Overcoming XML Challenges in RESTful API Inbound Adapters
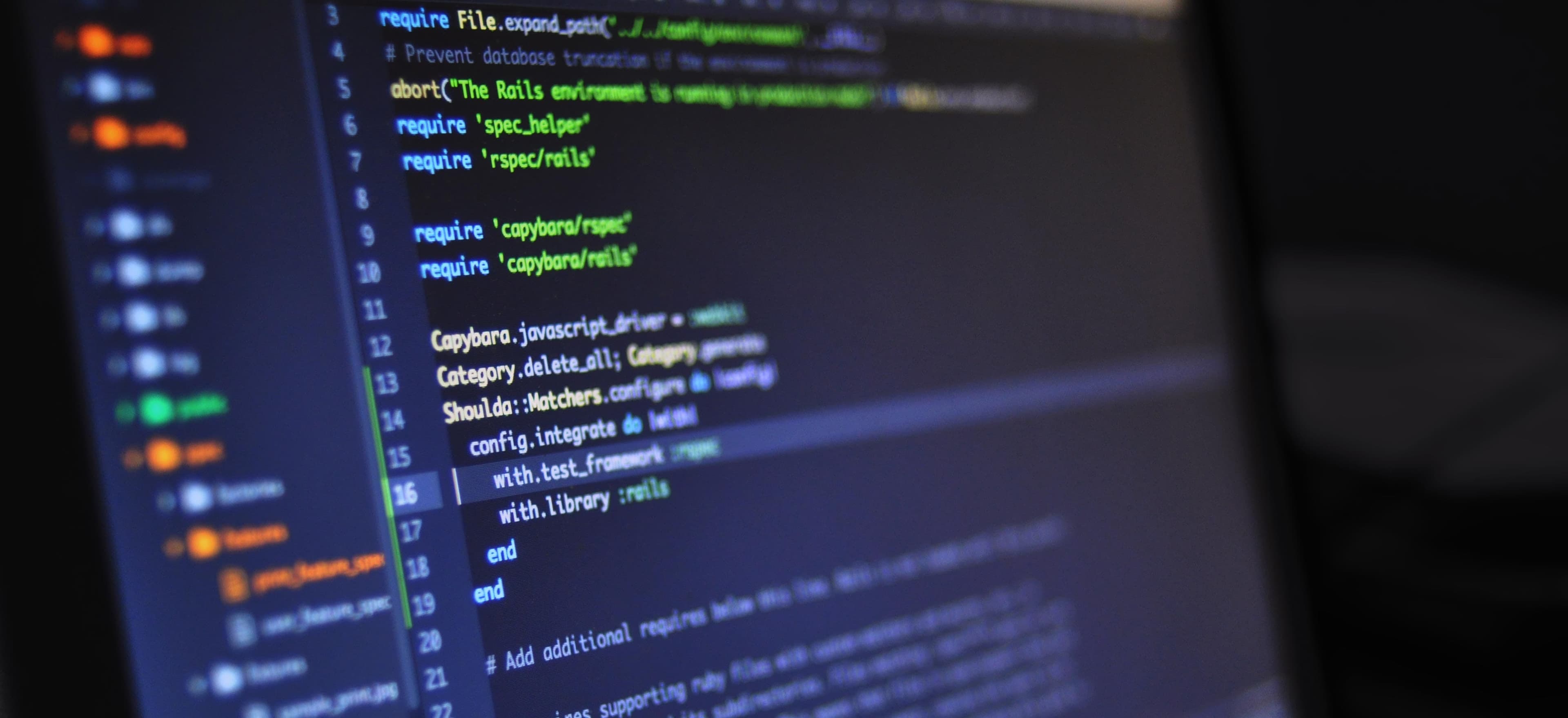
- Published on
Overcoming XML Challenges in RESTful API Inbound Adapters
RESTful APIs have become the standard for building and consuming web services due to their simplicity and flexibility. However, when dealing with XML data in RESTful API inbound adapters, developers often encounter various challenges. In this article, we'll explore these challenges and discuss effective strategies for overcoming them using Java.
The Challenge of Parsing XML Data
One of the primary challenges when working with XML data in RESTful API inbound adapters is parsing the incoming XML payload. XML, being a verbose and complex format, requires a robust parser that can efficiently extract relevant information while handling various edge cases.
Using JAXB for XML Binding
In Java, one of the most popular approaches for parsing XML data is using JAXB (Java Architecture for XML Binding). JAXB provides a convenient way to map XML schemas to Java objects, allowing for seamless conversion between XML and Java objects.
