How to handle SQL clauses in Java 8 streams
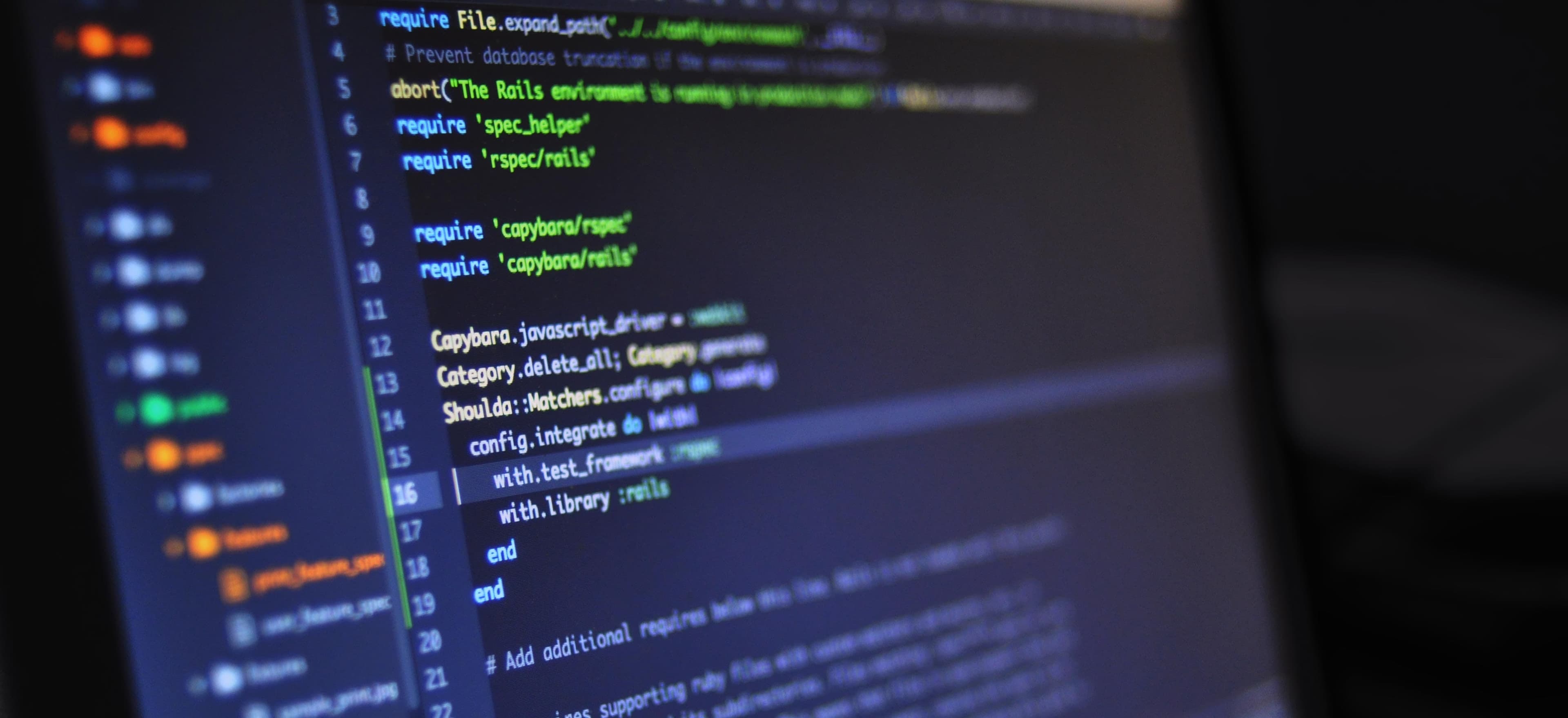
- Published on
Dealing with SQL Clauses in Java 8 Streams
Java 8 streams have revolutionized the way we work with collections in Java. With the introduction of lambda expressions and functional interfaces, processing data in a more declarative and functional manner has become a breeze. In this article, we will explore how to handle SQL clauses in Java 8 streams, allowing for cleaner and more concise code when working with collections of objects.
