Connecting MongoDB with Java: Best Practices
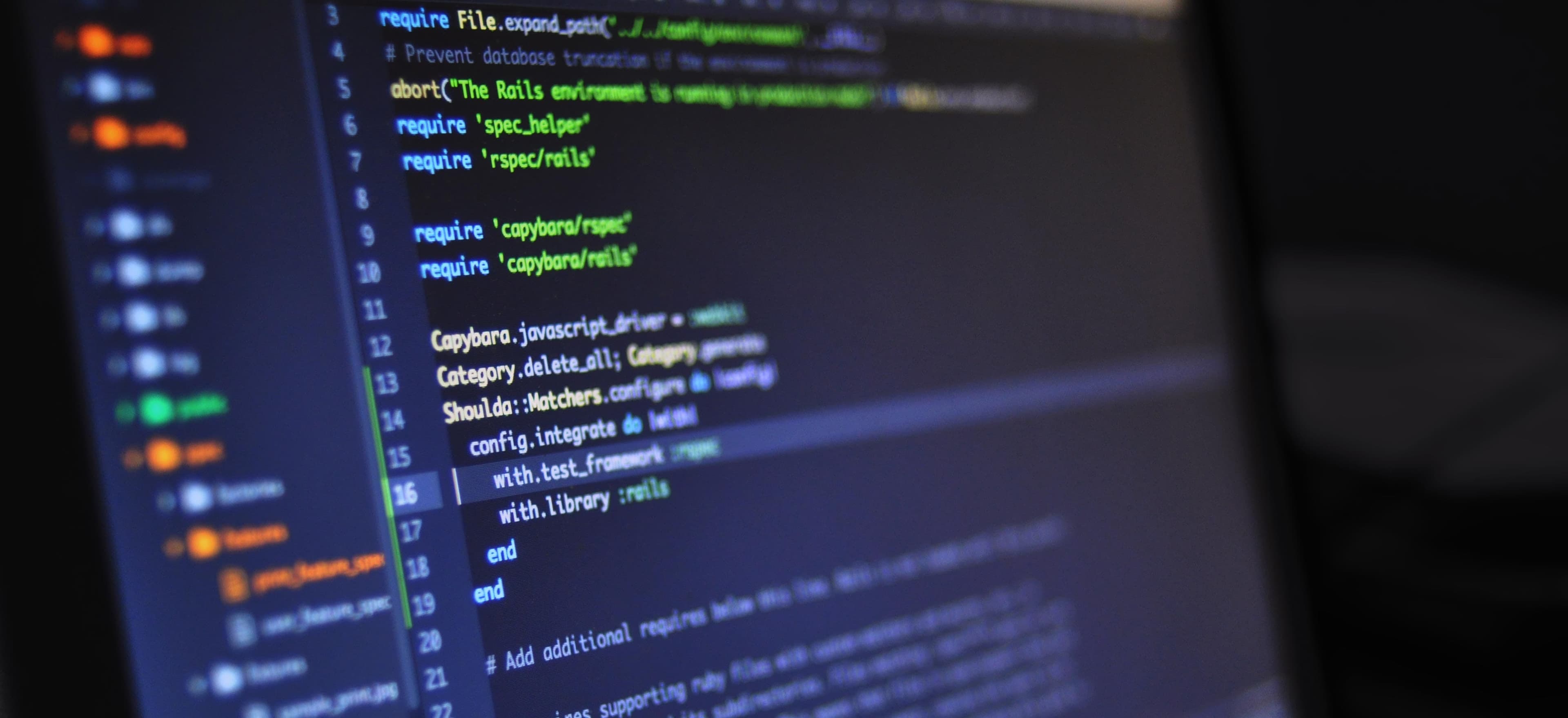
- Published on
A Complete Guide on Connecting MongoDB with Java
In the era of big data and dynamic applications, MongoDB has gained significant popularity due to its flexibility and scalability. As a NoSQL database, it offers a document-oriented data model, making it suitable for a wide range of use cases. If you're a Java developer looking to integrate MongoDB into your projects, this guide will walk you through the best practices for connecting MongoDB with Java.
Why Use MongoDB with Java?
