Thread Pool Overload: How Many Threads Is Too Many?
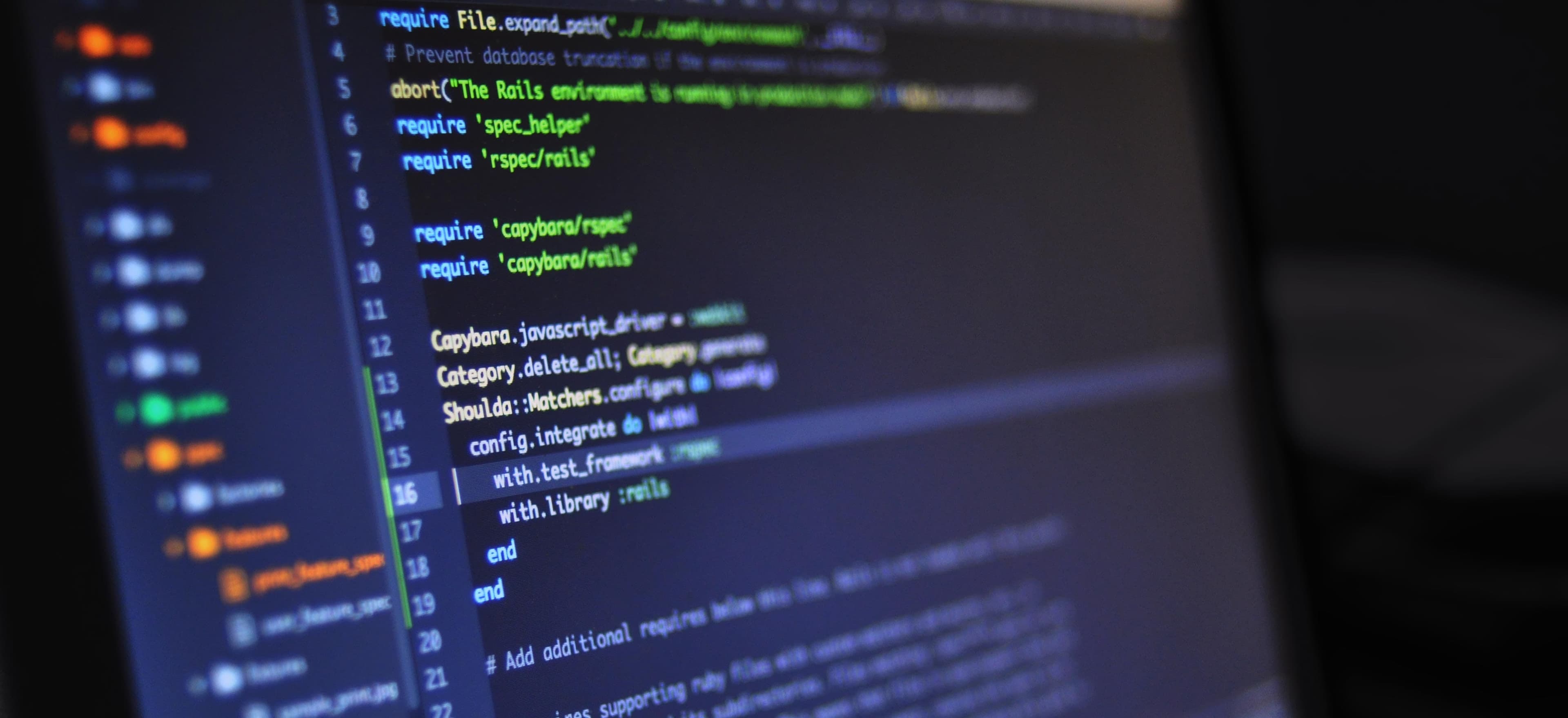
- Published on
Thread Pool Overload: How Many Threads Is Too Many in Java?
