Redefining Boundaries: Innovative Approaches to Markers
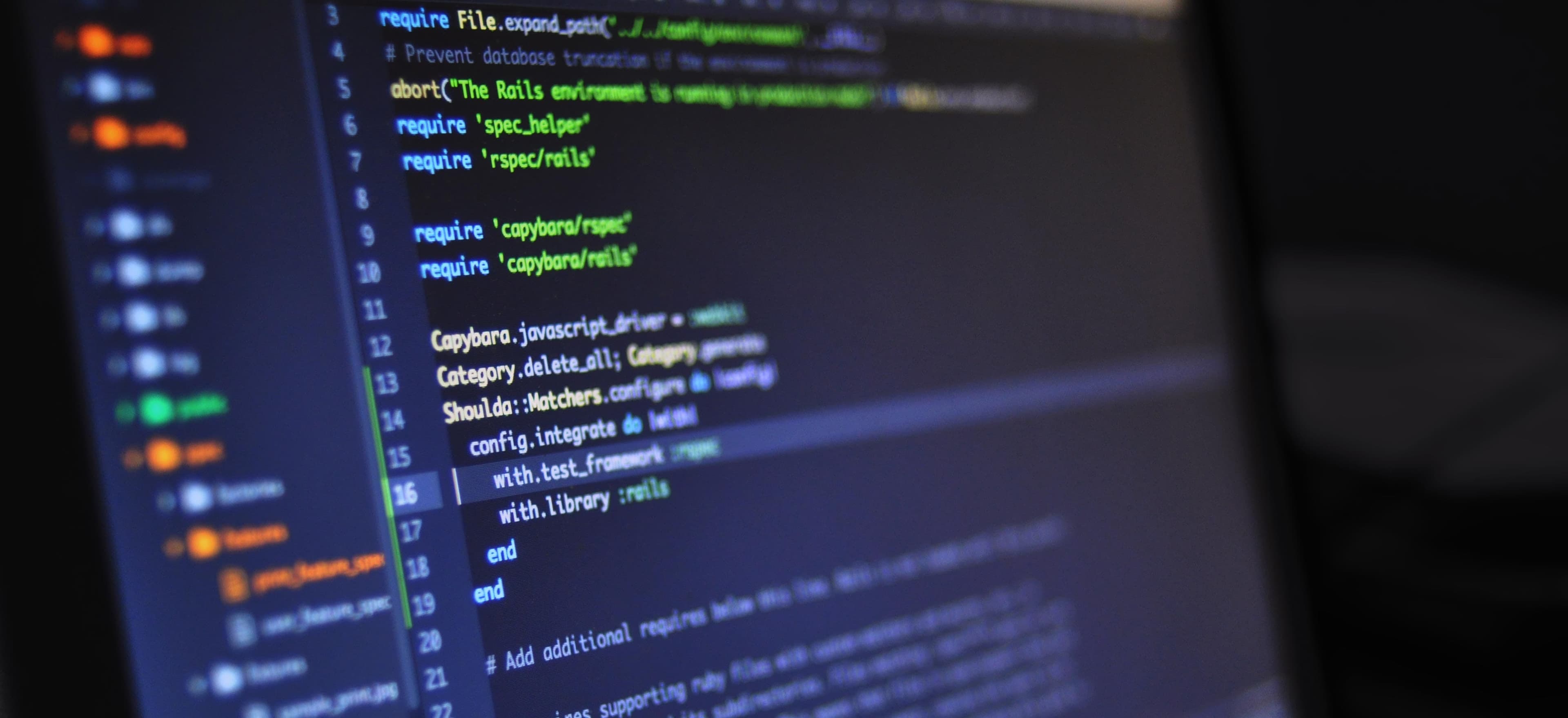
- Published on
Redefining Boundaries: Innovative Approaches to Java Markers
Markers are a crucial aspect of Java programming, allowing developers to categorize and organize code efficiently. In this article, we will explore innovative approaches to markers in Java, demonstrating how they can be utilized to enhance code readability, maintainability, and extensibility.