Before diving into the technical aspects, let's understand why using MongoDB with Java is a compelling choice. MongoDB's flexible schema and document-based structure align well with Java's object-oriented nature, facilitating seamless integration and data manipulation. Furthermore, MongoDB's scalability and high-performance capabilities make it an excellent fit for Java applications dealing with large volumes of data.
Setting Up the MongoDB Java Driver
The first step in connecting MongoDB with Java is to include the MongoDB Java driver in your project. The official MongoDB Java driver provides a high-performance, asynchronous API for interacting with MongoDB.
Maven Dependency
To include the MongoDB Java driver in a Maven project, add the following dependency to your pom.xml
file:
<dependency>
<groupId>org.mongodb</groupId>
<artifactId>mongodb-driver-sync</artifactId>
<version>4.4.2</version>
</dependency>
Gradle Dependency
For a Gradle-based project, include the dependency in the build.gradle
file:
implementation group: 'org.mongodb', name: 'mongodb-driver-sync', version: '4.4.2'
Connecting to a MongoDB Database
Now, let's look at how to establish a connection to a MongoDB database from your Java application.
Connecting to a Standalone MongoDB Instance
When connecting to a standalone MongoDB instance, you can use the following code to create a connection:
import com.mongodb.ConnectionString;
import com.mongodb.client.MongoClients;
import com.mongodb.client.MongoClient;
import com.mongodb.client.MongoDatabase;
public class MongoDBConnection {
public static void main(String[] args) {
String connectionString = "mongodb://localhost:27017"; // Replace with your MongoDB URI
try (MongoClient mongoClient = MongoClients.create(connectionString)) {
MongoDatabase database = mongoClient.getDatabase("your-database-name");
System.out.println("Connected to the database successfully");
} catch (Exception e) {
System.err.println("An error occurred: " + e);
}
}
}
In the above code, we use the MongoClients.create
method to create a connection to the MongoDB instance specified in the connection string.
Connecting to a MongoDB Replica Set or Cluster
If you're connecting to a replica set or a sharded cluster, you can modify the connection string to include the appropriate details, such as replica set members or sharding configuration.
String connectionString = "mongodb://host1:27017,host2:27017,host3:27017/?replicaSet=my-replica-set";
Always handle exceptions and close your connections properly for robust and efficient resource management.
Managing MongoDB Connection String
Using a connection string to connect to a MongoDB database is a best practice as it encapsulates all the connection details in a single URI. This approach not only simplifies the connection setup but also allows for more flexibility in managing connection options, such as authentication, SSL/TLS configuration, and connection pooling.
MongoDB connection strings adhere to a standard format and support a wide range of options, allowing you to customize the behavior of the MongoDB Java driver according to your application's requirements. You can find comprehensive documentation on MongoDB connection string options here.
Ensuring Thread Safety
When working with MongoDB in a multi-threaded environment, it's crucial to ensure thread safety. Fortunately, the MongoDB Java driver is designed to be thread-safe, allowing multiple threads to share a single MongoClient instance. This promotes efficient resource utilization and simplifies connection management.
Consider creating a singleton MongoClient instance to be shared across your application, ensuring that all threads utilize the same connection pool and configuration. This approach minimizes the overhead of creating multiple connections and contributes to better performance.
public class MongoDBConnectionManager {
private static final String connectionString = "mongodb://localhost:27017";
private static MongoClient mongoClient = null;
public static synchronized MongoClient getMongoClient() {
if (mongoClient == null) {
mongoClient = MongoClients.create(connectionString);
}
return mongoClient;
}
}
In the above code, the getMongoClient
method retrieves the shared MongoClient instance, creating it if it doesn't exist. The synchronized
keyword ensures that the method is thread-safe and that only one instance of MongoClient is created in a multi-threaded environment.
My Closing Thoughts on the Matter
In this guide, we've explored the best practices for connecting MongoDB with Java, covering essential topics such as setting up the MongoDB Java driver, establishing connections, managing connection strings, and ensuring thread safety. By following these best practices, you can seamlessly integrate MongoDB into your Java applications while leveraging its full potential for data storage and retrieval.
Embracing MongoDB's document-oriented approach and Java's object-oriented paradigm empowers developers to build robust, scalable, and high-performing applications. As you embark on your journey of integrating MongoDB with Java, remember to adhere to best practices, stay updated with the latest MongoDB Java driver releases, and explore additional features and optimizations offered by MongoDB for Java developers.