Boost JAX-RS and Spring Boot: Conquer Extreme Laziness!
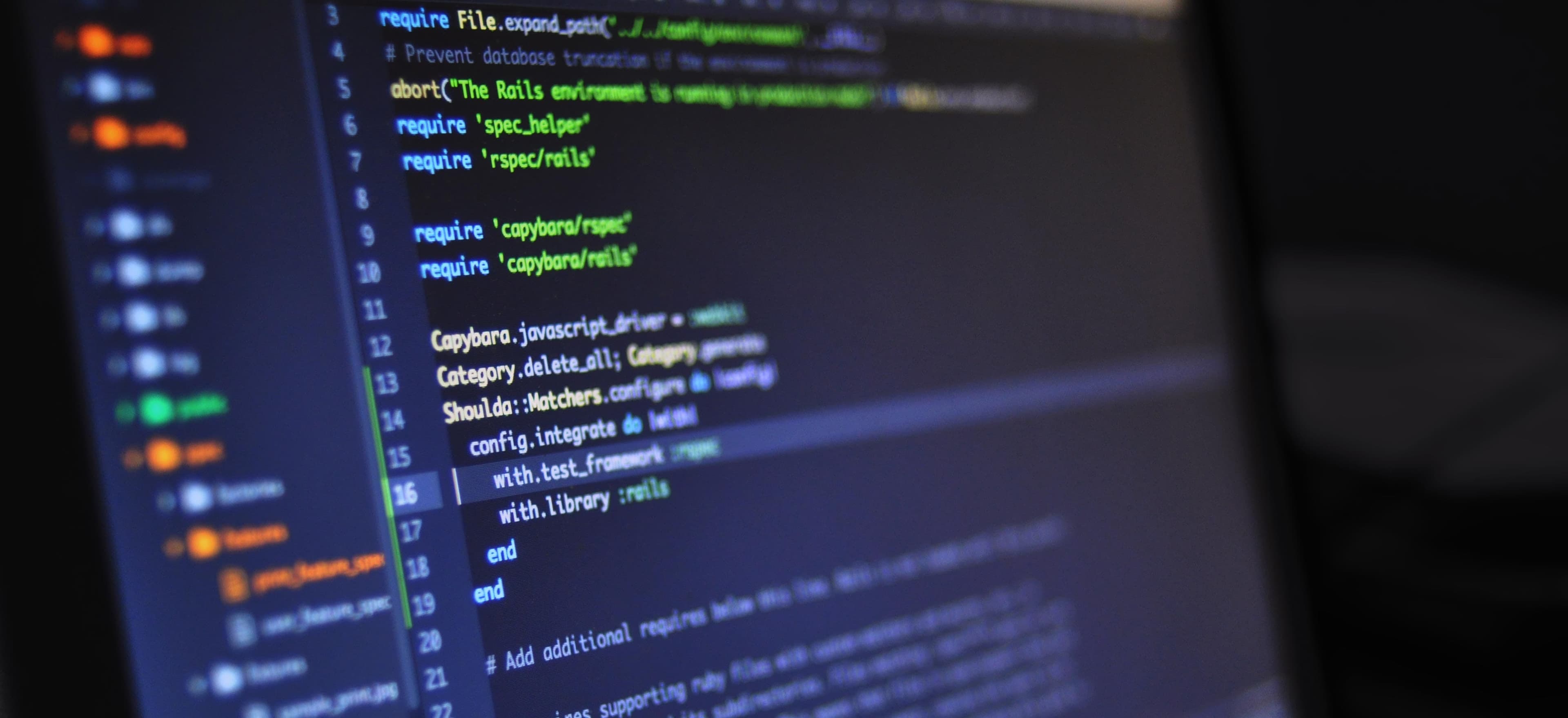
- Published on
Boost JAX-RS and Spring Boot: Conquer Extreme Laziness!
A Quick Look
In the world of Java development, building RESTful APIs has become a fundamental aspect of creating modern and scalable applications. Two technologies that have become increasingly popular for this purpose are JAX-RS and Spring Boot. JAX-RS, the Java API for RESTful Web Services, provides a powerful and versatile framework for building RESTful web services in Java. On the other hand, Spring Boot has emerged as a modern and efficient framework for developing microservices and standalone applications in the Java ecosystem.
Combining these two technologies, JAX-RS and Spring Boot, can provide developers with a comprehensive toolset for building robust, scalable, and maintainable RESTful APIs. In this article, we will explore the significance of using JAX-RS with Spring Boot, starting with an understanding of the individual technologies and then diving into the integration and practical application of these powerful tools.
What is JAX-RS?
JAX-RS, which stands for Java API for RESTful Web Services, is a set of APIs that provides support for building RESTful web services in Java. It is a key component of the Java EE (Enterprise Edition) ecosystem and is designed to simplify the development of web services that follow the Representational State Transfer (REST) architectural style.
Definition and Purpose of JAX-RS
At its core, JAX-RS provides a set of annotations and APIs that allow developers to build RESTful web services in Java. By adhering to the principles of REST, JAX-RS enables the creation of lightweight, scalable, and maintainable web services that can be accessed over the internet.
