Simplifying Spring MVC: Crafting the Perfect Controller
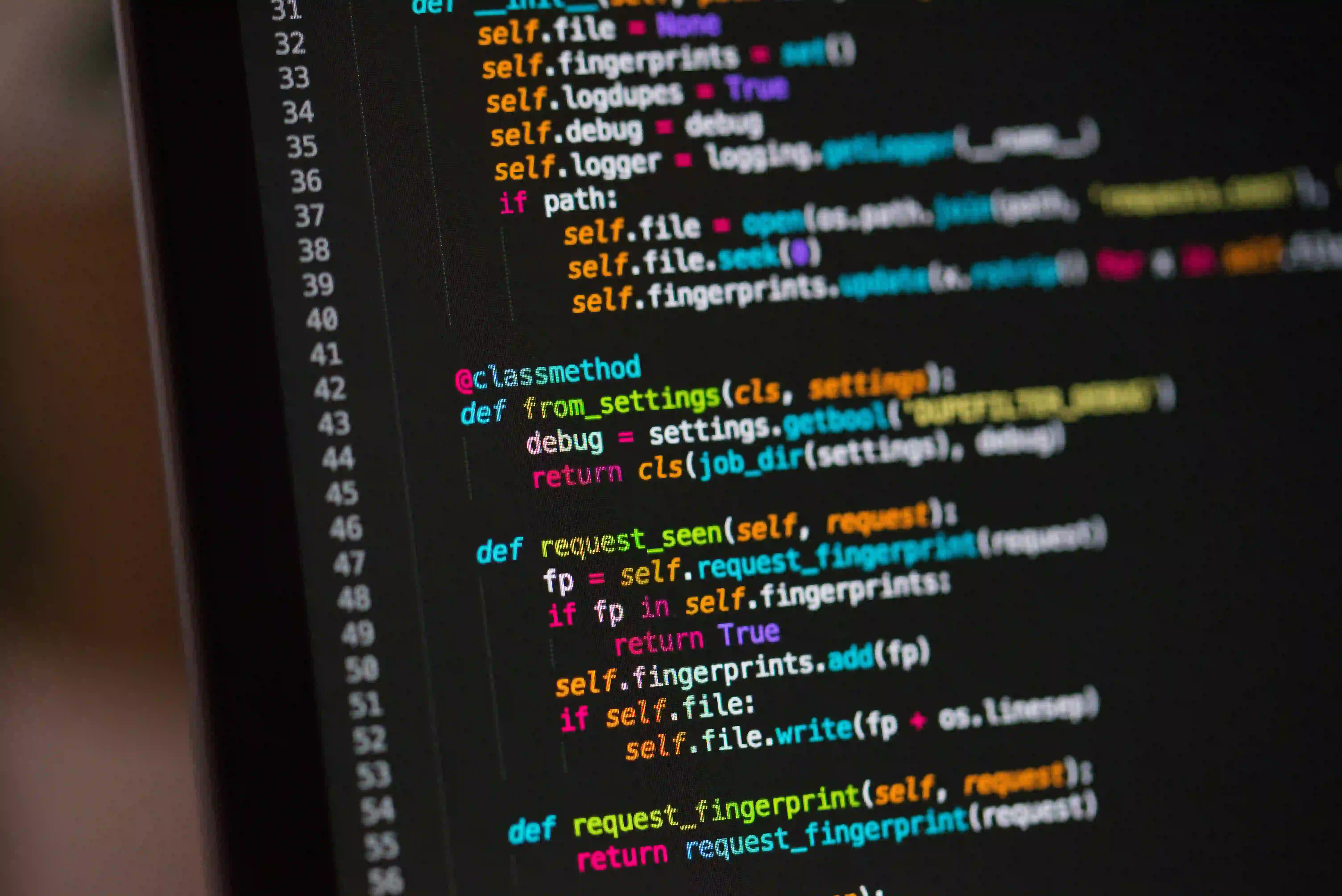
Simplifying Spring MVC: Crafting the Perfect Controller
When it comes to building a web application using Java, Spring MVC stands as one of the most popular and powerful frameworks. The MVC architecture pattern separates an application into three main components: Model, View, and Controller. In this article, we'll delve into the intricacies of Spring MVC controllers, discussing how to craft the perfect controller for your applications.
