Troubleshooting OSGI Bundle Loading Issues
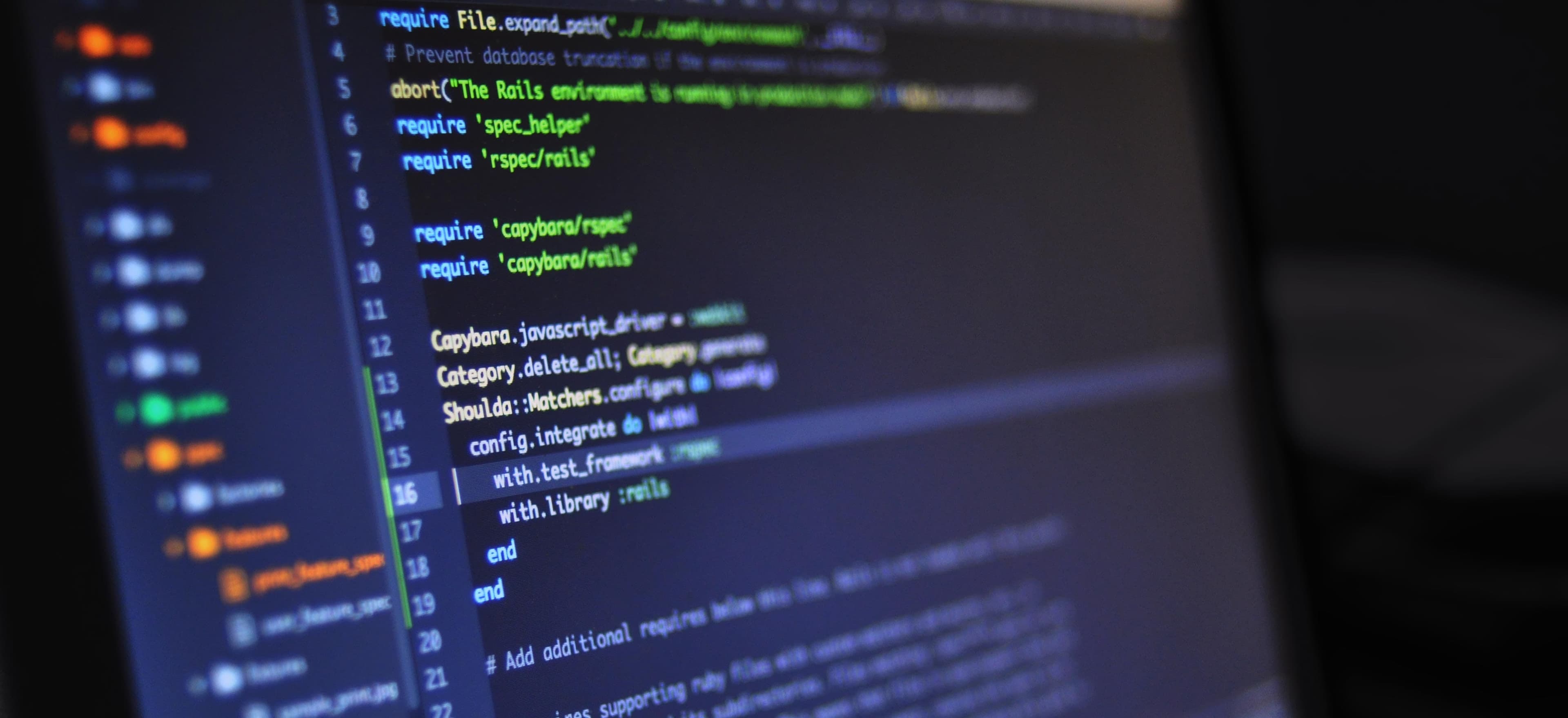
- Published on
Troubleshooting OSGi Bundle Loading Issues
In the realm of Java development, the Open Services Gateway initiative (OSGi) stands out as a pioneering framework that offers a modular approach to building applications. OSGi defines a dynamic component model for Java and is particularly effective for building scalable and maintainable applications. However, managing bundles effectively can sometimes lead to challenges, specifically around bundle loading issues. This blog post will guide you through identifying, analyzing, and resolving common OSGi bundle loading problems.
What is an OSGi Bundle?
An OSGi bundle is essentially a JAR file that contains not only the code but also metadata that describes the bundle and its capabilities. OSGi manages the lifecycle of these bundles, allowing for features such as dynamic updates, versioning, and service-oriented architectures.
