Mastering IPC in Android: Fixing Messenger Glitches
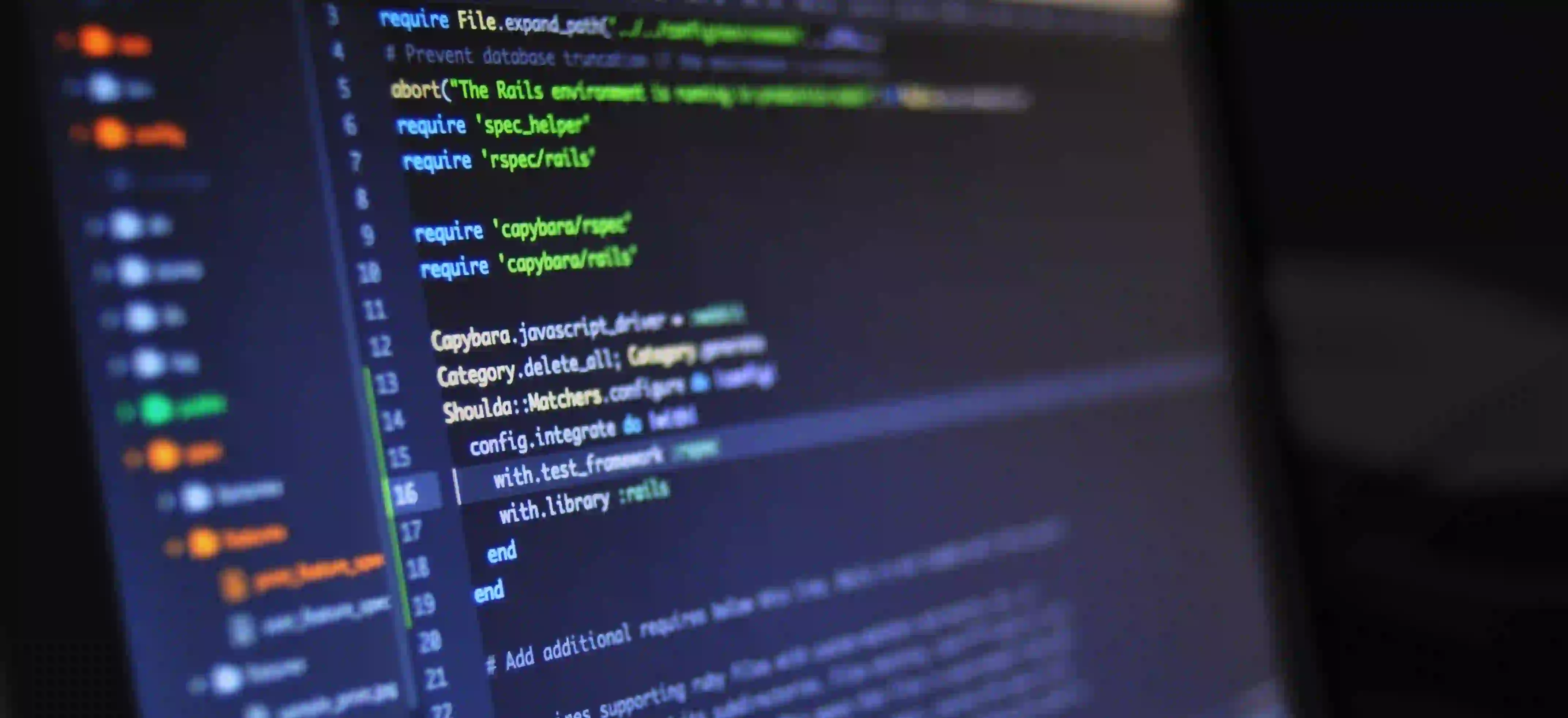
Mastering Inter-Process Communication in Android: Troubleshooting Messenger Defects
Inter-process communication (IPC) is an essential aspect of Android app development to enable different processes to communicate with each other. One common method of IPC in Android is through the use of the Messenger
class. However, when implementing Messenger
in Android apps, developers may encounter certain glitches that impact the performance and functionality of the app.
