Why Hystrix is Fading: Navigating Spring Cloud's Shift
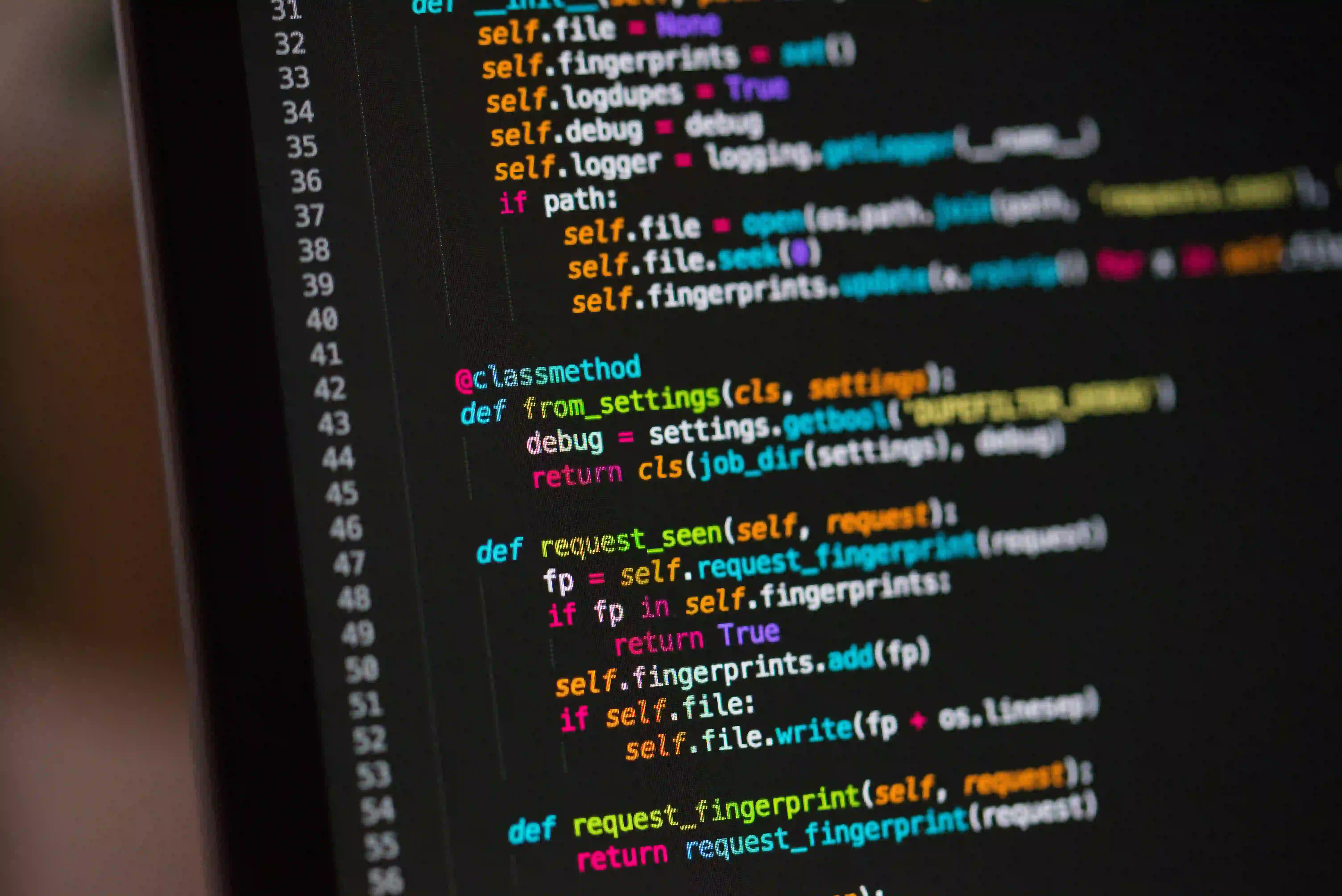
Why Hystrix is Fading: Navigating Spring Cloud's Shift
In the era of microservices, fault tolerance and resilience are critical aspects of a robust system. With the rise of Spring Cloud, developers have been provided with a suite of tools to address these challenges. One of the most popular solutions for managing faults in distributed systems was Netflix's Hystrix. However, as the landscape evolves, so do the best practices for dealing with these concerns. In this blog post, we will explore the decline of Hystrix and navigate through the shifting terrain of fault tolerance within Spring Cloud.
