Smooth JDBC User Store Integration with Identity Server
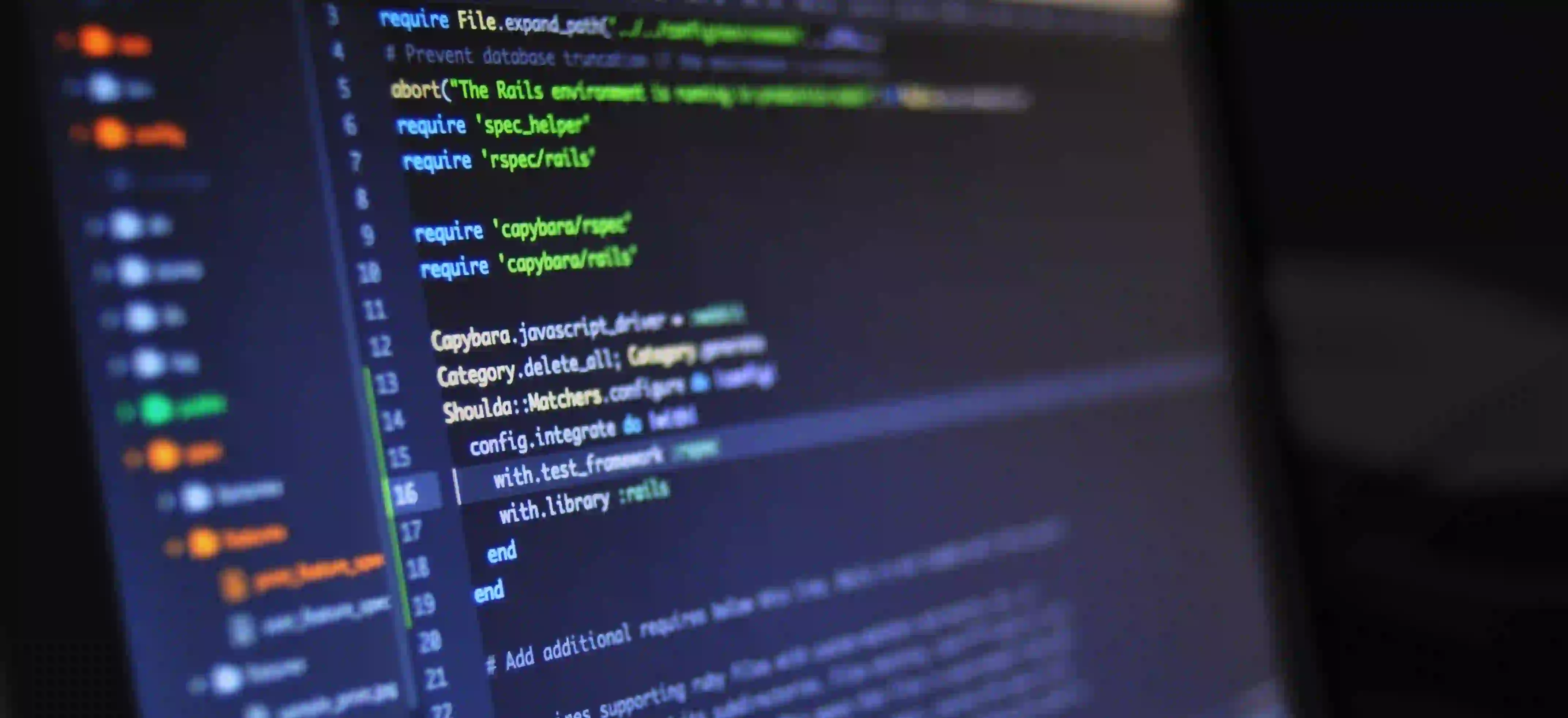
Smooth JDBC User Store Integration with Identity Server
When it comes to managing user information and credentials in a Java application, integrating a JDBC user store with an Identity Server can be a robust solution. This integration provides a seamless way to store and manage user data in a relational database while leveraging the authentication and authorization features provided by the Identity Server.
In this article, we will explore how to smoothly integrate a JDBC user store with an Identity Server using Java. We will cover the necessary steps to set up the JDBC user store, configure the Identity Server to use the JDBC user store, and provide insightful code snippets to illustrate the process.
Setting Up the JDBC User Store
The first step in integrating a JDBC user store with an Identity Server is to set up the database schema to store user information. You can use any relational database such as MySQL, PostgreSQL, or Oracle for this purpose.
Let's consider a simple schema for the user store with a users
table containing the user credentials and a roles
table for storing user roles. Here's an example of the SQL schema for the user store:
CREATE TABLE users (
id INT AUTO_INCREMENT PRIMARY KEY,
username VARCHAR(100) NOT NULL,
password VARCHAR(100) NOT NULL
);
CREATE TABLE roles (
id INT AUTO_INCREMENT PRIMARY KEY,
username VARCHAR(100) NOT NULL,
role_name VARCHAR(50) NOT NULL
);
In the users
table, we store the username
and password
fields, while the roles
table associates users with their respective roles.