Key Features of OSGi Bundles
- Dynamic Loading: Bundles can be started, stopped, installed, and uninstalled without bringing down the entire application.
- Service Registry: Bundles can publish and consume services dynamically.
- Versioning: OSGi supports multiple versions of the same bundle.
Common Bundle Loading Issues
While OSGi is powerful, it can sometimes lead to complexities. Here are some common issues developers face when loading bundles:
- Dependency Conflicts: One of the most frequent issues occurs when two bundles require different versions of the same dependency.
- Missing Requirements: A bundle may fail to load if it requires another bundle that is not currently active or is simply missing.
- Incorrect Manifest Configuration: Any syntactical or logical error in the
MANIFEST.MF
file can prevent the bundle from loading correctly.
Troubleshooting Steps
Step 1: Check the OSGi Console
OSGi provides a console where you can check the status of your bundles. Launch the console and execute the following command:
ss
This command lists all the bundles, their states, and any errors associated with them.
Example:
> ss
ID State Name
0 Active BundleA
1 Active BundleB
2 Resolution BundleC
3 Installed BundleD
If you see the state as "Resolution", it indicates that BundleC is trying to resolve its dependencies but is unable to. This can be a starting point for diagnosing issues.
Step 2: Examine the MANIFEST.MF
The MANIFEST.MF
file is critical in the lifecycle of an OSGi bundle. Here’s what to look for:
- Bundle-ClassPath: Ensure that all paths specified here are correct.
- Import-Package: Verify that any imported packages exist within the available bundles.
- Export-Package: Confirm that the relevant packages are correctly exported by their respective bundles.
Example MANIFEST.MF
Manifest-Version: 1.0
Bundle-ManifestVersion: 2
Bundle-Name: Example Bundle
Bundle-SymbolicName: com.example.bundle
Bundle-Version: 1.0.0
Import-Package: org.example.service;version="[1.0,2.0)"
Export-Package: org.example.api;version="1.0.0"
Wrong package names or versions can lead to issues during loading.
Step 3: Incompatible Versions
If you're dealing with multiple bundles that require different versions of the same package, it can lead to a failure in loading. You can check compatible versions by utilizing Declarative Services
or Blueprint
.
These frameworks allow you to declare a set of requirements elegantly without explicitly managing versioning, but they may not resolve all scenarios.
To troubleshoot manually, ensure the required versions of packages or bundles align properly across your application.
Step 4: Examine the Exception Logs
Whenever a bundle fails to load, OSGi typically logs exceptions that can give clues about what went wrong. Look for error logs in the OSGi container's log files. Logs often include stack traces which can assist in narrowing down to specific causes.
Step 5: Use the start
Command
If a bundle appears to have issues in the resolution state, you can try to start it manually using the following command:
start [bundle_id]
If the bundle cannot start, you'll typically see an output that details the problem, which can point you in the right direction for troubleshooting.
Step 6: Dependency Resolution Tools
There are numerous tools available that can ease the burden of dependency management within OSGi applications:
- Apache Felix: This lightweight OSGi framework allows you to examine bundle states, dependency trees, and other useful diagnostics.
- Bndtools: An IDE plugin that aids in managing OSGi bundles and their dependencies visually.
Example Code Snippet
Here is a simple example of how to use OSGi’s service registry in a bundle:
import org.osgi.framework.BundleActivator;
import org.osgi.framework.BundleContext;
import org.osgi.framework.ServiceRegistration;
public class Activator implements BundleActivator {
private ServiceRegistration<MyService> registration;
public void start(BundleContext context) {
MyService myService = new MyServiceImpl();
registration = context.registerService(MyService.class, myService, null);
System.out.println("MyService registered.");
}
public void stop(BundleContext context) {
registration.unregister();
System.out.println("MyService unregistered.");
}
}
Commentary
In the above code, start
method begins by creating an instance of MyServiceImpl
and registering it as a service. If there's a failure to register, it could indicate that MyService
is not seen by the OSGi framework, which could also point back to the MANIFEST.MF
for misconfiguration.
Lessons Learned
Troubleshooting OSGi bundle loading issues can be daunting, but understanding the lifecycle and dependencies is key. By leveraging the OSGi console, examining the MANIFEST.MF
, and using the right tools, you can efficiently identify and resolve common problems.
For further reading on OSGi, consider familiarizing yourself with the OSGi Alliance and looking into additional documentation from frameworks like Apache Felix.
Embrace the power of OSGi, and ensure your bundles load smoothly for an optimal application experience. Happy coding!