Multithreading in Java is a powerful mechanism allowing programmers to achieve parallelism and concurrency in their applications. However, with great power comes great responsibility. Overloading a thread pool can lead to various issues, including increased memory consumption, higher context-switching overheads, and ultimately, degraded application performance. In this comprehensive guide, we'll explore the intricacies of correctly sizing a thread pool, why it matters, and how to determine the optimal number of threads for your Java applications.
Understanding Thread Pools in Java
Before diving into the specifics of thread pool overload, let's first understand what thread pools are and why they're used. In Java, a thread pool is a collection of pre-instantiated reusable threads. These threads can be used to execute tasks concurrently. The primary advantage of using a thread pool is to minimize the overhead associated with thread creation, especially in applications that execute a large number of short-lived asynchronous tasks.
Java provides several thread pool implementations in the java.util.concurrent
package, such as the ExecutorService
framework, which allows for fine-grained control over thread management.
Why Size Matters
The size of a thread pool directly affects an application's performance and resource efficiency. A thread pool that's too large can waste valuable system resources, while a thread pool that's too small may cause underutilization of CPU resources, leading to suboptimal performance.
Identifying the Optimal Thread Pool Size
Determining the optimal thread pool size can be challenging, as it depends on various factors, including:
- CPU Utilization: The number of available CPU cores.
- Task Nature: The nature of the tasks being executed (CPU-bound vs. IO-bound tasks).
- System Resources: The memory and other system resources available.
A common formula that's often quoted for calculating the optimal number of threads is:
Number of Threads = Number of Available Cores * (1 + Waiting Time / Service Time)
Where Waiting Time is the average time a thread spends waiting for IO (or other resources), and Service Time is the average time a thread spends computing.
Avoiding Thread Pool Overload
To prevent thread pool overload, it's crucial to dynamically adjust the thread pool size based on the application's current state and the characteristics of the tasks being executed. Here's an example snippet using Executors.newFixedThreadPool(nThreads)
to create a fixed-size thread pool:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ThreadPoolExample {
public static void main(String[] args) {
// Determine the number of available processors
int nThreads = Runtime.getRuntime().availableProcessors();
ExecutorService executor = Executors.newFixedThreadPool(nThreads);
for (int i = 0; i < 100; i++) {
Runnable task = new WorkerThread(i);
executor.execute(task);
}
executor.shutdown();
}
}
class WorkerThread implements Runnable {
private int taskNumber;
WorkerThread(int taskNumber) {
this.taskNumber = taskNumber;
}
public void run() {
System.out.println("Executing task number: " + taskNumber + " " +
"on thread: " + Thread.currentThread().getName());
// Task implementation
}
}
In this example, we dynamically determine the number of threads in the pool based on the number of available processors. This is a basic approach and doesn't account for the nature of the tasks (CPU-bound vs. IO-bound), which should also be considered for optimal performance.
Advanced Techniques
For more complex scenarios, Java offers robust frameworks like ThreadPoolExecutor
and ForkJoinPool
that provide more granular control over thread management and task execution.
ThreadPoolExecutor
, for example, allows developers to configure core and maximum pool sizes, keep-alive times, and a blocking queue for tasks waiting to be executed. This flexibility is crucial for applications with varying workloads and performance requirements.
Further Reading
To deepen your understanding of Java's concurrency tools and thread management, the following resources are invaluable:
My Closing Thoughts on the Matter
Effectively managing thread pool size is crucial for Java applications to leverage the full potential of system resources while maintaining high performance and responsiveness. By understanding the underlying factors that affect thread pool sizing and leveraging Java's ExecutorService
framework, developers can make informed decisions to optimize thread pool usage.
Remember, there's no one-size-fits-all answer to "How many threads is too many?" It's about striking the right balance based on your application's specific needs, the nature of the tasks, and the underlying system architecture. By adhering to best practices and continuously monitoring and adjusting your thread pool strategy, you'll be well on your way to achieving efficient and effective multithreading in your Java applications.