Understanding the Role of a Controller
In the MVC pattern, the controller serves as the crucial link between the user's interactions and the application's behavior. It receives input, processes it through the model, and eventually provides the appropriate response. In Spring MVC, a controller is a Java class annotated with @Controller
and methods annotated with @RequestMapping
to map the incoming requests to the corresponding methods.
Simplifying the Controller Logic
1. Embracing Annotations
Gone are the days of implementing cumbersome interfaces or extending base classes. With Spring MVC, you can simply annotate a class with @Controller
and benefit from robust functionality, effortlessly.
@Controller
public class MyController {
@RequestMapping("/hello")
public String greet() {
return "hello";
}
}
By declaring the class with @Controller
, Spring automatically detects and registers it as a controller, while @RequestMapping
specifies the URL path to which the method responds.
2. Utilizing Request Mapping
Spring MVC's @RequestMapping
annotation allows you to map URL patterns directly to controller methods, simplifying the routing logic by intrinsic association.
@Controller
@RequestMapping("/products")
public class ProductController {
@RequestMapping("/{id}")
public String getProduct(@PathVariable("id") int productId, Model model) {
// Retrieve product details using the productId
return "productDetails";
}
}
Here, the @RequestMapping
annotation at the class level indicates the base path for all methods within the controller, while the method-level @RequestMapping
maps the {id}
path variable to the getProduct
method.
3. Leveraging Path Variables
In Spring MVC, path variables are denoted by the @PathVariable
annotation, enabling the extraction of data directly from the URL. This streamlines the process of passing dynamic values to controller methods.
@GetMapping("/products/{id}")
public String getProduct(@PathVariable Long id, Model model) {
// Fetch and populate product details
return "productDetails";
}
The @PathVariable
annotation binds the id
variable in the URL to the method parameter, obviating the need for manual extraction from the request URL.
Enhancing Efficiency with Request Parameters
1. Accessing Query Parameters
With Spring MVC, retrieving query parameters from the request is a seamless endeavor. Through the @RequestParam
annotation, you can directly bind URL query parameters to method parameters.
@GetMapping("/search")
public String searchProduct(@RequestParam("keyword") String keyword, Model model) {
// Perform product search based on the provided keyword
return "searchResults";
}
By specifying @RequestParam("keyword")
, the searchProduct
method seamlessly receives the query parameter keyword
from the request URL.
2. Embracing Default Parameter Values
In scenarios where specific query parameters might not be present, employing default parameter values simplifies the handling process.
@GetMapping("/search")
public String searchProduct(@RequestParam(name = "keyword", defaultValue = "all") String keyword, Model model) {
// Handle the 'all' scenario or proceed with the provided keyword
return "searchResults";
}
By assigning a default value to the keyword
parameter, the application gracefully accommodates cases where the query parameter is absent, sparing the need for additional conditional checks within the method.
Centralizing Common Functionality with Advice
1. Introducing Controller Advice
In Spring MVC, controller advice allows for the centralization of common functionalities such as exception handling or data binding across multiple controllers, promoting code reusability and maintainability.
@ControllerAdvice
public class GlobalControllerAdvice {
@ExceptionHandler(Exception.class)
public String handleGlobalException(Exception ex, Model model) {
// Handle and log the exception
return "error";
}
}
By annotating a class with @ControllerAdvice
, you can define methods that are globally applicable to all controllers. In the example, the handleGlobalException
method sensibly manages exceptions across the application.
A Final Look
Spring MVC's controller plays an integral role in shaping the user experience of an application, and simplifying its development and maintenance is paramount. By embracing the annotations and features provided by Spring MVC, you can streamline controller logic, enhance efficiency, and centralize common functionality, thereby crafting the perfect controller for your web application.
In this article, we've explored several techniques to simplify Spring MVC controller development, ranging from leveraging annotations for routing and parameter handling to centralizing common functionalities with controller advice. By applying these techniques, you can streamline and optimize your controller logic, crafting a robust foundation for your Spring MVC applications.
Intrigued to learn more about Spring MVC? Check out the official Spring MVC documentation for comprehensive insights into its features and capabilities.