Configuring the Identity Server
Once the JDBC user store is set up, the next step is to configure the Identity Server to use the JDBC user store for authentication and authorization.
The Identity Server, such as WSO2 Identity Server, provides a comprehensive set of features for identity and access management. To configure the Identity Server to use the JDBC user store, you need to define the data source, realm configuration, and user store configuration.
Data Source Configuration
The data source configuration involves defining the connection details for the relational database where the user store is located. This typically includes the database URL, username, password, and any specific database driver properties.
Here's an example of configuring a data source for WSO2 Identity Server in the repository/conf/datasources/master-datasources.xml
file:
<datasource>
<name>WSO2_CARBON_DB</name>
<description>The datasource used for user and registry data</description>
<jndiConfig>
<name>jdbc/WSO2CarbonDB</name>
</jndiConfig>
<definition type="RDBMS">
<configuration>
<url>jdbc:mysql://localhost:3306/user_store</url>
<username>db_user</username>
<password>db_password</password>
<driverClassName>com.mysql.jdbc.Driver</driverClassName>
<!-- other database-specific configurations -->
</configuration>
</definition>
</datasource>
Realm Configuration
In the realm configuration, you specify the user store manager and the user store properties. This includes defining the user store manager class, user store properties such as connection URL, username, password, and other relevant configurations.
The following is an example of configuring a JDBC user store in the WSO2 Identity Server user-mgt.xml
file:
<UserManager>
<Realm>
<Configuration>
<AddAdmin>true</AddAdmin>
<AdminRole>admin</AdminRole>
<AdminUser>
<UserName>admin</UserName>
<Password>admin</Password>
</AdminUser>
<EveryOneRoleName>everyone</EveryOneRoleName>
<Property name="dataSource">jdbc/WSO2CarbonDB</Property>
</Configuration>
<UserStoreManager class="org.wso2.carbon.user.core.jdbc.JDBCUserStoreManager">
<Property name="TenantManager">org.wso2.carbon.user.core.tenant.JDBCTenantManager</Property>
<Property name="ReadOnly">false</Property>
<Property name="MaxUserNameListLength">100</Property>
<!-- other user store manager properties -->
</UserStoreManager>
</Realm>
</UserManager>
Integrating with Java Application
With the Identity Server configured to use the JDBC user store, you can now integrate your Java application with the Identity Server's authentication and authorization mechanisms.
JDBC User Store Authentication
To authenticate users against the JDBC user store from your Java application, you can use the JDBC realm provided by the Identity Server. The JDBC realm allows you to validate user credentials against the JDBC user store.
Here's an example of using the JDBC realm for user authentication in a Java web application:
// Define the JDBC realm for user authentication
RealmConfiguration realmConfig = new RealmConfiguration();
realmConfig.setUserStoreClass("org.wso2.carbon.user.core.jdbc.JDBCUserStoreManager");
realmConfig.setAdminUserName("admin");
realmConfig.setAdminPassword("admin");
// Set other realm configurations
// Authenticate user using JDBC realm
UserRealm userRealm = new JDBCUserRealm(realmConfig);
boolean isAuthenticated = userRealm.getUserStoreManager().authenticateUser(username, password);
if (isAuthenticated) {
// User authenticated successfully
} else {
// Authentication failed
}
Role-Based Authorization
Once a user is authenticated, you may need to perform role-based authorization within your Java application. The Identity Server provides the necessary APIs to retrieve and validate user roles from the JDBC user store.
Here's an example of role-based authorization in Java using the Identity Server's APIs:
// Retrieve user roles from the Identity Server
String[] userRoles = userRealm.getUserStoreManager().getRoleListOfUser(username);
// Validate user roles for authorization
if (Arrays.asList(userRoles).contains("admin")) {
// User has admin role
// Perform admin-specific tasks
} else {
// User does not have admin role
// Handle unauthorized access
}
In Conclusion, Here is What Matters
Integrating a JDBC user store with an Identity Server provides a robust solution for managing user information and credentials in a Java application. By following the steps outlined in this article, you can seamlessly configure the Identity Server to use a JDBC user store and leverage its authentication and authorization mechanisms within your Java application.
As always, it's essential to ensure the security and integrity of user data when implementing such systems. Additionally, staying informed about the best practices in identity management and security is crucial for maintaining a robust and secure application.
In conclusion, the integration of a JDBC user store with an Identity Server empowers Java applications with scalable, secure, and efficient user management capabilities.
By implementing a smooth integration of JDBC user store with an Identity Server, Java applications can level up their user management functionalities while enhancing security. Through this tutorial, developers can gain insights into setting up the JDBC user store, configuring the Identity Server, and integrating the Java application seamlessly. Seamless management of user information and credentials, enhanced security, and streamlined user authentication and authorization are guaranteed with this integration.