Boost Your Data Game: Top JPA Query Hacks Unveiled!
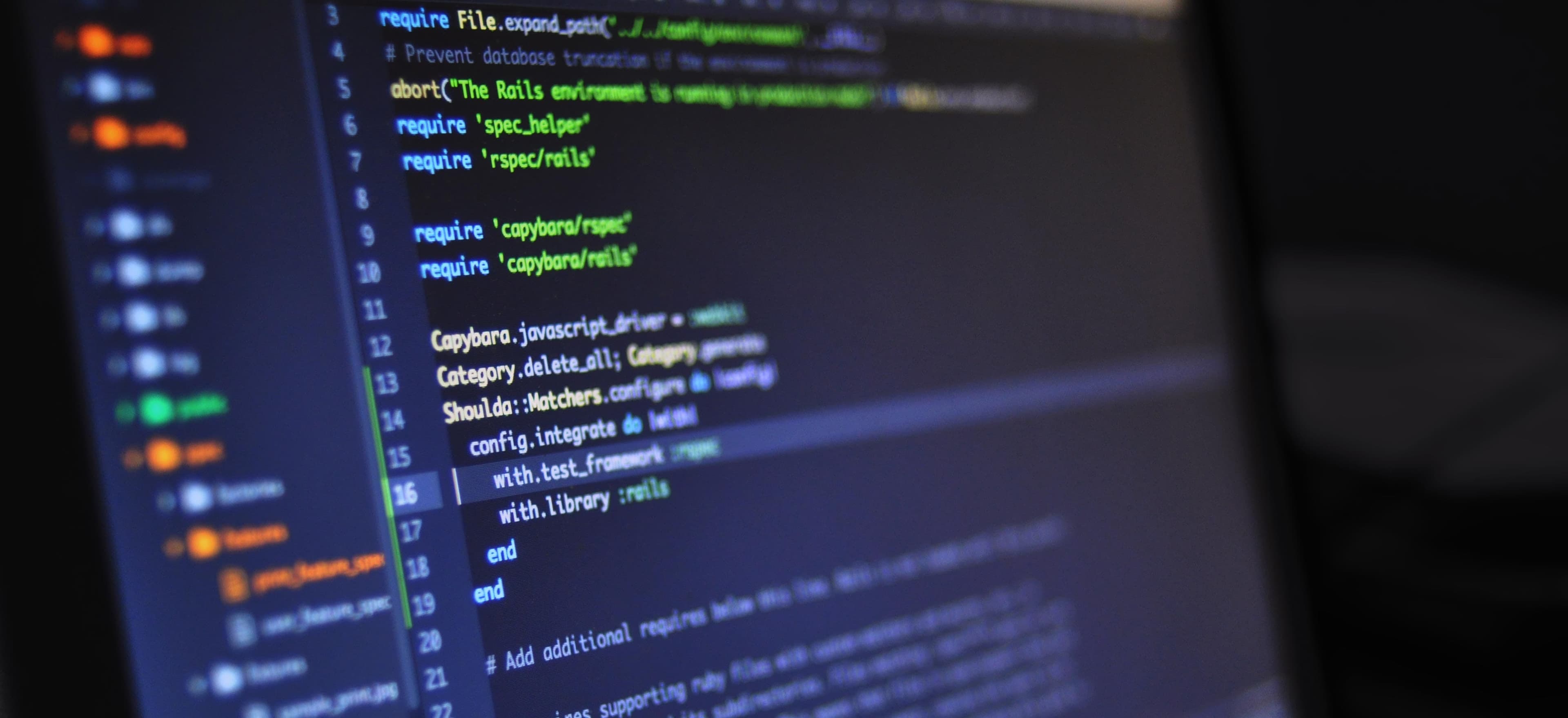
- Published on
Boost Your Data Game: Top JPA Query Hacks Unveiled!
