Boost Your Trading Game: Simplifying Systems with Akka
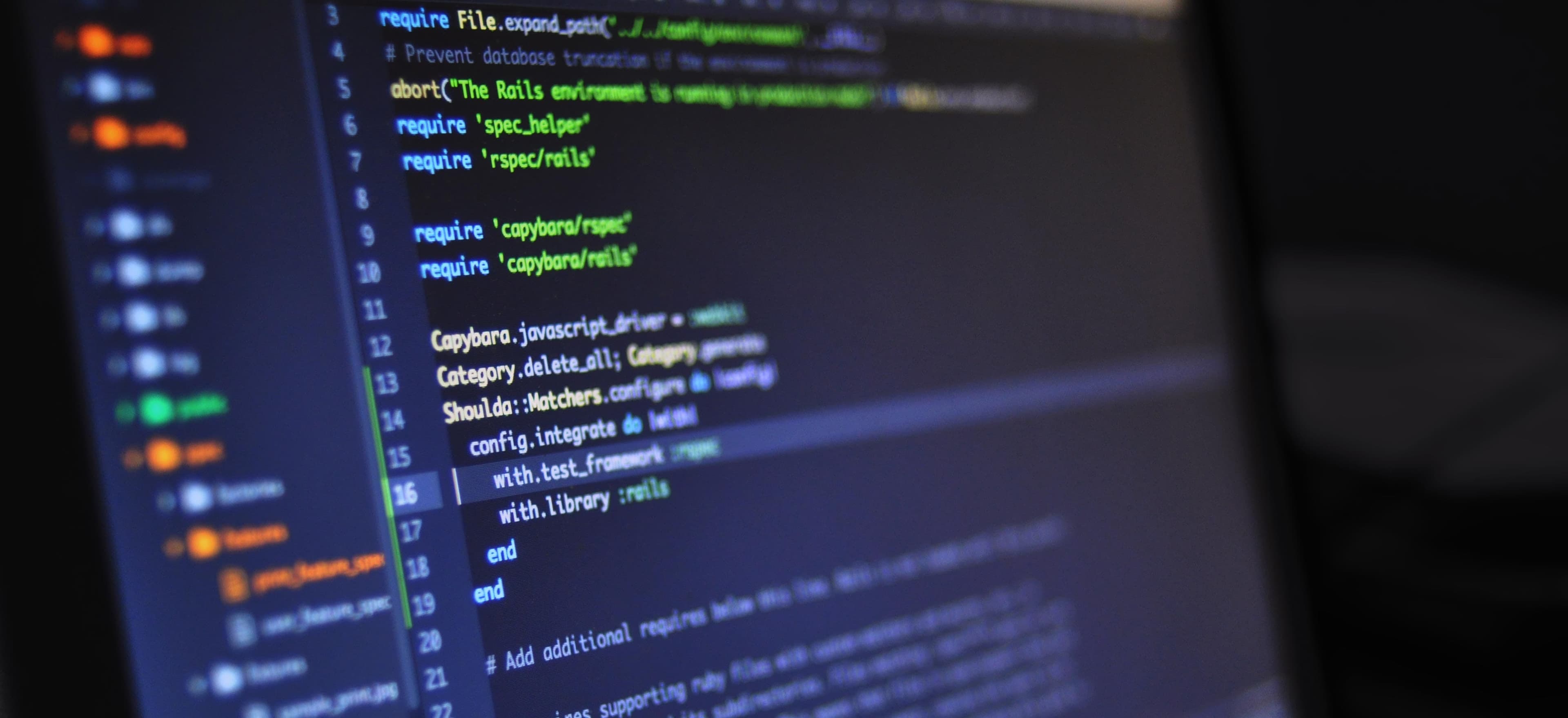
- Published on
Boost Your Trading Game: Simplifying Systems with Akka
In the fast-paced world of financial trading, time isn't just money—it's everything. Given the complex nature of trading systems, which need to process thousands of transactions per second while maintaining high levels of accuracy and reliability, Java developers are constantly on the lookout for more efficient, robust solutions. This is where Akka, a toolkit and runtime for building highly concurrent, distributed, and fault-tolerant event-driven applications on the JVM, comes into play. Today, we're diving deep into how Akka can revolutionize your trading applications, making them more responsive, scalable, and easier to maintain.
