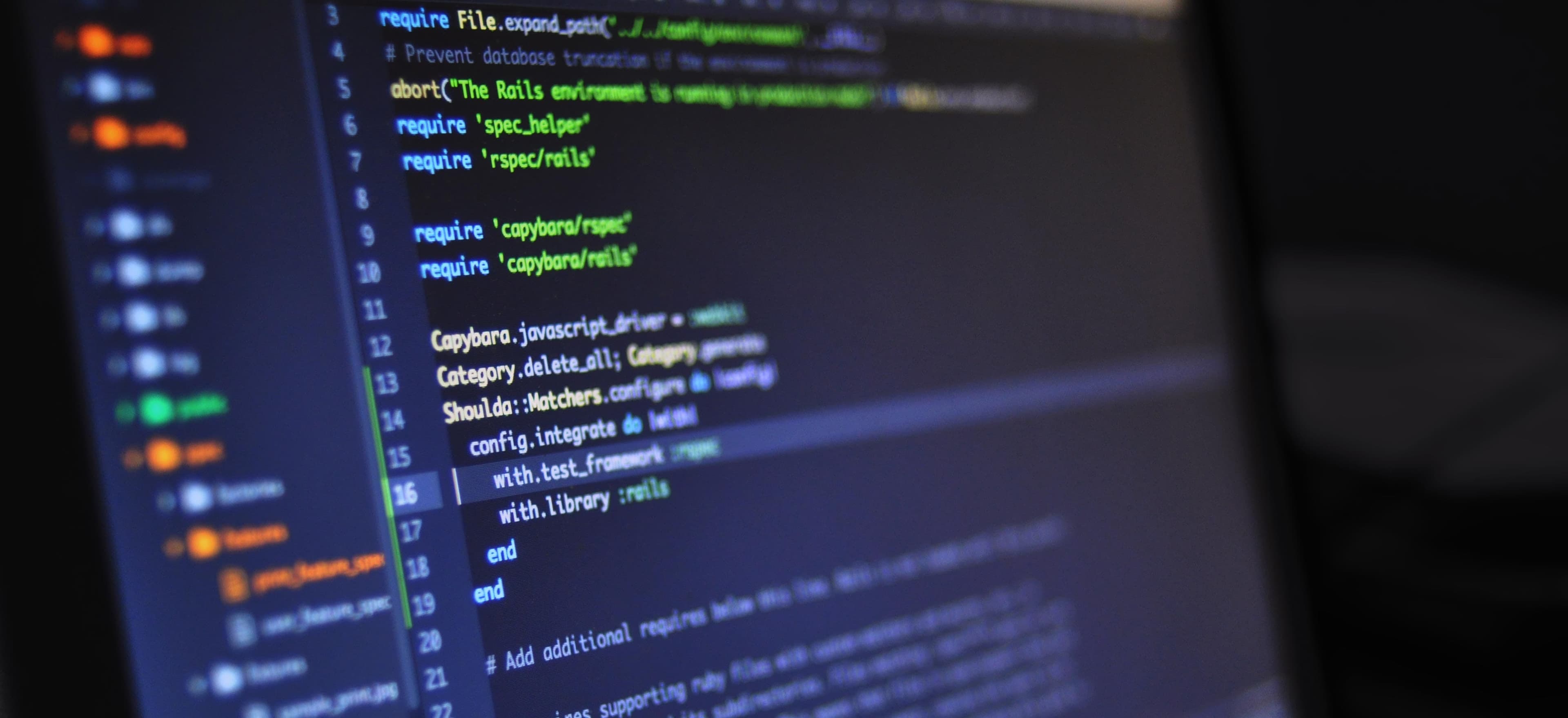
- Published on
Overcoming Type Erasure in Java Generics Polymorphism
Java is a powerful and versatile programming language, known for its robust support for generics. Generics allow developers to create classes, interfaces, and methods that operate on objects of various types, while providing compile-time type safety. However, Java's generics are subject to a concept known as type erasure, which can present challenges when working with polymorphism. In this blog post, we'll explore the concept of type erasure, its implications for polymorphism, and strategies for overcoming these challenges in Java.
Understanding Type Erasure
Java's generics are implemented using type erasure, which means that the type parameters used in generic classes and methods are erased at compile time. This allows for compatibility with pre-existing code that does not use generics, as the generated bytecode will not contain any information about the generic types.
For example, consider the following generic class:
public class Box<T> {
private T value;
public void setValue(T value) {
this.value = value;
}
public T getValue() {
return value;
}
}
When compiled, the bytecode for the Box
class contains no information about the type parameter T
. As a result, at runtime, the JVM has no knowledge of the generic type used, and all instances of Box
will effectively be treated as if they are Box<Object>
.