Understanding Markers in Java
In Java, markers are often used as a form of metadata to label or categorize code elements such as classes, methods, or variables. They serve as annotations that convey additional information about the marked element. While markers are commonly associated with annotations such as @Deprecated
or @Override
, they can be leveraged creatively to fulfill a variety of purposes.
Leveraging Marker Interfaces
One innovative approach to markers in Java is the use of marker interfaces. A marker interface is an interface with no methods, serving only to mark classes that implement it. This technique allows for the categorization of classes based on their functionality or characteristics.
Consider the following example, where we define a marker interface Drawable
:
public interface Drawable {
// No methods
}
We can then have various classes implement the Drawable
interface to indicate that they are drawable elements. For instance:
public class Circle implements Drawable {
// Class implementation
}
By utilizing marker interfaces, we can effectively categorize and identify classes based on their common traits, enabling more streamlined processing and organization of the codebase.
Custom Annotations as Markers
Custom annotations can also be employed as markers to convey specific information about code elements. By creating custom annotations, developers can define their markers with associated attributes, providing additional context or instructions.
Let's create a custom annotation @ConcurrencySafe
to mark classes that are designed to be thread-safe:
import java.lang.annotation.ElementType;
import java.lang.annotation.Target;
@Target(ElementType.TYPE)
public @interface ConcurrencySafe {
String description() default "This class is designed to be thread-safe";
}
We can then apply the @ConcurrencySafe
marker to relevant classes:
@ConcurrencySafe
public class ConcurrentQueue {
// Class implementation
}
By utilizing custom annotations as markers, developers can effectively communicate the intended usage or behavior of code elements, promoting better understanding and maintenance of the codebase.
Marker-Based Component Scanning in Spring Framework
In the context of the Spring Framework, markers play a vital role in component scanning, a process that automatically detects and instantiates Spring components. By leveraging markers, developers can efficiently identify and manage components within the application.
Consider the use of the @Component
marker annotation in Spring:
import org.springframework.stereotype.Component;
@Component
public class UserService {
// Class implementation
}
This usage of marker annotations allows the Spring container to detect and register the UserService
component without explicit configuration, streamlining the development process.
The 'Why' Behind Marker Innovation
The innovative approaches to markers in Java discussed above offer various benefits that align with modern software development practices. Here's why these strategies are valuable:
Enhanced Readability and Maintainability
By effectively utilizing markers, developers can improve the readability of code and facilitate easier navigation within the codebase. Marker interfaces and custom annotations serve as descriptive indicators, allowing developers to discern the purpose and characteristics of code elements at a glance.
Promoting Extensibility and Modularization
Markers enable a more modular approach to software design, facilitating the organization of code elements into distinct categories based on their functional or structural attributes. This supports extensibility, as new functionality can be seamlessly integrated into the appropriate categories through marker-based identification.
Facilitating Framework Integration and Interoperability
In the context of frameworks such as Spring, marker annotations play a pivotal role in enabling seamless integration and interoperability. Leveraging markers aligns with the conventions and patterns established by the framework, ensuring smooth collaboration with the underlying infrastructure.
In conclusion, the innovative approaches to markers in Java outlined in this article underscore the significance of leveraging metadata to enhance code organization, communication, and extensibility. By embracing these strategies, developers can cultivate codebases that are not only functional but also well-structured and easily maintainable.
Incorporating marker-based techniques into Java development practices can yield substantial improvements in code clarity, modularity, and integration with frameworks, ultimately contributing to the development of robust and scalable software solutions.
For further exploration of Java annotations and markers, refer to the official Java Annotations Documentation and the Java Marker Interfaces guide.
The realm of Java markers continues to evolve, presenting abundant opportunities for innovative applications and best practices. Embrace the power of markers, and redefine the boundaries of Java programming with ingenuity and precision.