Unlocking Metrics: Micrometer in Spring Boot 2 Explained
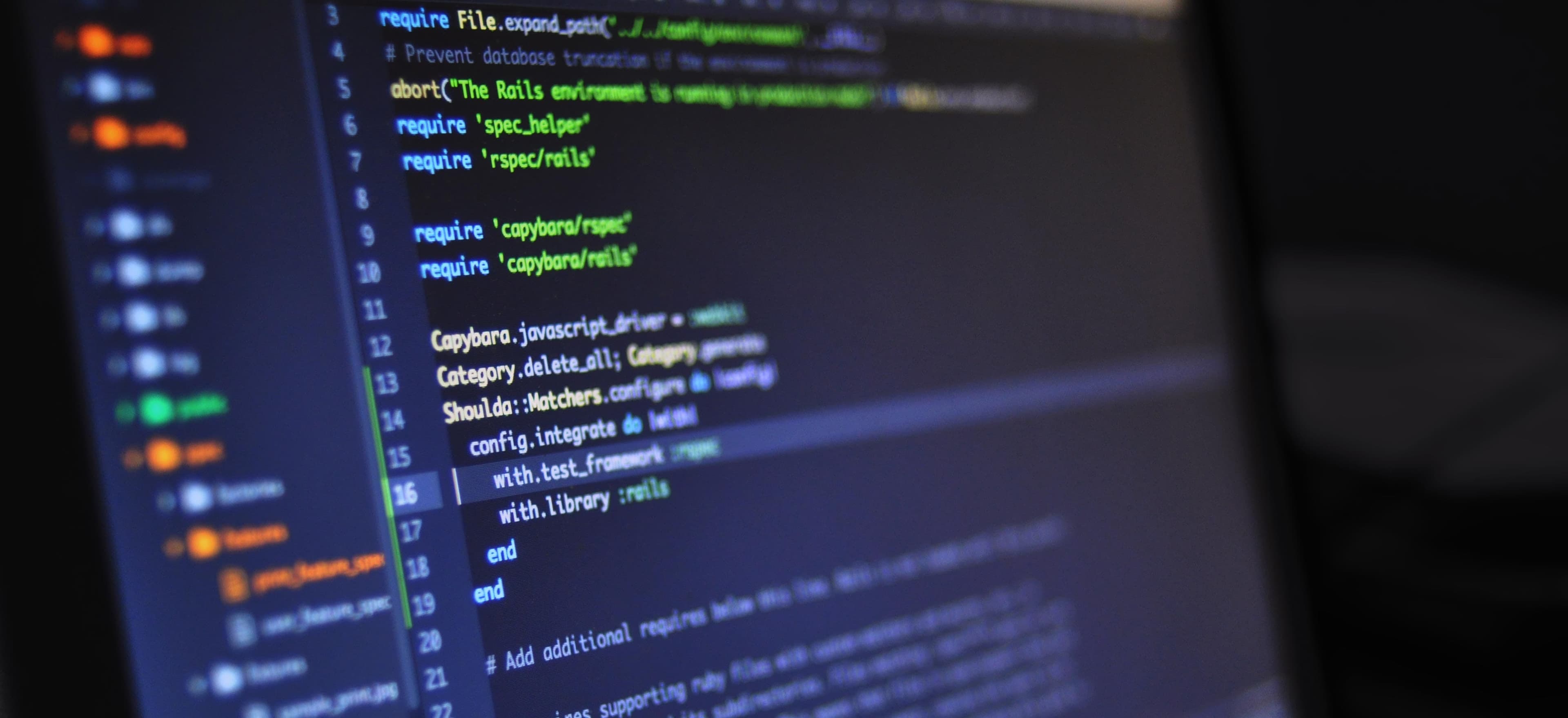
- Published on
Unlocking Metrics: Micrometer in Spring Boot 2 Explained
In the fast-paced world of software development, it's crucial to have insights into the performance and behavior of our applications. Metrics play a vital role in understanding the health and efficiency of our systems. With the rise of microservices and distributed systems, the need for a standardized approach to gathering and exposing metrics has become increasingly important.
In this blog post, we will delve into the world of Micrometer, a powerful library for application metrics instrumentation, and explore how it seamlessly integrates with Spring Boot 2. We'll cover the fundamentals of Micrometer, its integration with various monitoring systems, and demonstrate how to leverage its capabilities in a Spring Boot 2 application.
