Fixing Java Decompiler Issues in JDeveloper: A Guide
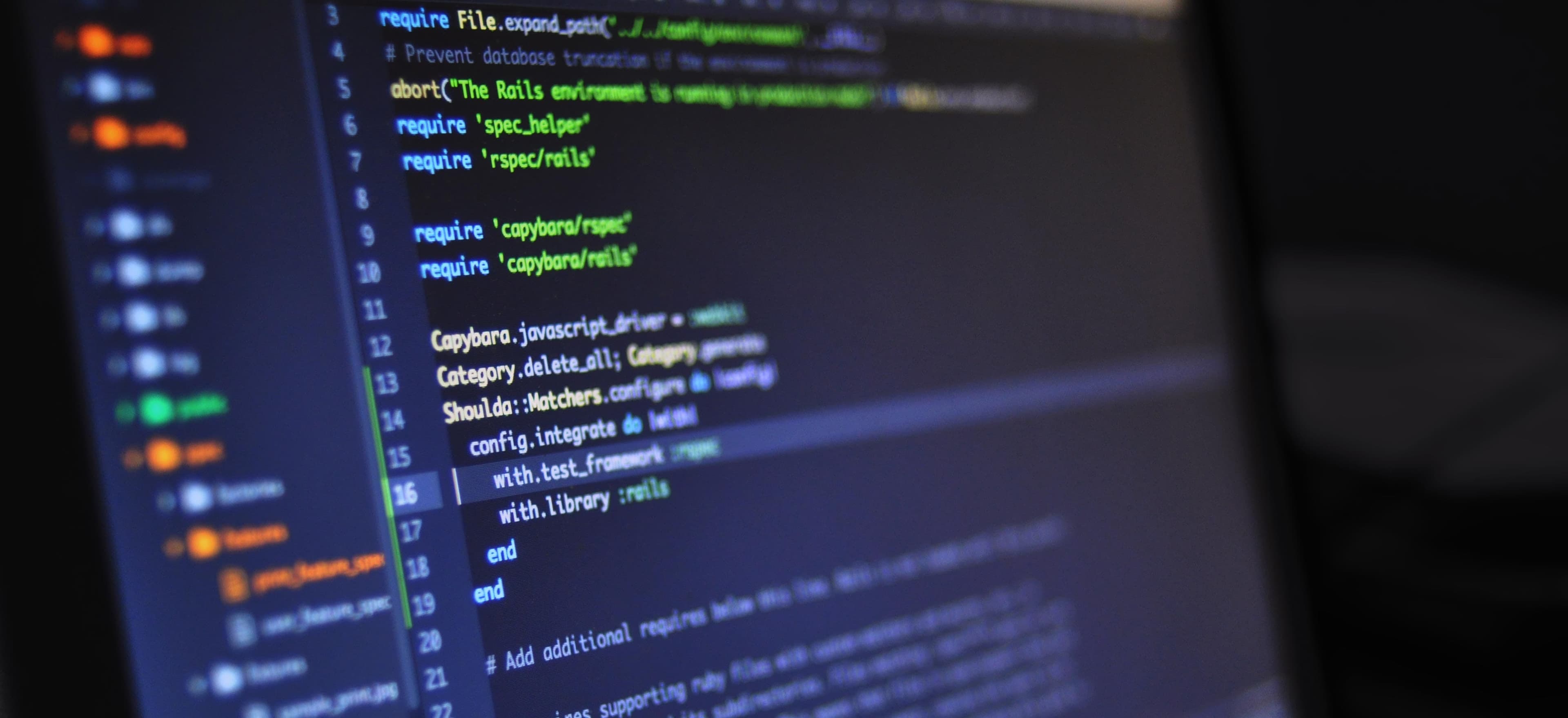
- Published on
Fixing Java Decompiler Issues in JDeveloper: A Complete Guide
Java development can sometimes feel like a tightrope walk, especially when tools act up unexpectedly. JDeveloper, Oracle's flagship integrated development environment (IDE) for Java, is no stranger to this rule. One such hiccup that can seriously hinder your workflow involves its decompiler. A decompiler is vital for developers; it turns the compiled .class
files back into readable Java code, which can be a lifesaver when dealing with lost sources or trying to understand third-party libraries. But what do you do when the decompiler in JDeveloper isn't playing ball?
