Unlocking EntityManager: Boost Performance in Spring Data JPA
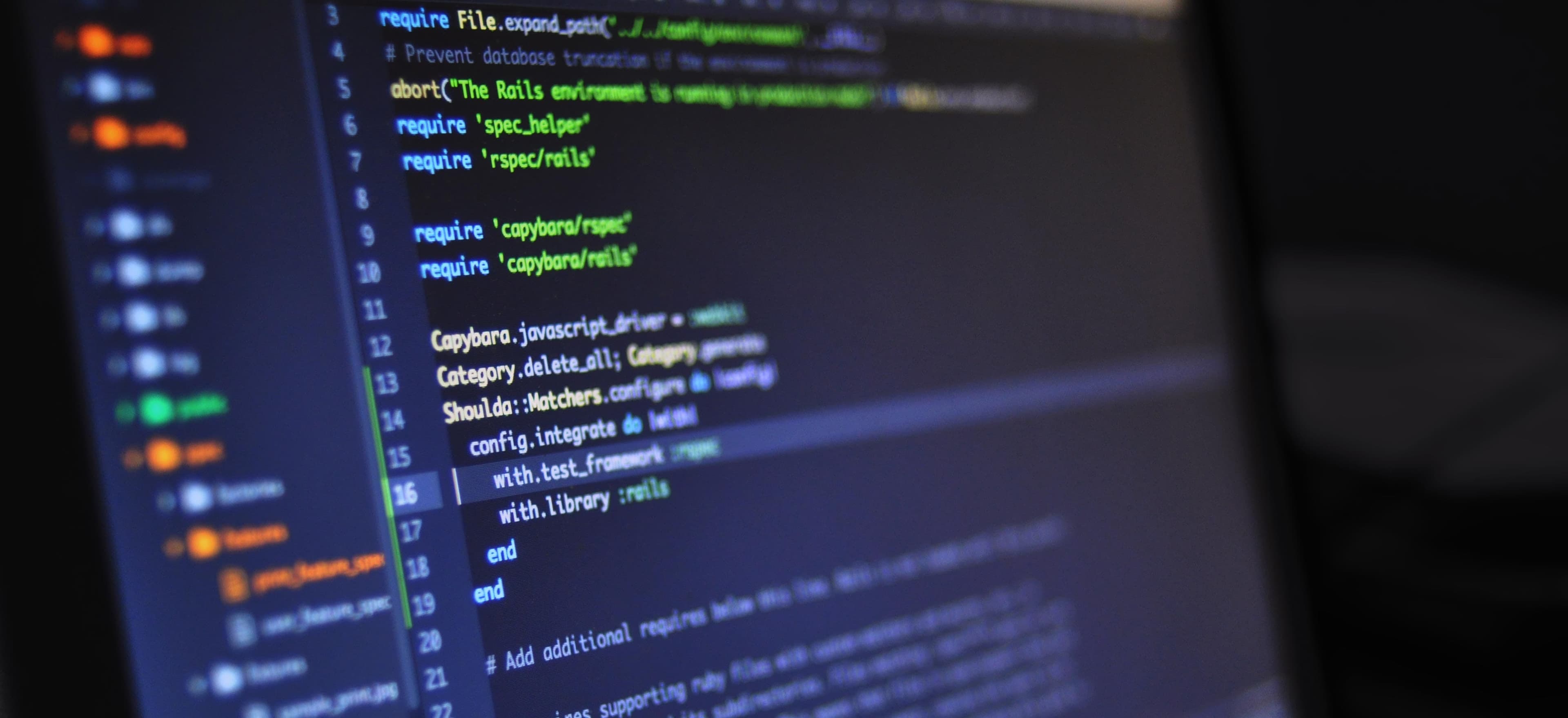
- Published on
Unlocking EntityManager: Boost Performance in Spring Data JPA
When working with Spring Data JPA, one of the key components is the EntityManager. Understanding how to properly utilize and optimize the EntityManager can significantly impact the performance of your application. In this article, we will dive into the best practices for unlocking the potential of the EntityManager to boost overall performance in your Java application.
What is EntityManager?
In the context of Spring Data JPA, EntityManager serves as the interface for interacting with the database. It is responsible for managing the lifecycle of entities, performing CRUD operations, and executing queries. The EntityManager acts as a bridge between the Java application and the underlying database, providing a set of powerful methods for data manipulation and retrieval.
Utilizing EntityManager for Performance Optimization
1. Batch Operations
When dealing with a large number of entities, leveraging batch operations can significantly enhance performance. By utilizing the EntityManager's persist()
and flush()
methods within a transaction, you can efficiently process and persist a batch of entities in a single database round-trip.
@Transactional
public void saveAllEntities(List<Entity> entities) {
int batchSize = 20;
for (int i = 0; i < entities.size(); i++) {
entityManager.persist(entities.get(i));
if (i % batchSize == 0 && i > 0) {
entityManager.flush();
entityManager.clear();
}
}
entityManager.flush();
entityManager.clear();
}
Why: This approach optimizes database writes by minimizing the number of round-trips, thereby enhancing overall performance.
2. Fetching Strategies
The EntityManager allows you to define fetch strategies for entity associations, such as lazy and eager fetching. Carefully selecting the appropriate fetch strategy based on the specific use case can prevent redundant queries and optimize data retrieval.
@OneToMany(fetch = FetchType.LAZY)
private List<ChildEntity> children;
Why: Utilizing lazy fetching for collections that are not immediately required can prevent unnecessary data retrieval, thus improving performance.
3. Explicit Query Tuning
Spring Data JPA provides the flexibility to execute custom queries through the EntityManager. By crafting optimized JPQL or native SQL queries, you can precisely tailor the data retrieval process to align with the performance requirements of your application.
TypedQuery<Entity> query = entityManager.createQuery("SELECT e FROM Entity e WHERE e.status = :status", Entity.class);
query.setParameter("status", "ACTIVE");
List<Entity> resultList = query.getResultList();
Why: Crafting explicit queries enables you to retrieve only the required data, resulting in improved performance and reduced overhead.
4. Second-level Caching
By enabling second-level caching in the EntityManager, you can cache entity data at the EntityManagerFactory level, thereby reducing the number of database hits for commonly accessed entities.
@Entity
@Cacheable
public class Entity {
// Entity mapping
}
Why: Utilizing second-level caching can lead to a significant performance boost by minimizing database interactions for frequently accessed data.
5. EntityManager Lifecycle Management
Proper management of the EntityManager's lifecycle is crucial for performance optimization. Ensuring that the EntityManager is utilized within the appropriate scope and closed when no longer needed helps to conserve resources and prevent memory leaks.
@PersistenceContext
private EntityManager entityManager;
Why: Managing the lifecycle of the EntityManager effectively ensures efficient resource utilization and prevents potential performance bottlenecks.
Closing Remarks
Optimizing the performance of your Spring Data JPA application with the EntityManager is essential for achieving scalability and efficiency. By embracing best practices such as batch operations, fetch strategies, query tuning, caching, and lifecycle management, you can unleash the full potential of the EntityManager and elevate the overall performance of your Java application.
Unlock the power of EntityManager, and witness the transformation in performance that it brings to your Spring Data JPA application.
With these optimization tips and best practices, you can drive performance gains in your Spring Data JPA applications. Be sure to implement these strategies thoughtfully and measure the impact to fine-tune your application for optimal efficiency. Do follow us for more insightful articles on Java development and performance optimization.