EasyMock Woes: Solving Common Setup Struggles
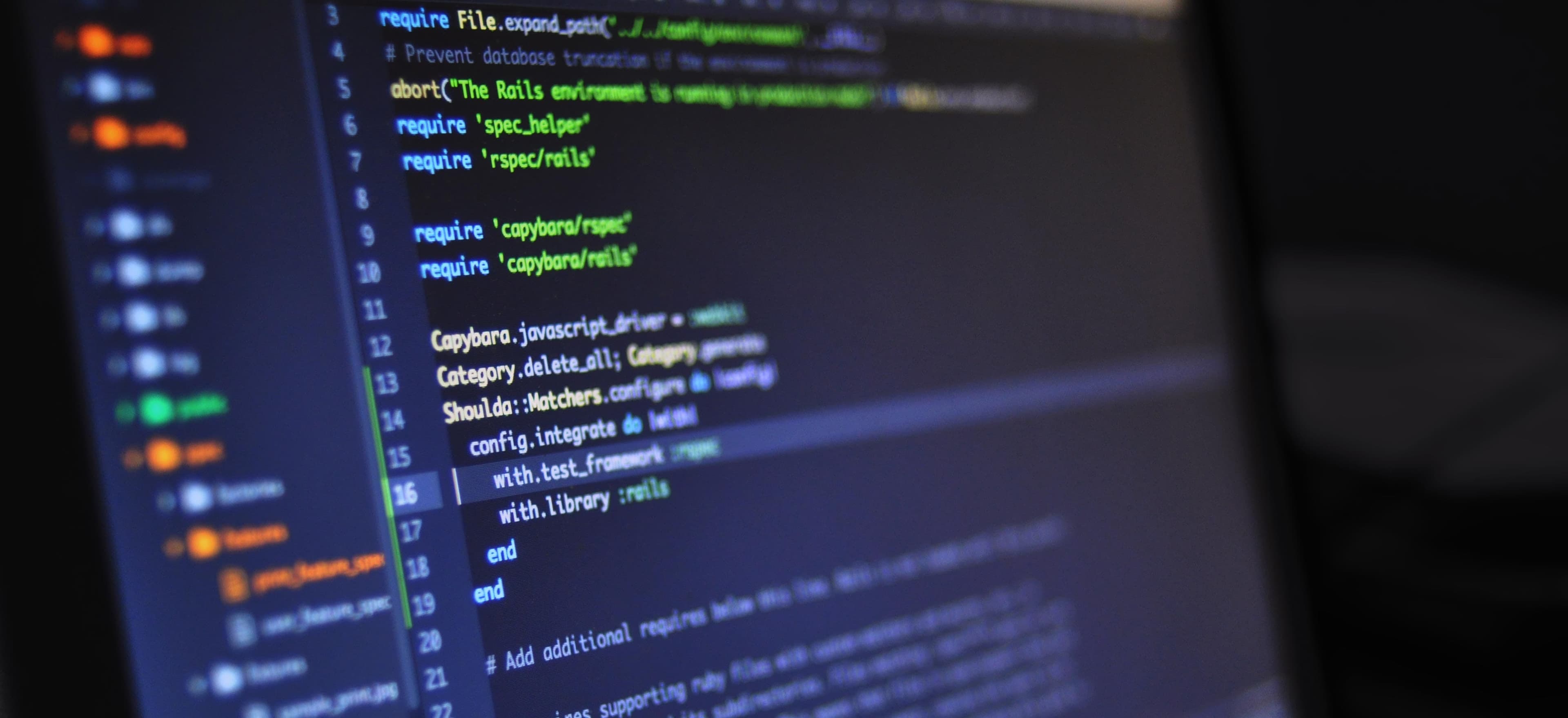
- Published on
EasyMock Woes: Solving Common Setup Struggles
When it comes to writing unit tests for Java applications, EasyMock is a widely-used and powerful tool for creating mock objects. However, while EasyMock can provide significant benefits in terms of test coverage and quality, it is not without its challenges. In this post, we will address some common setup struggles that developers encounter when using EasyMock and provide solutions to overcome them.