Implications for Polymorphism
Type erasure can have implications for polymorphism, which is the ability for different classes to be treated as instances of a common superclass during runtime. Consider the following scenario:
public class FruitBox<T extends Fruit> {
private T fruit;
public void setFruit(T fruit) {
this.fruit = fruit;
}
public T getFruit() {
return fruit;
}
public void displayFruitInfo() {
System.out.println("Fruit type: " + fruit.getClass().getSimpleName());
}
}
public class Apple extends Fruit {
// Apple-specific implementation
}
public class Orange extends Fruit {
// Orange-specific implementation
}
In this example, FruitBox
is a generic class constrained to types that extend Fruit
. We have Apple
and Orange
classes that extend Fruit
. If we create instances of FruitBox<Apple>
and FruitBox<Orange>
, Java's type erasure will cause both instances to be treated as FruitBox<Fruit>
at runtime, effectively losing the specific type information.
Overcoming Type Erasure with Wildcards
To overcome the challenges posed by type erasure in the context of polymorphism, Java provides the concept of wildcards. Wildcards allow for more flexibility when working with generic types, enabling the specification of unknown types or ranges of types.
Unbounded Wildcards
An unbounded wildcard is denoted by the ?
symbol and represents an unknown type. It can be used to process instances of generic classes without knowing their specific type. Consider the following example:
public void processFruitBox(FruitBox<?> fruitBox) {
// Perform operations without knowing the exact type of fruits inside the box
}
In this example, FruitBox<?>
denotes a FruitBox
of unknown type. This allows us to process the FruitBox
without needing to know the specific type of fruits it contains.
Bounded Wildcards
In addition to unbounded wildcards, Java also supports bounded wildcards, which allow for the specification of certain bounds or restrictions on the type. Bounded wildcards are denoted using the extends
and super
keywords.
Upper Bounded Wildcards
An upper bounded wildcard, denoted by <? extends T>
, restricts the unknown type to be a specific type or any of its subtypes. This allows for flexibility when working with polymorphic behavior. Consider the following example:
public void processFruitBoxWithUpperBound(FruitBox<? extends Fruit> fruitBox) {
// Perform operations knowing that the fruits inside the box are of type Fruit or its subtypes
}
In this example, FruitBox<? extends Fruit>
allows us to process a FruitBox
containing fruits of type Fruit
or any of its subtypes.
Lower Bounded Wildcards
A lower bounded wildcard, denoted by <? super T>
, restricts the unknown type to be a specific type or any of its supertypes. This can be useful in scenarios where we need to add elements to a collection. Consider the following example:
public void addFruitToFruitBoxWithLowerBound(FruitBox<? super Apple> fruitBox) {
fruitBox.setFruit(new Apple());
// This method allows adding instances of Apple or its subtypes to the fruit box
}
In this example, FruitBox<? super Apple>
allows us to add fruits of type Apple
or any of its subtypes to the FruitBox
.
Overcoming Type Erasure with Reflection
Another approach to overcoming the limitations of type erasure in Java is to use reflection. Reflection allows for the inspection and manipulation of classes, interfaces, fields, and methods at runtime, enabling access to type information that is not available through regular generic constructs.
Capturing Generic Type Information with Reflection
By using reflection, it is possible to capture generic type information at runtime, despite the effects of type erasure. Consider the following example:
import java.lang.reflect.ParameterizedType;
import java.lang.reflect.Type;
public class TypeCapture<T> {
private final Class<T> type;
@SuppressWarnings("unchecked")
public TypeCapture() {
Type superClass = getClass().getGenericSuperclass();
this.type = (Class<T>) ((ParameterizedType) superClass).getActualTypeArguments()[0];
}
public Class<T> getType() {
return type;
}
}
In this example, the TypeCapture
class captures the generic type information at runtime using reflection. By accessing the class's generic superclass and extracting the actual type arguments, we are able to obtain the generic type information that would otherwise be lost due to type erasure.
In Conclusion, Here is What Matters
In conclusion, type erasure in Java generics presents challenges when working with polymorphism, as the specific type information is lost at runtime. However, by leveraging wildcards and reflection, it is possible to overcome these challenges and maintain the benefits of polymorphic behavior while working with generic types.
Additional Resources
To further explore the concepts discussed in this blog post, you may find the following resources helpful:
Thank you for reading! We hope this blog post has provided valuable insights into overcoming type erasure in Java generics polymorphism. If you have any questions or feedback, please feel free to reach out. Happy coding!