The Rise of Hystrix
Hystrix was a game-changer when it was introduced. It provided a solution for handling faults in distributed systems by isolating and controlling interactions between services. With its circuit breaking capabilities and fault tolerance features, Hystrix became the go-to library for many Spring developers. However, as the industry progressed, new patterns and technologies emerged, prompting a shift away from Hystrix.
The Decline of Hystrix
Several factors have contributed to the waning popularity of Hystrix. One of the primary reasons is the announcement by Netflix that they are no longer actively developing the library. This declaration signaled the beginning of the end for Hystrix, prompting the community to seek alternative solutions for fault tolerance.
Spring Cloud's Resilience4j
In response to the diminishing support for Hystrix, Spring Cloud has embraced Resilience4j as the recommended library for handling resilience and fault tolerance. Resilience4j provides a more lightweight and flexible approach to managing faults in distributed systems. With its focus on functional composition and ease of use, Resilience4j has gained traction as the successor to Hystrix.
Understanding Resilience4j
Let's delve into some code to understand why Resilience4j is gaining favor among developers. Below is an example of how to use Resilience4j's CircuitBreaker in a Spring Boot application.
import io.github.resilience4j.circuitbreaker.CircuitBreakerRegistry;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class Resilience4jConfig {
@Bean
public CircuitBreakerRegistry circuitBreakerRegistry() {
return CircuitBreakerRegistry.ofDefaults();
}
}
In this snippet, we are configuring a CircuitBreakerRegistry bean, which is a key component of Resilience4j. The registry provides a central place to manage CircuitBreaker instances, allowing for easy customization and configuration.
import io.github.resilience4j.circuitbreaker.CircuitBreaker;
import org.springframework.web.client.RestTemplate;
public class MyService {
private final CircuitBreaker circuitBreaker;
private final RestTemplate restTemplate;
public MyService(CircuitBreaker circuitBreaker, RestTemplate restTemplate) {
this.circuitBreaker = circuitBreaker;
this.restTemplate = restTemplate;
}
public String doSomething() {
return circuitBreaker.executeSupplier(() -> restTemplate.getForObject("https://api.example.com", String.class));
}
}
In this example, we have a MyService class that utilizes a CircuitBreaker to protect the call to an external service. The circuitBreaker.executeSupplier method wraps the restTemplater.getForObject call, providing fault tolerance and resilience.
As seen in these examples, Resilience4j offers a more concise and functional approach to managing fault tolerance compared to Hystrix. Its lightweight nature and alignment with modern functional programming paradigms have made it a compelling choice for Spring Cloud developers.
Embracing the Future with Spring Cloud Circuit Breaker
In addition to Resilience4j, Spring Cloud has introduced its own abstraction for circuit breakers, allowing for seamless integration with different underlying implementations, including Resilience4j. The Spring Cloud Circuit Breaker project provides a unified interface for working with various circuit breaker libraries, enabling developers to switch between implementations with ease.
import org.springframework.cloud.circuitbreaker.resilience4j.Resilience4JCircuitBreakerFactory;
import org.springframework.cloud.circuitbreaker.CircuitBreakerFactory;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class CircuitBreakerConfig {
@Bean
public CircuitBreakerFactory circuitBreakerFactory() {
return new Resilience4JCircuitBreakerFactory();
}
}
In this snippet, we are configuring a CircuitBreakerFactory bean using Resilience4j as the underlying implementation. This seamless integration demonstrates the flexibility and future-proofing that Spring Cloud Circuit Breaker brings to the table.
Key Takeaways
As the industry landscape continues to evolve, it's important for developers to stay abreast of the latest best practices and technologies. With the decline of Hystrix, Spring Cloud has adapted by embracing Resilience4j and introducing its own abstraction for circuit breakers. By leveraging these tools, developers can ensure the fault tolerance and resilience of their microservices, paving the way for robust and reliable distributed systems.
In conclusion, the shift away from Hystrix signals a new phase in the realm of fault tolerance within Spring Cloud. As we navigate this transition, embracing Resilience4j and Spring Cloud Circuit Breaker will enable us to build resilient and fault-tolerant systems that meet the demands of modern microservice architectures.
Remember, staying updated with the latest trends and tools is crucial for staying competitive in the ever-changing world of software development.
References: