Unlock Java Mastery: 20 Must-Know Libraries & APIs for 2023
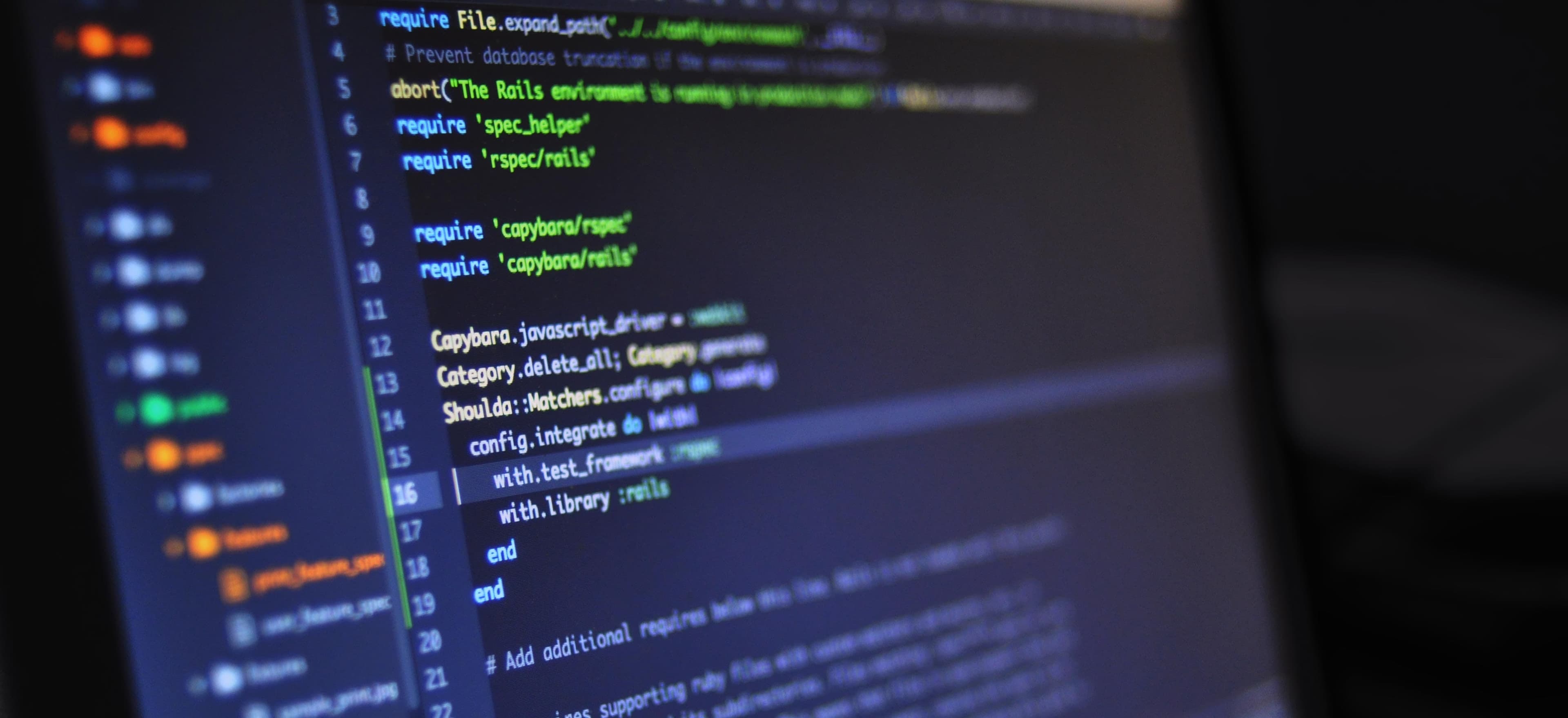
- Published on
Unlock Java Mastery: 20 Must-Know Libraries & APIs for 2023
Java, a powerhouse in the world of programming, continues to be pivotal for software development across diverse industries. Whether you're developing enterprise-level applications, dynamic web applications, or embarking on new-age technologies like IoT and big data, Java provides a robust platform for building scalable and performant solutions. In 2023, it's more crucial than ever to stay updated with the essential libraries and APIs that can significantly enhance your Java projects. This comprehensive guide introduces you to 20 must-know Java libraries and APIs, promising to elevate your code functionality and streamline your development process.
