Easy Password Hash Conversion in Grails: A Guide
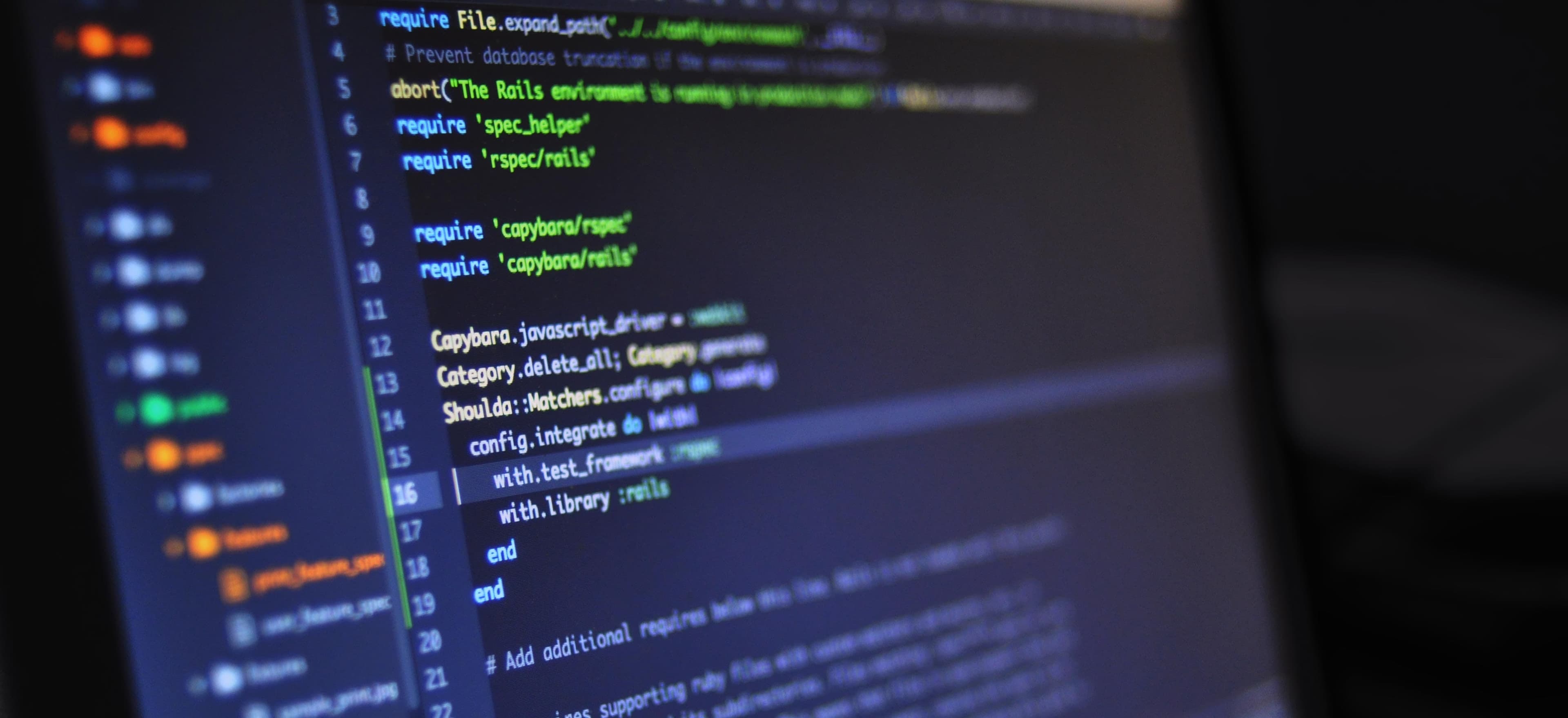
- Published on
Easy Password Hash Conversion in Grails: A Guide
In web development, securing user passwords is of utmost importance. Hashing passwords before storing them in a database is a common and effective security measure. In this blog post, we will explore how to easily hash and convert passwords in a Grails application using the powerful Bcrypt algorithm.
Understanding Password Hashing
Before diving into the code, let's briefly discuss what password hashing is and why it's crucial for web applications. Password hashing is the process of converting a plain-text password into a hashed, irreversible string of characters. This hashed password is then stored in the database. When a user tries to log in, the system hashes the entered password and compares it with the stored hash, granting access if they match.
Hashing passwords is essential for security because it ensures that even if the database is compromised, the passwords are not easily decipherable. Additionally, using a strong hashing algorithm further enhances the security of user passwords.
Introducing Bcrypt
Grails, a powerful web application framework based on the Groovy language, comes with excellent support for password hashing using the Bcrypt algorithm. Bcrypt is a well-established hashing algorithm known for its strength and robustness. It uses a salt to protect against rainbow table attacks and allows for customizable cost factors, making it adaptable to future hardware improvements.
Implementation in Grails
Let's now delve into the implementation of password hashing with Bcrypt in a Grails application. We'll start by creating a simple user domain class and then proceed to demonstrate how to hash and convert passwords before persisting them to the database.
Setting Up the User Domain Class
// User.groovy
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder
class User {
String username
String password
def beforeInsert() {
encodePassword()
}
def encodePassword() {
password = new BCryptPasswordEncoder().encode(password)
}
}
In the above code, we have a basic User
domain class with username
and password
properties. We've utilized the BCryptPasswordEncoder
provided by the Spring Security plugin in Grails to hash the password before it is inserted into the database. The beforeInsert
hook ensures that the encodePassword
method is called before the user is persisted.
Why Use beforeInsert
Hook?
Using the beforeInsert
hook ensures that the password is hashed only when a new user instance is created and persisted. This approach separates the concerns of user management and password hashing, keeping the code clean and maintainable.
Creating a User
Now, let's create a new user using the User
class and observe the password hashing in action.
def user = new User(username: 'john_doe', password: 'secret123')
user.save(failOnError: true)
Upon executing the code above, the password 'secret123' will be hashed using Bcrypt and stored in the database. This one-time hashing provides a reliable and secure way of safeguarding user passwords.
Verifying Passwords
In addition to hashing and persisting passwords, it's essential to verify hashed passwords during the login process. Let's see how we can achieve this in a Grails application.
Verifying the Password
// UserController.groovy
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder
def login(String username, String password) {
def user = User.findByUsername(username)
if (user && new BCryptPasswordEncoder().matches(password, user.password)) {
// User authenticated
} else {
// Authentication failed
}
}
In the code snippet above, when a user attempts to log in with a username and password, we retrieve the user from the database based on the provided username. We then use the matches
method of BCryptPasswordEncoder
to compare the entered password with the stored hashed password. If the passwords match, the user is authenticated. If not, the authentication fails.
Why Use matches
Method?
The matches
method provided by BCryptPasswordEncoder
compares a plain-text password with a hashed password. It handles the complexity of hashing and comparison, making it easy to verify passwords securely.
To Wrap Things Up
In this guide, we've explored the importance of password hashing and how to implement it in a Grails application using the Bcrypt algorithm. By utilizing the BCryptPasswordEncoder
and Grails' domain class hooks, we've ensured that user passwords are securely hashed and verified.
It's important to note that while Bcrypt is a robust hashing algorithm, staying informed about the latest industry practices and periodically updating the hashing mechanisms in your application is crucial for maintaining strong security standards.
With the knowledge gained from this guide, you are now well-equipped to strengthen the security of user passwords in your Grails application. Keep coding securely!