Fear not! This guide is here to walk you through the troubleshooting steps to fix common Java decompiler issues in JDeveloper, ensuring your development process is smooth and uninterrupted.
Understanding the Problem
Before diving into the solutions, let's understand what could go wrong. Decompiler issues in JDeveloper can manifest in several ways, ranging from the decompiler not activating when needed, showing error messages, or producing incomplete or incorrect code.
Solution 1: Ensuring Correct Decompiler Configuration
The first step is to ensure that the decompiler is correctly configured in JDeveloper. JDeveloper supports integrating external decompilers, so let's focus on configuring one of the most popular ones – JD-GUI.
-
Download JD-GUI: Obtain JD-GUI from its official website (http://java-decompiler.github.io/). This step is critical as using the latest version ensures compatibility and feature completeness.
-
Integrate JD-GUI with JDeveloper: Integration typically involves updating JDeveloper's
ide.conf
orjdev.conf
files to recognize JD-GUI. These files can usually be found in thebin
directory of your JDeveloper installation.Here's an example snippet to add to your
ide.conf
:AddVMOption -Djdeveloper.decompiler.path=/path/to/jd-gui.exe
Replace
/path/to/jd-gui.exe
with the actual path where JD-GUI is installed on your system. This tells JDeveloper where to find the decompiler. -
Restart JDeveloper: For the changes to take effect.
Checking the configuration is a quick win, often resolving most issues without further ado.
Solution 2: Updating JDeveloper and Decompiler
Software updates are a fact of digital life, often packing bug fixes, improved performances, and new features:
-
Update JDeveloper: Oracle frequently releases updates for JDeveloper. Check for any available updates on Oracle's official site. An updated version might contain fixes for your decompiler issues.
-
Update JD-GUI: Similarly, ensure your JD-GUI is the latest version. Developers constantly enhance decompilers, and staying updated can resolve previously encountered issues.
Occasionally, the problem isn’t with the tool but with the environment it operates in. Ensuring both JDeveloper and the decompiler are up-to-date is an easy yet effective solution.
Solution 3: Exploring Alternative Decompilers
If JD-GUI isn't cutting it, exploring alternatives might be your best bet. Other popular Java decompilers include Procyon, CFR, and Fernflower. Integrating a different decompiler is similar to the steps outlined above but customized to the specific tool.
Consider this example using CFR:
-
Download CFR: First, obtain the latest version from its GitHub repository (https://github.com/leibnitz27/cfr).
-
Configuration: Depending on the decompiler, you might configure it directly in JDeveloper or through external scripts. With CFR, you might need to include it as a library in your JDeveloper project and call it programmatically.
Here’s a simple snippet on how to use CFR programmatically:
import org.benf.cfr.reader.api.CfrDriver;
Map<String, String> options = new HashMap<>();
options.put("outputdir", "<output-directory>");
CfrDriver driver = new CfrDriver.Builder().withOptions(options).build();
driver.analyse(Collections.singletonList("<path-to-class-file>"));
This snippet demonstrates how to direct CFR to decompile a specific class file to a chosen directory. Make sure to replace <output-directory>
and <path-to-class-file>
with your actual paths.
Solution 4: Checking for Bytecode Compatibility
Sometimes, the issue isn't with the decompiler or its configuration but with the Java bytecode itself. With each Java version, bytecode might evolve, potentially rendering older decompilers incapable of correctly interpreting newer constructs.
Ensure that your decompiler supports the Java version used to compile the bytecode. Updating the decompiler or switching to one that supports the newer versions can resolve these issues.
Helpful Tips and Debugging Practices
- Verbose Output: Most decompilers provide options for verbose output, which can be invaluable for diagnosing issues.
- Minimal Reproducible Example: If you're stuck, try to isolate the problem to a minimal, reproducible example. This step can often highlight the precise issue, whether it's a configuration mistake, a bug in the decompiler, or something else entirely.
- Community Support: Don't forget the power of community. Forums, Stack Overflow, and even the official support channels for JDeveloper or the decompiler you're using can provide insights and solutions.
By approaching Java decompiler issues in JDeveloper methodically, you can often quickly identify and address the root cause, minimizing disruption to your development process. Whether it's double-checking configurations, keeping your tools up to date, exploring alternative solutions, or ensuring compatibility with Java bytecode, there's usually a path forward. Happy coding!