Filtering Data with WHERE Clause
In SQL, the WHERE
clause is used to filter records based on a specified condition. Similarly, in Java 8 streams, the filter
method is used to select elements based on a given predicate. Let's say we have a list of Person
objects and we want to filter out the persons whose age is greater than 30. We can achieve this using the filter
method:
List<Person> filteredPersons = persons.stream()
.filter(person -> person.getAge() > 30)
.collect(Collectors.toList());
In the above example, persons
is a list of Person
objects. We use the stream
method to convert the list into a stream, and then we use the filter
method to apply the condition for age greater than 30. Finally, we collect the filtered elements into a new list using the Collectors.toList()
method.
Mapping Data with SELECT Clause
The SELECT
clause in SQL is used to retrieve specific columns from a table. In Java 8 streams, the map
method is used to transform elements from one form to another. For example, if we have a list of Person
objects and we want to retrieve only the names of these persons, we can use the map
method:
List<String> personNames = persons.stream()
.map(Person::getName)
.collect(Collectors.toList());
In this example, we use the map
method to transform each Person
object into their respective names. The Person::getName
is a method reference which is equivalent to person -> person.getName()
. Finally, we collect the transformed names into a new list.
Sorting Data with ORDER BY Clause
The ORDER BY
clause in SQL is used to sort the result set in ascending or descending order. In Java 8 streams, the sorted
method is used to sort elements based on a comparator. If we have a list of Person
objects and we want to sort them based on their age in descending order, we can use the sorted
method:
List<Person> sortedPersons = persons.stream()
.sorted(Comparator.comparing(Person::getAge).reversed())
.collect(Collectors.toList());
In this example, we use the sorted
method with a comparator that compares the ages of the persons in descending order using the reversed
method. Finally, we collect the sorted elements into a new list.
Combining Multiple Clauses
In SQL, we often combine multiple clauses to form complex queries. The same can be achieved in Java 8 streams by chaining multiple stream operations. For example, let's say we want to select the names of persons whose age is greater than 30 and sort them in ascending order:
List<String> selectedAndSortedNames = persons.stream()
.filter(person -> person.getAge() > 30)
.map(Person::getName)
.sorted()
.collect(Collectors.toList());
In this example, we chain the filter
, map
, and sorted
methods to first filter the persons based on age, then map them to their names, and finally sort the names in ascending order. The result is a list of selected and sorted names.
Dealing with NULL values
In SQL, handling NULL values is a crucial aspect of querying. Similarly, when working with Java 8 streams, it's important to handle null values properly to avoid NullPointerExceptions. The Optional
class in Java provides a way to represent optional values instead of null references. We can use Optional
to handle potentially null elements when working with streams:
Optional<Person> firstPerson = persons.stream()
.filter(person -> person.getAge() > 30)
.findFirst();
if (firstPerson.isPresent()) {
System.out.println("First person over 30: " + firstPerson.get().getName());
} else {
System.out.println("No person found over 30 years old");
}
In this example, we use the findFirst
method to retrieve the first person over 30 years old, and we wrap the result in an Optional
to check if the result is present before accessing it. This helps to handle the possibility of no matching elements without throwing a NullPointerException.
My Closing Thoughts on the Matter
In conclusion, Java 8 streams provide a powerful and expressive way to handle data processing in a manner similar to SQL clauses. The filter
, map
, sorted
, and other stream operations allow for concise and readable code when dealing with collections of objects. By leveraging these stream operations, developers can perform various manipulations on data with ease, leading to more maintainable and efficient code.
By understanding how to handle SQL clauses in Java 8 streams, developers can unlock the full potential of functional programming and streamline their data processing tasks with ease.
To delve deeper into the topic and explore more advanced stream operations, check out this detailed guide on Java 8 Stream API.