Why Your Code Loops Infinitely: Debugging 101
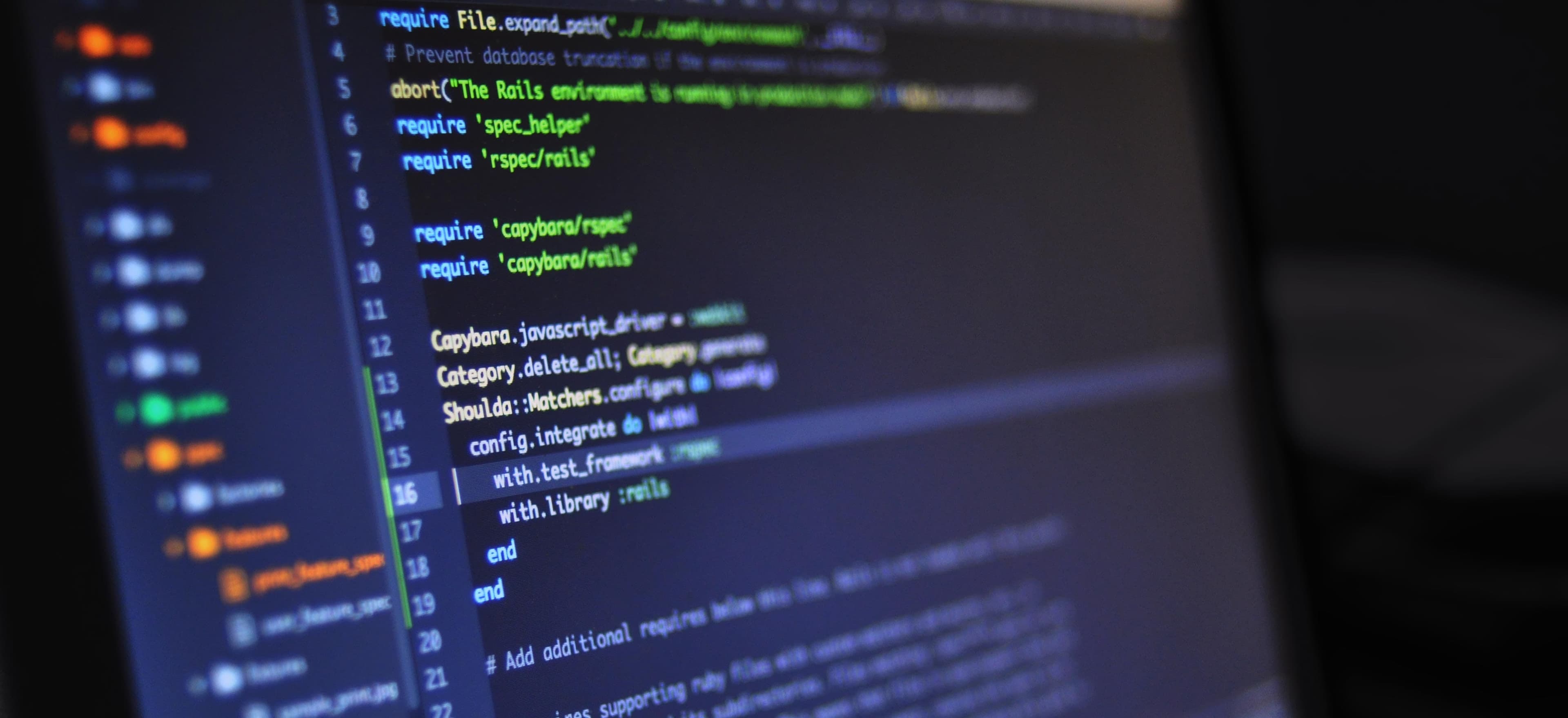
- Published on
Why Your Code Loops Infinitely: Debugging 101
As a Java developer, encountering an infinite loop in your code can be frustrating and time-consuming to resolve. Infinite loops occur when a condition in a loop never evaluates to false, causing the loop to repeat indefinitely. In this blog post, we will explore common reasons for infinite loops in Java code and how to effectively debug and resolve them.
Understanding Infinite Loops
Infinite loops can manifest in various forms, such as while
loops, for
loops, or recursive functions that fail to reach a base case. These loops can consume system resources and lead to unresponsive or crashing applications if not addressed promptly.
Let’s start by examining a simple example of an infinite loop:
public class InfiniteLoopExample {
public static void main(String[] args) {
int i = 0;
while (i < 5) {
System.out.println(i);
// Missing increment statement
}
}
}
In this example, the i
variable is never incremented within the while
loop, causing the loop to continue indefinitely since the condition i < 5
never becomes false.
Reasons for Infinite Loops
1. Missing or Incorrect Condition
One of the most common reasons for an infinite loop is a missing or incorrect condition within the loop statement. This could be an oversight during the coding process or a logic error that prevents the loop from terminating as expected.
Let’s consider the following example:
public class InfiniteLoopExample {
public static void main(String[] args) {
int i = 0;
while (i > 0) {
System.out.println(i);
i--; // Incorrect decrement statement
}
}
}
In this case, the loop condition i > 0
should have been i >= 0
to ensure that the loop terminates when i
reaches 0. The incorrect condition leads to an infinite loop as i
never becomes less than or equal to 0.
2. Unintended Side Effects
Unintended side effects within the loop body can also trigger infinite loops. These side effects may alter the loop control variables in unexpected ways, preventing the loop from reaching its exit condition.
Consider the following code snippet:
public class InfiniteLoopExample {
public static void main(String[] args) {
int i = 0;
while (i < 5) {
System.out.println(i);
i = i--;
}
}
}
In this example, the expression i = i--;
introduces an unintended side effect. The post-decrement operation sets i
to its current value and then decrements it, effectively maintaining i
at its initial value and causing the loop to iterate infinitely.
Debugging Infinite Loops
1. Review Loop Conditions and Control Variables
When faced with an infinite loop, start by reviewing the loop conditions and the manipulation of control variables within the loop. Ensure that the conditions are appropriate and that the control variables are being modified in a way that allows the loop to progress towards its termination.
public class InfiniteLoopExample {
public static void main(String[] args) {
int i = 0;
while (i < 5) {
System.out.println(i);
i++; // Correct increment statement
}
}
}
Adding the correct increment statement (i++
) resolves the infinite loop, allowing the loop to terminate once i
reaches 5.
2. Use Debugging Tools
Utilize debugging tools provided by Integrated Development Environments (IDEs) such as IntelliJ IDEA, Eclipse, or NetBeans. These tools allow you to set breakpoints, inspect variable values, and step through the code to identify the specific point at which the loop enters the infinite state.
Key Takeaways
In conclusion, infinite loops can arise from various issues such as missing or incorrect conditions, unintended side effects, or logical errors. When encountering an infinite loop in your Java code, carefully review the loop conditions and control variable manipulation. Additionally, leverage debugging tools to pinpoint the root cause of the infinite loop and track the flow of your code effectively. By understanding and addressing the common reasons for infinite loops, you can enhance the robustness and reliability of your Java applications.
Debugging infinite loops is a fundamental skill for Java developers and mastering it will contribute to more efficient and bug-free code. Happy coding!
Remember, a keen eye for detail and the use of effective debugging techniques are essential in identifying and resolving infinite loops in your Java code. This practice will not only enhance the quality of your code but also sharpen your problem-solving skills as a developer.
To deepen your understanding of debugging in Java, check out this article on Java Debugging Techniques. Additionally, exploring the concept of Loop Optimization in Java can provide insights into streamlining your loops for improved performance.
Checkout our other articles