Building a Mini Search Engine: Tackling Data Structure
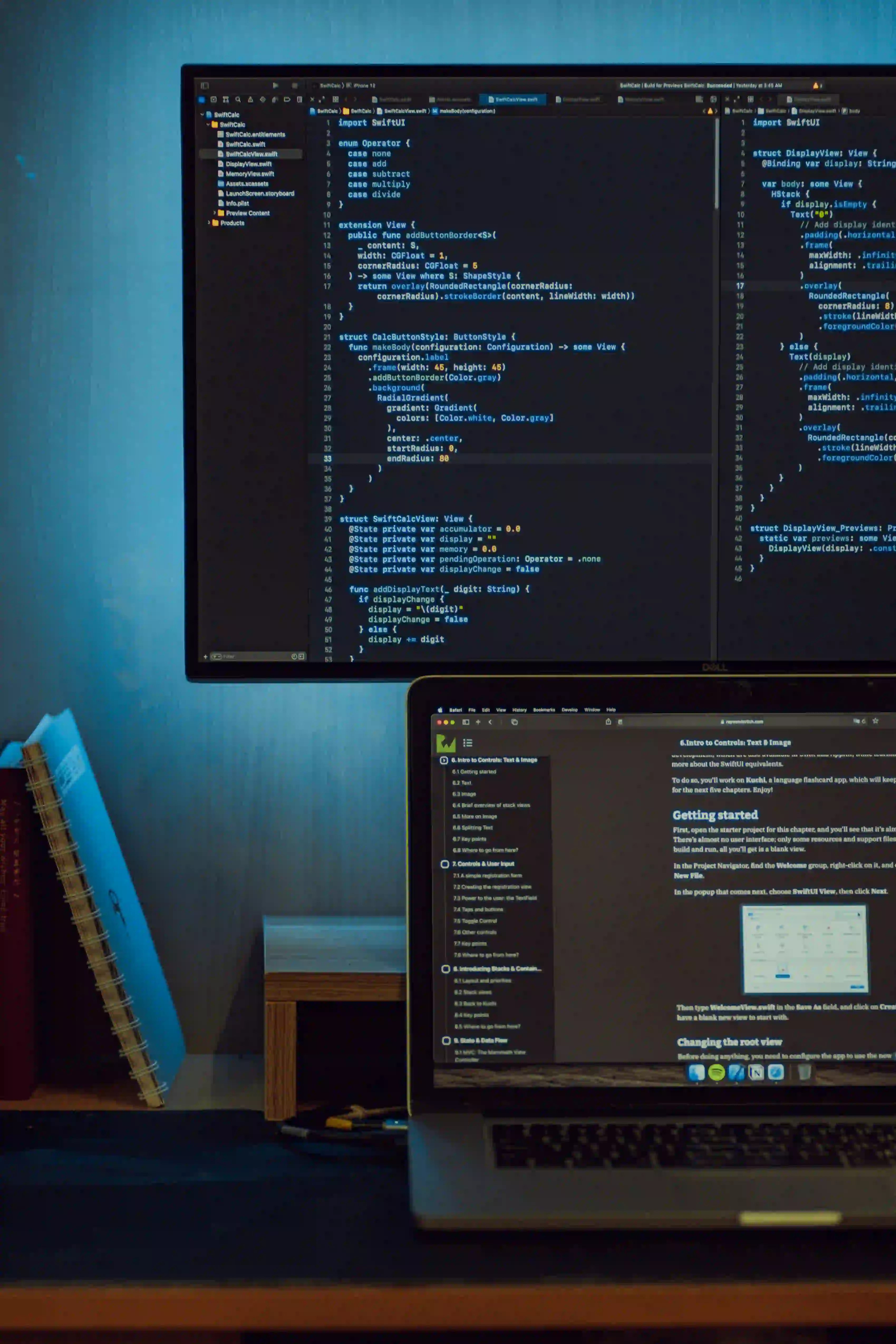
Building a Mini Search Engine: Tackling Data Structure
In the world of programming, the efficient management of data plays a pivotal role. When it comes to building a search engine, the importance of data structure cannot be overstated. In this blog post, we will delve into the essential data structures and algorithms that form the backbone of a mini search engine using Java. By understanding the intricacies of these data structures, you can lay a solid foundation for creating an efficient and scalable search engine.
Why Data Structure Matters in Search Engine Development
Data structures are critical in search engine development due to the need for organizing and retrieving vast amounts of data with optimal efficiency. Search engines deal with indexing and querying substantial volumes of data, and the choice of data structures directly impacts the speed and accuracy of search results. Efficient data structures enable quicker retrieval and processing of data, ultimately enhancing the overall performance of the search engine.
The Role of Java in Search Engine Development
Java, with its robust standard library and support for data structures, is an ideal choice for building a search engine. Its platform independence and extensive ecosystem of libraries make it well-suited for handling the complex requirements of search engine development. Utilizing Java's rich set of data structure implementations allows for streamlined handling of data, making it a top contender for this purpose.
Essential Data Structures for Search Engine Development
1. Trie Data Structure
The Trie data structure is an efficient retrieval tree structure used for storing and searching a dynamic set of strings. In the context of a search engine, Tries are particularly useful for prefix-based string matching and autocomplete features. Implementing Trie in Java involves the use of nested HashMaps or arrays to represent the hierarchical nature of the data.
class TrieNode {
Map<Character, TrieNode> children;
boolean isEndOfWord;
public TrieNode() {
children = new HashMap<>();
isEndOfWord = false;
}
}
The above code snippet illustrates a simple implementation of a TrieNode in Java. The use of HashMap for children nodes allows for efficient storage and retrieval of words in the Trie data structure.
2. Inverted Index
Inverted Index is another fundamental data structure in search engines, which maps content to its location. It consists of a mapping between words and the documents in which they occur. In Java, this can be implemented using HashMaps or TreeMap for efficient storing and retrieval of the index.
Map<String, Set<String>> invertedIndex = new HashMap<>();
Here, the invertedIndex
maps each word to a set of documents where the word appears. This allows for quick lookup of documents containing specific words during the search process.
3. Priority Queue
A Priority Queue is crucial for ranking search results based on certain criteria. In Java, the PriorityQueue class provides an efficient implementation of a priority queue, allowing for the ordering of elements based on their natural ordering or a specified comparator. This is indispensable for presenting the most relevant search results to the user.
Queue<Document> priorityQueue = new PriorityQueue<>(Comparator.comparingDouble(Document::getRelevance));
The above code snippet demonstrates the use of PriorityQueue to prioritize search results based on the relevance of the documents.
Incorporating Algorithms for Search Engine Functionality
In addition to data structures, search engines rely on various algorithms for indexing, ranking, and retrieving content. Some essential algorithms include:
1. PageRank Algorithm
The PageRank algorithm, initially developed by Google, assigns a numerical weighting to each element of a hyperlinked set of documents, with the purpose of measuring its relative importance within the set. Implementing the PageRank algorithm involves a combination of graph and probability theory, which can be efficiently executed using Java's graph processing libraries such as JGraphT.
2. Vector Space Model
The Vector Space Model is a mathematical model used to represent text documents as vectors, enabling the calculation of similarity between documents. This model incorporates algorithms for measuring the relevance of documents to a search query, such as cosine similarity. Implementing this model in Java involves vector operations and mathematical computations using libraries like Apache Commons Math.
Closing Remarks
In the realm of search engine development, data structures form the backbone on which efficient algorithms operate. With Java's versatile support for a wide range of data structures and algorithms, it becomes an indispensable tool for building a mini search engine. By leveraging the discussed data structures and algorithms, you can embark on the journey of creating a powerful and responsive search engine in Java.
Building a search engine from the ground up may seem like a daunting task, but with a solid understanding of data structures and algorithms, coupled with the versatility of Java, you are well-equipped to take on the challenge.
Start exploring the world of search engine development with Java, and witness the transformative impact of effective data structures in action. Happy coding!
Remember to check out the official Java documentation for detailed insights into Java's data structures and algorithms.