Let's take a look at an example of how JAXB can be used to parse XML data in a RESTful API inbound adapter:
import javax.xml.bind.JAXBContext;
import javax.xml.bind.JAXBException;
import javax.xml.bind.Unmarshaller;
import java.io.StringReader;
public class XmlParser {
public static <T> T unmarshal(String xml, Class<T> type) throws JAXBException {
JAXBContext jaxbContext = JAXBContext.newInstance(type);
Unmarshaller jaxbUnmarshaller = jaxbContext.createUnmarshaller();
return type.cast(jaxbUnmarshaller.unmarshal(new StringReader(xml)));
}
}
In this example, we define a XmlParser
class with a method that uses JAXB to unmarshal an XML string into a Java object of a given type. This allows the RESTful API inbound adapter to work with the parsed Java object directly, simplifying the data handling process.
Handling XML Validation
Another significant challenge when dealing with XML data in RESTful API inbound adapters is ensuring the incoming XML payload adheres to a specific schema or structure. Validating XML data is crucial for maintaining data integrity and preventing unexpected errors further down the processing pipeline.
Using XML Schema for Validation
To address this challenge, Java provides support for validating XML data against an XML schema using the javax.xml.validation package. By defining an XML schema and validating incoming XML data against it, developers can ensure that the data conforms to the expected structure.
Let's consider a code snippet that demonstrates XML validation using an XML schema:
import javax.xml.XMLConstants;
import javax.xml.transform.stream.StreamSource;
import javax.xml.validation.Schema;
import javax.xml.validation.SchemaFactory;
import javax.xml.validation.Validator;
import org.xml.sax.SAXException;
import java.io.IOException;
public class XmlValidator {
public static boolean validateXmlAgainstSchema(String xml, String schemaPath) {
try {
SchemaFactory factory = SchemaFactory.newInstance(XMLConstants.W3C_XML_SCHEMA_NS_URI);
Schema schema = factory.newSchema(new StreamSource(XmlValidator.class.getClassLoader().getResourceAsStream(schemaPath));
Validator validator = schema.newValidator();
validator.validate(new StreamSource(new StringReader(xml)));
return true;
} catch (SAXException | IOException e) {
// Handle validation errors
return false;
}
}
}
In this example, the XmlValidator
class contains a method that validates an XML string against a specified XML schema. If the validation fails, the method returns false, allowing the RESTful API inbound adapter to handle the validation errors gracefully.
Transforming XML Data
In certain scenarios, it may be necessary to transform the incoming XML data into a different structure before processing it in the RESTful API inbound adapter. XML transformation involves applying specific rules or mappings to convert the XML data into a format that aligns with the internal data model or processing requirements.
Leveraging XSLT for XML Transformation
Java offers support for XML transformation using XSLT (eXtensible Stylesheet Language Transformations), which provides a powerful mechanism for defining transformation rules and applying them to XML documents.
Let's examine a code snippet that showcases XML transformation using XSLT:
import javax.xml.transform.Transformer;
import javax.xml.transform.TransformerFactory;
import javax.xml.transform.stream.StreamResult;
import javax.xml.transform.stream.StreamSource;
import java.io.StringReader;
import java.io.StringWriter;
public class XmlTransformer {
public static String transformXml(String xml, String xslt) {
try {
TransformerFactory factory = TransformerFactory.newInstance();
Transformer transformer = factory.newTransformer(new StreamSource(new StringReader(xslt)));
StringWriter result = new StringWriter();
transformer.transform(new StreamSource(new StringReader(xml)), new StreamResult(result));
return result.toString();
} catch (Exception e) {
// Handle transformation errors
return null;
}
}
}
In this example, the XmlTransformer
class contains a method that applies an XSLT stylesheet to transform an XML string into a new structure. If any errors occur during the transformation process, they can be handled appropriately within the RESTful API inbound adapter.
To Wrap Things Up
In conclusion, working with XML data in RESTful API inbound adapters presents several challenges, including parsing, validation, and transformation. By leveraging Java's capabilities for XML processing, such as JAXB for parsing, XML schema validation, and XSLT for transformation, developers can effectively overcome these challenges and ensure robust handling of XML data within their RESTful API implementations.
By adopting best practices for XML processing and utilizing appropriate Java libraries, developers can streamline the handling of XML data in RESTful API inbound adapters, ultimately enhancing the reliability and efficiency of their API integrations.
In future blog posts, we will delve into advanced XML processing techniques and explore how to tackle additional complexities that may arise in RESTful API implementations.
Feel free to share your thoughts and experiences with XML processing in RESTful API inbound adapters in the comments below.
For further information on XML processing in Java, refer to the official documentation: Java XML API
Checkout our other articles