1. Spring Framework
The Spring Framework remains a titan in the Java ecosystem, offering comprehensive infrastructure support for developing Java applications. At its core, Spring simplifies the development of complex enterprise applications, with functionalities for dependency injection, aspect-oriented programming, and more. It's a must for developers aiming to build high-performance, testable, and reusable code.
@Autowired
private UserRepository userRepository;
public void saveUser(User user) {
userRepository.save(user);
}
In this snippet, @Autowired
elegantly handles dependency injection, showcasing Spring's simplicity and power.
Explore more: Spring Framework
2. Apache Kafka
As the digital world increasingly shifts towards real-time data processing, Apache Kafka has emerged as a go-to platform for building robust data pipelines and streaming applications. Its distributed architecture ensures high throughput, fault tolerance, and scalability, critical for processing large streams of data efficiently.
ProducerRecord<String, String> record = new ProducerRecord<>("Topic", "Hello, Kafka!");
producer.send(record);
Here, we're creating a simple producer that sends a message to a Kafka topic, illustrating Kafka's straightforward approach to real-time data streaming.
Dive deeper: Apache Kafka
3. Jackson
In the realm of web services and APIs, data interchange formats such as JSON are indispensable. Jackson, a high-performance JSON processor, enables easy serialization and deserialization of Java objects, facilitating smooth data exchange between Java applications and RESTful APIs.
ObjectMapper mapper = new ObjectMapper();
String json = mapper.writeValueAsString(myObject);
This code converts a Java object to a JSON string, showcasing Jackson's efficiency in handling JSON data.
4. JUnit
Testing is a critical phase of software development, and JUnit stands as the framework of choice for implementing unit tests in Java. It promotes the idea of "test early, test often," helping developers catch and fix bugs early in the development lifecycle.
@Test
public void shouldAnswerWithTrue() {
assertTrue(true);
}
The simplicity of declaring a test with @Test
and the readability of assertions like assertTrue
make JUnit essential for quality assurance.
5. Apache Maven
Maven is more than just a build tool; it's a comprehensive project management and comprehension tool that simplifies the process of building and managing Java projects. It automates the process of project builds, dependencies, documentation, and more, using an XML file (pom.xml) for configuration.
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>5.3.10</version>
</dependency>
This pom.xml
snippet demonstrates how dependencies are declared, making it straightforward to manage and update them.
6. Apache Camel
Integration is a common and complex problem in enterprise application development. Apache Camel is a powerful open-source integration framework that simplifies the integration of disparate systems and APIs using various patterns.
from("direct:start").to("log:end");
With just one line, we define a route from a start point to an end, underscoring Camel's ability to make integration flow as smooth as possible.
7. Google Guava
Guava enriches the Java ecosystem with a suite of core libraries that provide utility functions for collections, caching, primitives support, concurrency, and more. It's particularly useful for filling in gaps in the Java standard library.
List<String> names = Lists.newArrayList("Alice", "Bob", "Charlie");
By using Guava’s Lists.newArrayList
, creating and initializing a list becomes cleaner and more concise.
8. Hibernate
Hibernate ORM (Object-Relational Mapping) offers a framework for mapping an object-oriented domain model to a relational database. It deals with data persistence logically, abstracting away the complexities of database interactions.
Session session = sessionFactory.openSession();
session.beginTransaction();
session.save(new Event("Our very first event!"));
session.getTransaction().commit();
session.close();
This code snippet succinctly demonstrates the process of creating a new database entry, illustrating Hibernate's effectiveness in ORM.
9. Log4j
Logging is an indispensable part of debugging and monitoring Java applications. Log4j provides a reliable logging framework that is flexible and configurable, making it a go-to choice for developers.
