Overcoming Hibernate 3-Spring Integration Challenges
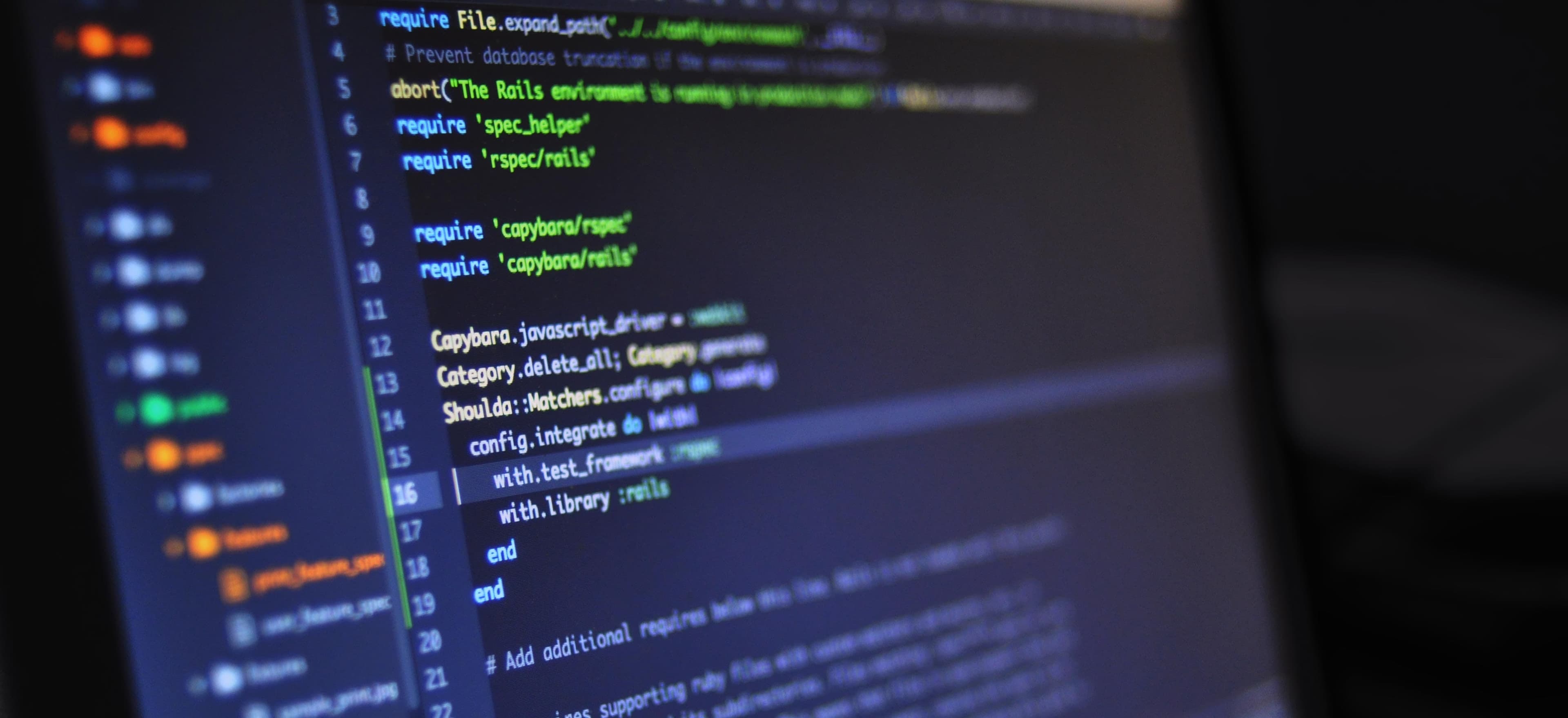
- Published on
Overcoming Hibernate 3-Spring Integration Challenges
When it comes to building robust, enterprise-scale Java applications, the combination of Hibernate 3 and Spring framework has been a popular choice for quite some time. However, integrating these two powerhouses can sometimes be a challenging task, especially when dealing with Hibernate 3-Spring compatibility issues. In this article, we will explore some common challenges developers face when integrating Hibernate 3 with Spring and discuss effective strategies to overcome them.
Understanding the Challenges
Outdated Configurations
One of the primary challenges developers encounter when integrating Hibernate 3 with Spring is dealing with outdated configurations. Hibernate 3 relies on XML-based configurations, whereas modern Spring projects embrace Java-based configurations. This mismatch can lead to conflicts and inconsistencies in the application setup.
Transaction Management
Another common challenge is managing transactions effectively. While both Hibernate 3 and Spring provide transaction management capabilities, aligning them to work seamlessly together requires a deep understanding of their underlying mechanisms.
Session Management
Hibernate utilizes the concept of a SessionFactory to manage database connections, while Spring manages its own contexts and scopes. Integrating these two different mechanisms can result in intricate session management challenges.
Now that we've identified the challenges, let's delve into the strategies to overcome them.
Overcoming the Challenges
1. Updating Configuration to Spring Style
To address the issue of outdated configurations, it's crucial to update the Hibernate 3 configurations to align with the modern Spring style. This involves migrating from XML-based configurations to Java-based configurations. By utilizing Spring's LocalSessionFactoryBean
, we can define Hibernate 3 session factory as a Spring bean, allowing for seamless integration within the Spring application context.
Example:
@Configuration
@EnableTransactionManagement
public class HibernateConfig {
@Bean
public LocalSessionFactoryBean sessionFactory() {
LocalSessionFactoryBean sessionFactory = new LocalSessionFactoryBean();
sessionFactory.setDataSource(dataSource());
sessionFactory.setPackagesToScan("com.example.models");
sessionFactory.setHibernateProperties(hibernateProperties());
return sessionFactory;
}
// Other bean declarations and configuration methods
}
In the example above, the LocalSessionFactoryBean
from the org.springframework.orm.hibernate3.LocalSessionFactoryBean
package is used to define the Hibernate 3 session factory as a Spring bean. This facilitates an updated, Spring-friendly configuration for Hibernate 3.
2. Employing Spring's Declarative Transaction Management
When it comes to transaction management, leveraging Spring's declarative transaction management capabilities can streamline the integration of Hibernate 3 with Spring. Spring's @Transactional
annotation provides a clean and elegant way to manage transactions, allowing for fine-grained control over transaction boundaries.
Example:
@Service
public class ProductService {
@Autowired
private ProductDAO productDAO;
@Transactional
public void saveProduct(Product product) {
productDAO.save(product);
}
// Other business logic methods
}
In this example, the @Transactional
annotation is used to mark the saveProduct
method for transaction management. This enables seamless integration of Hibernate 3 transactions with Spring's declarative transaction management.
3. Integrating Session Management
To address the challenges related to session management, leveraging Spring's OpenSessionInViewFilter
can prove to be beneficial. This filter ensures that a Hibernate session is bound to the thread for the entire processing of the request, thereby simplifying session management in the context of Spring's web MVC framework.
Example (web.xml):
<filter>
<filter-name>hibernateFilter</filter-name>
<filter-class>org.springframework.orm.hibernate3.support.OpenSessionInViewFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>hibernateFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
In the example above, the OpenSessionInViewFilter
is configured in the web.xml
file to ensure seamless session management when using Hibernate 3 with Spring's web MVC framework.
Final Thoughts
Integrating Hibernate 3 with Spring certainly presents its challenges, but with a solid understanding of modern Spring configurations, declarative transaction management, and effective session management strategies, these challenges can be overcome. By updating configurations to align with Spring's style, harnessing Spring's transaction management capabilities, and integrating session management effectively, developers can ensure a smooth and cohesive integration of Hibernate 3 with the Spring framework.
In conclusion, effectively integrating Hibernate 3 with Spring requires adapting to modern Spring configurations, leveraging Spring's transaction management capabilities, and implementing strategies for seamless session management. With the provided strategies and examples, developers can overcome the challenges and build robust, well-integrated applications. For more in-depth insights into Hibernate 3 and Spring integration, refer to the official Spring documentation and Hibernate community resources.
Checkout our other articles