Understanding EasyMock Basics
Before we delve into the common setup struggles, let's have a quick refresher on the basics of EasyMock.
EasyMock is a Java library that provides an easy way to create mock objects for interfaces and classes. Mock objects are simulated objects that mimic the behavior of real objects, allowing developers to test the interactions between objects in isolation.
The key components of EasyMock include:
- Creating mock objects using the
createMock
method - Recording the expected behavior of the mock objects using the
expect
method - Replaying the behavior using the
replay
method - Verifying that the expected behavior occurred using the
verify
method
With this understanding in place, let's explore some common setup struggles and how to address them.
1. Dealing with Unnecessary Expectations
One common struggle when using EasyMock is setting up unnecessary expectations, leading to overly complex and brittle tests. It's important to focus on the essential interactions between objects and avoid cluttering the test with unnecessary expectations.
// Bad example: Unnecessary expectation
expect(mockObject.someMethod()).andReturn(someValue);
The above code sets up an expectation for a method call that may not be relevant to the specific behavior being tested. It can lead to tightly-coupled tests that break easily when the implementation changes.
To address this, always prioritize setting up expectations that are directly related to the behavior being tested. This ensures that the tests remain focused and resilient to changes in the codebase.
2. Over-Reliance on Concrete Implementations
Another common struggle is an over-reliance on concrete implementations when creating mock objects. This can lead to tightly-coupled tests that are difficult to maintain and refactor.
// Bad example: Mocking a concrete class
ConcreteClass mockObject = createMock(ConcreteClass.class);
Instead of directly mocking concrete classes, it's recommended to favor interfaces over concrete implementations. By mocking interfaces, tests become more resilient to changes in the underlying implementations, allowing for greater flexibility and maintainability.
3. Complex Setup for Void Methods
When dealing with void methods in EasyMock, developers often struggle with setting up the expected behavior, as void methods do not have a return value to specify.
// Bad example: Setting up expectations for a void method
mockObject.voidMethod();
expectLastCall().once();
To address this, use the expectLastCall
method to set the expected behavior for void methods. This approach allows developers to specify the number of expected calls and other behaviors such as throwing exceptions or executing callbacks.
4. Misusing Argument Matchers
EasyMock provides argument matchers as a way to specify flexible expectations for method arguments. However, misusing argument matchers can lead to unexpected behavior and difficulties in debugging failing tests.
// Bad example: Misusing argument matchers
expect(mockObject.someMethod(eq("expectedValue"))).andReturn(someValue);
When using argument matchers, it's important to ensure that they are used appropriately and consistently. Overuse or misuse of argument matchers can make tests harder to understand and maintain. Instead, consider using concrete values where possible and argument matchers only when necessary for flexible expectations.
5. Failing to Verify Expected Behavior
One common struggle, especially for developers new to EasyMock, is forgetting to verify the expected behavior after the test has been executed. Without verifying the expectations, it's possible for tests to pass even when the expected behavior did not occur.
// Bad example: Failing to verify expected behavior
replay(mockObject);
// ... test execution
// Missing verify step
Always remember to call the verify
method after executing the test to ensure that the expected behavior has occurred. This step is crucial for the reliability of the tests and provides confidence that the interactions between objects are as expected.
The Last Word
In this post, we've addressed common setup struggles encountered when using EasyMock and provided solutions to overcome them. By prioritizing essential expectations, favoring interfaces over concrete implementations, handling void methods effectively, using argument matchers judiciously, and verifying expected behavior, developers can harness the full power of EasyMock in unit testing Java applications.
It's important to remember that while EasyMock can be a valuable tool for creating mock objects, it's essential to use it judiciously and with a focus on simplicity and maintainability. By following best practices and understanding the nuances of setting up mock objects, developers can write robust and reliable unit tests that contribute to the overall quality of their Java applications.
For further reading and advanced techniques with EasyMock, consider exploring the official EasyMock documentation and the various resources available online.
Happy testing with EasyMock!
Checkout our other articles