Logger logger = LogManager.getLogger(MyClass.class);
logger.info("This is an info log entry");
Simple yet powerful, this example shows how to log an information message, crucial for tracking application behavior.
10. Mockito
In unit testing, mocking objects is a common requirement for isolating test environments. Mockito is a popular mocking framework that simplifies the creation of mock objects in automated unit tests, allowing for more precise and focused testing.
List mockedList = mock(List.class);
when(mockedList.get(0)).thenReturn("first");
assertEquals("first", mockedList.get(0));
Mockito's ease of creating and manipulating mock objects is evident, making unit tests more reliable.
11. REST-assured
Testing REST APIs is critical in ensuring the smooth functioning of web applications. REST-assured provides a domain-specific language to simplify testing REST services, bringing simplicity and elegance to API tests.
given().when().get("/events").then().statusCode(200);
This fluent API for testing HTTP endpoints highlights REST-assured's capacity for clear and concise API testing.
12. Quartz Scheduler
In applications that require scheduling jobs to run at certain times, Quartz Scheduler offers a flexible and robust solution. It allows for the scheduling of jobs that execute your Java code based on various criteria.
JobDetail job = newJob(MyJob.class)
.withIdentity("myJob", "group1")
.build();
Quartz’s approach to defining jobs is not only simple but highly configurable, addressing a wide array of scheduling needs.
13. JavaMail API
Sending emails programmatically is a common requirement for Java applications. The JavaMail API provides a platform-independent and protocol-independent framework to build mail and messaging applications.
Message message = new MimeMessage(session);
message.setFrom(new InternetAddress("from@email.com"));
message.setRecipients(
Message.RecipientType.TO,
InternetAddress.parse("to@email.com")
);
message.setSubject("Test Mail");
message.setText("This is a test mail from JavaMail API");
This snippet illustrates sending a simple email, highlighting the JavaMail API's ease of use.
14. Java Persistence API (JPA)
For managing relational data in applications developed in the Java platform, JPA offers a specification for ORM functionalities. It simplifies data persistence in applications, reducing boilerplate code and improving application performance.
15. Lombok
Boilerplate code is a known pain point in Java. Lombok provides annotations to eliminate this, automatically generating getters, setters, constructors, and more.
@Getter @Setter
private String name;
Lombok's annotations like @Getter
and @Setter
drastically reduce the verbosity of Java code, enhancing readability and maintainability.
16. Google TensorFlow for Java
In the burgeoning field of AI and machine learning, Google TensorFlow offers Java APIs for employing machine learning models in Java applications, tapping into powerful computational libraries for data flow programming.
17. Elasticsearch Java API
For applications requiring search capabilities, Elasticsearch offers a distributed, RESTful search and analytics engine. The Elasticsearch Java API allows for real-time data indexing and search functionality, powering complex search experiences.
18. Vaadin
Developing web UIs in Java is made simpler with Vaadin, a web framework that allows developers to build secure, high-quality web apps with a rich set of components and a responsive design.
19. JHipster
For rapid application development, JHipster offers a development platform to generate, develop, and deploy Spring Boot + Angular/React applications and Spring microservices.
20. Vert.x
Vert.x is a tool-kit for building reactive applications on the JVM. It provides a simple and responsive programming model, supporting a broad range of languages including Java.
In the dynamic and expansive world of Java development, staying abreast of the latest libraries and APIs is paramount. These 20 must-know libraries and APIs for 2023 equip you with the tools and frameworks to create efficient, robust, and scalable Java applications. The power of Java combined with these libraries and APIs provides a versatile foundation for solving a myriad of software development challenges, ushering in a new era of innovation and efficiency in the Java ecosystem.
Remember, the journey to mastering Java is ongoing and evolving. Continuously exploring, learning, and integrating these libraries and APIs into your projects will not only sharpen your Java skills but also significantly enhance the quality and performance of your applications. Explore, experiment, and excel in your Java development endeavors!
Checkout our other articles