What is Micrometer?
Micrometer acts as a unifying facade for various monitoring systems, providing a simple and consistent way to instrument application code. It abstracts the complexity of different monitoring systems and allows developers to focus on writing application-specific metrics code without being tied to a particular monitoring system. This means that developers can instrument their code once and then choose which monitoring system to use, whether it's Prometheus, Graphite, Datadog, or any other compatible system supported by Micrometer.
Why Micrometer?
Before Micrometer, integrating an application with multiple monitoring systems required writing code specific to each system. This led to a maintenance nightmare and made it hard to switch between monitoring systems due to the tight coupling of the code with a particular system's APIs.
Micrometer addresses this by providing a vendor-neutral metrics facade, allowing developers to write instrumentation code once and then ship the metrics to the monitoring system of their choice. This greatly simplifies the instrumentation process and promotes portability and flexibility.
Micrometer in Spring Boot 2
Adding Micrometer to a Spring Boot 2 Project
Integrating Micrometer with a Spring Boot 2 project is straightforward. We can do so by adding the Micrometer dependency to the project's pom.xml
(if using Maven) or build.gradle
(if using Gradle):
<dependency>
<groupId>io.micrometer</groupId>
<artifactId>micrometer-registry-prometheus</artifactId>
<version>latest_version</version>
</dependency>
After adding the dependency, Spring Boot auto-configures Micrometer with sensible defaults, making it effortless to start collecting and exposing metrics from our application.
Instrumenting Code with Micrometer
Micrometer provides a rich set of instrumentation APIs to measure things like counters, gauges, timers, histograms, and distribution summaries. Let's take a look at a simple example of how we can instrument our code using Micrometer.
Example 1: Counting HTTP Requests
@RestController
public class ExampleController {
private final Counter httpRequestsCounter;
public ExampleController(MeterRegistry registry) {
this.httpRequestsCounter = Counter.builder("http.requests.total")
.description("Total HTTP requests")
.register(registry);
}
@GetMapping("/example")
public String exampleEndpoint() {
// Increment the counter for each incoming HTTP request
httpRequestsCounter.increment();
return "Example response";
}
}
In this example, we use Micrometer's Counter
to count the total number of HTTP requests received by our ExampleController
. The MeterRegistry
is automatically provided by Spring Boot and acts as a central registry for managing and collecting metrics.
Example 2: Monitoring Method Execution Time
@Service
public class ExampleService {
private final Timer methodExecutionTimer;
public ExampleService(MeterRegistry registry) {
this.methodExecutionTimer = Timer.builder("method.execution.time")
.description("Time taken for method execution")
.register(registry);
}
public void performTask() {
// Record the execution time of the method
methodExecutionTimer.record(() -> {
// Logic to be timed
});
}
}
In this example, we use Micrometer's Timer
to measure the execution time of a method in our ExampleService
. The Timer
automatically records the duration of the provided logic block and exposes it as a metric.
Integration with Monitoring Systems
Micrometer provides seamless integration with various monitoring systems, allowing us to effortlessly export metrics to systems like Prometheus, Graphite, Datadog, and more. To integrate with a specific monitoring system, we need to include the corresponding Micrometer registry dependency in our project.
For example, to integrate with Prometheus, we include the micrometer-registry-prometheus
dependency, as shown earlier. Spring Boot automatically configures the Prometheus registry based on the presence of this dependency.
Closing Remarks
In this post, we've explored the essential concepts of Micrometer and its integration with Spring Boot 2. We've seen how Micrometer acts as a unifying facade for monitoring systems, simplifying the instrumentation process and promoting portability. We've also demonstrated how to instrument code using Micrometer's rich set of APIs and how to seamlessly integrate with monitoring systems like Prometheus.
By incorporating Micrometer into our Spring Boot 2 applications, we gain valuable insights into the behavior and performance of our systems, enabling us to make informed decisions and ensure the health and efficiency of our applications.
With Micrometer, unlocking actionable metrics in our Spring Boot 2 applications has become more accessible and standardized than ever.
Remember, actionable metrics lead to informed decisions. So, go ahead, unlock your metrics with Micrometer, and gain the insights your applications deserve!