Brief History and Evolution of JAX-RS
JAX-RS was first introduced as part of the Java Community Process through JSR 311, which aimed to standardize the way web services are built in Java. Over time, JAX-RS has evolved and matured, with the latest version being JAX-RS 2.1, offering enhancements and additional features to support modern RESTful API development in Java.
Key Features of JAX-RS
- Annotations: JAX-RS provides a rich set of annotations that can be used to define resources, define HTTP methods, inject parameters, and handle request and response entities.
- Client API: In addition to server-side capabilities, JAX-RS also provides a client API that allows Java applications to consume RESTful services, making it a comprehensive solution for both building and consuming RESTful APIs.
Spring Boot: The Modern Java Framework
Spring Boot has gained immense popularity as a lightweight and opinionated framework for building stand-alone, production-grade Spring-based applications. It simplifies the process of creating and deploying microservices, making it an attractive choice for developers looking to build scalable and efficient applications with minimal configuration.
Quick Introduction to Spring Boot
Spring Boot takes the Spring framework to the next level by providing an opinionated approach to configuration, allowing developers to create standalone Spring applications with minimal fuss. It offers a range of features, including auto-configuration, which eliminates the need for manual setup, and an embedded servlet container, which allows applications to run with the bundled Tomcat, Jetty, or Undertow servers.
Advantage in Building Microservices
One of the key strengths of Spring Boot is its ability to streamline the development of microservices. With built-in support for creating RESTful web services, along with features like metrics, health checks, and externalized configuration, Spring Boot provides a robust foundation for developing microservices that can be deployed and scaled with ease.
Combining Forces: JAX-RS with Spring Boot
Integrating JAX-RS with Spring Boot allows developers to leverage the strengths of both technologies, resulting in a powerful and flexible platform for building RESTful APIs in Java. By combining JAX-RS's capabilities for defining RESTful endpoints and handling HTTP requests with Spring Boot's ease of development and deployment, developers can achieve a seamless and efficient workflow for creating web services.
How JAX-RS Complements Spring Boot's REST Capabilities
JAX-RS brings a wealth of features to the table, including resource mapping, request handling, and response generation, all of which are essential for building RESTful APIs. By integrating JAX-RS with Spring Boot, developers can take advantage of these features within the Spring ecosystem, benefiting from the ease of configuration and dependency management provided by Spring Boot.
Configuration and Setup for Using JAX-RS with Spring Boot
To integrate JAX-RS into a Spring Boot application, developers can simply include the appropriate dependencies and annotate their resource classes with JAX-RS annotations. This seamless integration allows for the creation of RESTful APIs with minimal ceremony, leveraging the strengths of both technologies without unnecessary complexity.
Pros and Cons of this Approach
Integrating JAX-RS with Spring Boot offers several advantages, including the ability to leverage the rich feature set of JAX-RS within the Spring Boot ecosystem, simplified configuration, and enhanced flexibility in designing RESTful APIs. However, it's important to note that this approach may introduce a slight learning curve for developers who are new to either JAX-RS or Spring Boot, and care should be taken to ensure that the integration is done in a way that aligns with best practices and performance considerations.
Exemplary Code Snippet
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import org.springframework.stereotype.Component;
@Component
@Path("/hello")
public class HelloResource {
@GET
public String sayHello() {
return "Hello, World!";
}
}
In this example, a simple JAX-RS resource class is annotated with both JAX-RS and Spring annotations, showcasing the integration of JAX-RS with Spring Boot.
Instructions
To configure a simple project setup that uses JAX-RS inside a Spring Boot application for creating a RESTful service, follow these steps:
- Create a new Spring Boot project using your preferred build tool (Maven/Gradle).
- Add the necessary dependencies for JAX-RS and Spring Boot in your project configuration file (pom.xml for Maven or build.gradle for Gradle).
- Define a JAX-RS resource class and annotate it with JAX-RS annotations, along with Spring annotations to manage it as a Spring component.
- Run the Spring Boot application, and you can access the RESTful endpoint defined in your JAX-RS resource.
Why?
By integrating JAX-RS with Spring Boot, developers can benefit from a harmonious combination of powerful RESTful capabilities and the streamlined development experience offered by Spring Boot, resulting in enhanced flexibility and control over the design and implementation of RESTful services.
Building a RESTful API: A Step-by-Step Guide
Now that we understand the significance of combining JAX-RS with Spring Boot, let's dive into a comprehensive tutorial on creating a RESTful API using these technologies.
Project Setup
To get started, let's create a new Spring Boot project with the necessary dependencies for JAX-RS. You can use Maven or Gradle for this purpose.