Understanding Akka and the Actor Model
Akka leverages the Actor Model to offer a higher level of abstraction for writing concurrent and distributed systems. This model treats "actors" as the fundamental building blocks of an application. Each actor encapsulates state and behavior, communicates with asynchronous messages, and operates concurrently without the need of traditional lock mechanisms. This approach simplifies the development of complex applications by freeing developers from having to deal with explicit locking and thread management, making the system easier to write, understand, and maintain.
Why Akka for Trading Systems?
-
Concurrency Made Simple: Trading systems require handling numerous tasks like reading market data, executing trades, and surveillance, concurrently. Akka actors model allows easy management of these concurrent tasks, making your system highly responsive.
-
Fault Tolerance: The financial domain demands high reliability. Akka provides a sophisticated fault tolerance model based on the let-it-crash philosophy where actors can automatically recover from failures, ensuring that your trading system remains robust at all times.
-
Distributed by Nature: As your trading application grows, so does the need to scale out. Akka supports building distributed systems and offers location transparency. Your actors can reside on different nodes, enabling your system to scale horizontally without significant changes to the codebase.
-
Back Pressure Management: With Akka Streams, you can efficiently manage the flow of data through your system, ensuring that slower components do not get overwhelmed. This is essential for trading systems to maintain consistency and performance during high-frequency trading periods.
Dive into Code: Simplifying Trade Execution with Akka
Let’s code a simple example to demonstrate how Akka can simplify building a trading system. Our focus here is on a trade execution service that processes trade requests. We’ll use SBT (Simple Build Tool) for building Scala applications, though it’s equally feasible with Maven or Gradle for pure Java projects.
Setting Up
First, ensure you have SBT installed. Create a new project directory and initialize a new SBT project:
mkdir akka-trading-system
cd akka-trading-system
sbt new akka/akka-quickstart-scala.g8
Add Akka dependency in your build.sbt
if it's not already there:
libraryDependencies += "com.typesafe.akka" %% "akka-actor-typed" % "2.6.14"
Defining Actors
We define two actors: TradeExecutor
for executing trades and TradeProcessor
for processing the result.
import akka.actor.typed.{ActorSystem, Behavior}
import akka.actor.typed.scaladsl.Behaviors
object TradeExecutor {
final case class ExecuteTrade(tradeId: String, quantity: Int)
def apply(): Behavior[ExecuteTrade] = Behaviors.receive { (context, message) =>
// Execute trade logic
println(s"Executing Trade: ${message.tradeId}")
// Here would be the execution logic
Behaviors.same
}
}
object TradeProcessor {
final case class ProcessTradeResult(tradeId: String, success: Boolean)
def apply(): Behavior[ProcessTradeResult] = Behaviors.receiveMessage { message =>
// Process trade result
println(s"Processed Trade ${message.tradeId} with success: ${message.success}")
Behaviors.same
}
}
Bootstrapping the System
Initialize the actor system and send a message to the TradeExecutor
:
object AkkaTradingSystem extends App {
val tradeSystem = ActorSystem(TradeExecutor(), "tradeSystem")
tradeSystem ! TradeExecutor.ExecuteTrade("trade123", 100)
}
Discussion
This simple example scratches the surface of what's possible with Akka in trading systems. By decoupling components into actors, we've made our system more modular and easier to extend or maintain. Failure in one actor (e.g., a trade execution failing) doesn't crash the system but rather is handled gracefully, allowing for robust recovery strategies.
Lessons Learned
In the demanding world of financial trading, Akka shines as a powerful ally, offering Java developers the tools to build more efficient, reliable, and scalable systems. By abstracting away the complexities of concurrency and distribution, Akka lets you focus on the business logic, making your system easier to develop, understand, and maintain. Whether you're handling millions of transactions or dealing with real-time data processing, incorporating Akka into your trading applications can significantly boost performance and reliability.
Remember, the example provided is a basic demonstration. Real-world trading systems can exploit the full spectrum of Akka's capabilities, including clustering, persistence, and streaming, to build comprehensive, feature-rich applications. The journey into Akka's world is an investment in your system's future, providing you with the tools to navigate the complexities of modern financial trading landscapes efficiently.
For those eager to dive deeper, exploring the official Akka documentation is a good next step. It offers a wealth of information from beginner guides to advanced topics, ensuring you have the knowledge to harness the full power of Akka in your trading solutions. Happy coding, and may your trades always be in your favor!