In this article, we will explore common glitches associated with Messenger
in Android and provide effective solutions to fix these issues. By mastering IPC in Android and addressing Messenger
defects, developers can ensure the seamless communication between processes within their apps.
The Role of Messenger in Android IPC
The Messenger
class in Android provides a mechanism for performing IPC between processes. It acts as a simple wrapper around a Handler
and allows messages to be sent and processed across different processes. Using Messenger
is beneficial for scenarios where inter-process communication is required, such as when working with background services, remote services, or multiple app components.
Identifying Common Messenger Glitches
Before delving into the solutions, let’s identify some common glitches that developers may encounter when utilizing Messenger
in their Android apps:
1. Dead Object Exception
When sending a message using Messenger
, a "DeadObjectException" may occur if the targeted Messenger
is no longer valid. This can happen when the target process has crashed or been terminated.
2. Message Loss
In some cases, messages sent via Messenger
may get lost during communication between processes, leading to incomplete or inconsistent behavior within the app.
3. Threading Issues
Improper threading within Messenger
implementation can lead to concurrency issues, such as deadlocks or race conditions, impacting the reliability and performance of the IPC.
Fixing Messenger Glitches
Now, let’s dive into the solutions for addressing the identified glitches when using Messenger
in Android app development.
1. Handling Dead Object Exception
To handle the Dead Object Exception, it is crucial to establish a robust mechanism for detecting and re-establishing communication with the target Messenger
. This can be achieved by implementing the following steps:
Using a ServiceConnection
private ServiceConnection mConnection = new ServiceConnection() {
@Override
public void onServiceConnected(ComponentName className, IBinder service) {
mServiceMessenger = new Messenger(service);
}
@Override
public void onServiceDisconnected(ComponentName className) {
mServiceMessenger = null;
// Re-bind to the service or take appropriate action
// for re-establishing communication.
}
};
In the onServiceDisconnected
method, developers can implement the logic to re-bind to the service or take appropriate actions for re-establishing communication with the target Messenger
.
2. Ensuring Message Reliability
To address message loss and reliability issues, applying proper acknowledgment mechanisms within the messaging protocol can help ensure reliable message delivery. This involves confirming the receipt of messages and re-sending them if necessary.
Implementing Acknowledgment Mechanism
// Sender side
Message message = Message.obtain(null, MSG_DATA);
message.replyTo = mReplyMessenger;
mServiceMessenger.send(message);
// Receiver side
public void handleMessage(Message msg) {
// Handle the received message
// Send acknowledgment to the sender if required
if (acknowledgmentNeeded) {
Message ackMessage = Message.obtain(null, MSG_ACK);
msg.replyTo.send(ackMessage);
}
}
By implementing an acknowledgment mechanism, the sender can ensure that the message has been successfully received and processed by the recipient.
3. Managing Threading Issues
To prevent threading issues within the Messenger
implementation, it is essential to adhere to the following best practices:
-
Use Handlers for Asynchronous Messaging: Utilize handlers to process messages asynchronously, avoiding potential threading conflicts.
-
Avoid Long-Running Operations on the Main Thread: Offload time-consuming operations to background threads to prevent UI thread blocking.
-
Synchronize Access to Shared Resources: When accessing shared resources across threads, employ synchronization mechanisms to avoid data inconsistencies.
Wrapping Up
Mastering IPC in Android, especially when using the Messenger
class, is crucial for seamless communication between processes within an app. By addressing common glitches such as Dead Object Exceptions, message loss, and threading issues, developers can ensure the reliability, performance, and stability of their Android apps.
Implementing robust error-handling mechanisms, ensuring message reliability, and managing threading issues are key steps towards fixing Messenger
glitches and mastering IPC in Android.
In conclusion, by adopting these solutions and best practices, developers can elevate their skills in IPC and confidently navigate the intricacies of inter-process communication in Android app development.
To further enhance your understanding of IPC in Android and the Messenger
class, refer to the official Android Developer documentation and resources such as Android Developer Guide on Messenger and Android IPC Overview for comprehensive insights. Happy coding!