Maven Configuration
Add the following dependencies to your pom.xml
for Maven:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jersey</artifactId>
</dependency>
</dependencies>
Gradle Configuration
For Gradle, include the following dependencies in your build.gradle
file:
dependencies {
implementation 'org.springframework.boot:spring-boot-starter-web'
implementation 'org.springframework.boot:spring-boot-starter-jersey'
}
Defining a Model and Repository
In our example, we'll create a simple data model and a corresponding repository interface to manage the persistence of our model.
public class Book {
private Long id;
private String title;
// Getters and setters
}
public interface BookRepository {
List<Book> findAll();
Book findById(Long id);
void save(Book book);
void delete(Book book);
}
Creating RESTful Endpoints with JAX-RS
Next, let's define our RESTful endpoints for managing books. We'll create resources for retrieving all books, retrieving a specific book by ID, adding a new book, updating an existing book, and deleting a book.
@Path("/books")
public class BookResource {
private BookRepository repository;
@GET
public List<Book> getAllBooks() {
return repository.findAll();
}
@GET
@Path("/{id}")
public Book getBook(@PathParam("id") Long id) {
return repository.findById(id);
}
@POST
public Response addBook(Book book) {
repository.save(book);
return Response.status(Response.Status.CREATED).build();
}
@PUT
@Path("/{id}")
public Response updateBook(@PathParam("id") Long id, Book book) {
Book existingBook = repository.findById(id);
if (existingBook != null) {
repository.save(book);
return Response.status(Response.Status.OK).build();
} else {
return Response.status(Response.Status.NOT_FOUND).build();
}
}
@DELETE
@Path("/{id}")
public Response deleteBook(@PathParam("id") Long id) {
Book book = repository.findById(id);
if (book != null) {
repository.delete(book);
return Response.status(Response.Status.OK).build();
} else {
return Response.status(Response.Status.NOT_FOUND).build();
}
}
}
Service Layer
To encapsulate the business logic related to managing books, we can create a service class that interacts with the repository and provides additional functionality if needed.
@Service
public class BookService {
private BookRepository repository;
public List<Book> getAllBooks() {
return repository.findAll();
}
public Book getBook(Long id) {
return repository.findById(id);
}
public void addBook(Book book) {
repository.save(book);
}
public void updateBook(Long id, Book book) {
Book existingBook = repository.findById(id);
if (existingBook != null) {
repository.save(book);
} else {
throw new NotFoundException("Book not found");
}
}
public void deleteBook(Long id) {
Book book = repository.findById(id);
if (book != null) {
repository.delete(book);
} else {
throw new NotFoundException("Book not found");
}
}
}
Testing Your API
Once our RESTful API is in place, we can use tools like Postman to test the endpoints and ensure that they behave as expected. Send various HTTP requests (GET, POST, PUT, DELETE) to interact with the API and verify the responses.
Real-World Applications
Integrating JAX-RS with Spring Boot has proven to be beneficial in a wide range of real-world applications, from e-commerce platforms to social media backends. The combination of these technologies offers scalable, maintainable, and efficient solutions for building RESTful APIs in Java.
One prominent example of real-world application is a social media analytics platform that utilizes JAX-RS with Spring Boot to handle an extensive network of data endpoints and provide real-time analytics to its users. By leveraging the strengths of both technologies, the platform achieves high levels of scalability, ease of development, and performance improvements in processing and analyzing large volumes of social media data.
Best Practices for Using JAX-RS with Spring Boot
To ensure the effective and efficient utilization of JAX-RS with Spring Boot for building RESTful services, it's important to follow best practices in various aspects of development.
Code Organization
Organize your JAX-RS resources, service classes, and repository implementations in a structured manner that aligns with the principles of modularity and separation of concerns. Group related functionality into appropriate packages and classes to maintain a clear and maintainable codebase.
Exception Handling
Implement robust exception handling strategies to gracefully handle errors and exceptional conditions that may occur during the execution of your RESTful services. Utilize the appropriate exception types and status codes to convey meaningful error messages to clients consuming your APIs.
Validation
Incorporate validation mechanisms to ensure that incoming requests adhere to the expected formats and constraints. Leverage validation annotations or custom validation logic to validate and sanitize input data before processing it within your API endpoints.
Documentation (Swagger or OpenAPI)
Leverage tools like Swagger or OpenAPI to generate comprehensive documentation for your RESTful APIs. By documenting your endpoints, request and response structures, and supported operations, you can provide valuable insights to consumers and facilitate seamless integration with client applications.
Bringing It All Together
In this article, we've explored the integration of JAX-RS with Spring Boot, highlighting the significance and benefits of combining these technologies to develop RESTful APIs in Java. By harnessing the capabilities of JAX-RS for defining RESTful endpoints and leveraging the streamlined development experience offered by Spring Boot, developers can create scalable, maintainable, and performant web services.
As you embark on your journey to build RESTful APIs, we encourage you to experiment with JAX-RS and Spring Boot in your projects. The harmonious synergy between these technologies offers an exciting opportunity to elevate your API development endeavors. For further exploration, we recommend delving into the official documentation for JAX-RS and Spring Boot, along with engaging in discussions and learning from the community through resources like forums and educational platforms.
With an informed approach and a spirit of exploration, you can conquer extreme laziness and boost your API development with the powerful combination of JAX-RS and Spring Boot.
Happy Coding!