Are you a Java developer looking to take your data access to the next level? If so, you're in the right place. In this post, we'll explore some powerful Java Persistence API (JPA) query optimizations and hacks that will supercharge your data manipulation and retrieval processes.
Understanding JPA
Before we dive into the nitty-gritty details, let's take a quick refresher on JPA. Java Persistence API (JPA) is a Java specification for accessing, persisting, and managing data between Java objects and a relational database. It provides a way to work with ORM (Object-Relational Mapping) in Java applications.
Why Optimize JPA Queries?
Efficient database access is crucial for the performance of any application dealing with data. Slow and inefficient queries can lead to decreased application responsiveness and scalability issues. By optimizing JPA queries, you can significantly enhance the overall efficiency and speed of your application.
Top JPA Query Hacks
1. Use Lazy Loading for Relationships
When working with JPA entities that have relationships, it's essential to optimize the data retrieval process. By default, JPA uses lazy loading for relationships, which means the related entities are fetched from the database only when accessed for the first time. This can prevent unnecessary data retrieval and improve performance.
Example:
@Entity
public class Order {
// Other fields
@OneToMany(fetch = FetchType.LAZY)
private List<OrderItem> orderItems;
// Getters and setters
}
2. Leverage JPA Criteria API
The JPA Criteria API provides a programmatic and type-safe way to build queries dynamically. It allows you to construct query criteria, predicates, and expressions using Java method calls. This can be especially useful when you need to build complex queries dynamically at runtime.
Example:
CriteriaBuilder cb = entityManager.getCriteriaBuilder();
CriteriaQuery<Order> query = cb.createQuery(Order.class);
Root<Order> root = query.from(Order.class);
query.select(root).where(cb.equal(root.get("status"), OrderStatus.COMPLETED));
List<Order> completedOrders = entityManager.createQuery(query).getResultList();
3. Batch Fetching for Performance
Fetching large amounts of data can be a performance bottleneck. JPA provides batch fetching, which allows you to optimize data retrieval by fetching related entities in batches rather than individually. This reduces the number of database round trips, improving overall performance.
Example:
@Entity
public class Order {
// Other fields
@OneToMany(fetch = FetchType.LAZY)
@BatchSize(size = 10)
private List<OrderItem> orderItems;
// Getters and setters
}
4. Use Named Queries for Code Organization
Named queries allow you to define queries in a central location, typically near the entity that they operate on. This promotes better code organization and maintenance by separating the query logic from the rest of the codebase. Named queries are defined using annotations on the entity class.
Example:
@Entity
@NamedQuery(name = "Order.findCompletedOrders", query = "SELECT o FROM Order o WHERE o.status = 'COMPLETED'")
public class Order {
// Entity fields and methods
}
5. Optimistic Locking for Concurrency Control
Optimistic locking is a technique used to prevent conflicts and data inconsistency in concurrent database access scenarios. JPA provides support for optimistic locking through versioned entities. By using version columns, JPA can automatically manage and resolve concurrent modification issues.
Example:
@Entity
public class Order {
// Other fields
@Version
private Long version;
// Getters and setters
}
6. Native SQL Queries for Complex Operations
While JPA provides a powerful set of features for working with entities and relationships, there are scenarios where native SQL queries are more suitable, especially for complex operations and database-specific functionality that may not be directly supported by JPA. JPA allows you to execute native SQL queries using the createNativeQuery
method.
Example:
Query query = entityManager.createNativeQuery("SELECT * FROM orders WHERE order_date > :startDate", Order.class);
query.setParameter("startDate", someDate);
List<Order> orders = query.getResultList();
Closing the Chapter
Optimizing JPA queries is essential for maximizing the performance and efficiency of your data access layer. By leveraging the powerful features and optimizations provided by JPA, you can ensure that your Java applications perform at their best when interacting with the database.
So, go ahead and put these JPA query hacks to good use in your next Java project, and witness a significant boost in your data access capabilities!
Ready to elevate your Java skills even further? Check out these Java Development Resources to stay ahead in the game!