Mastering Microservices: Docker & Kubernetes at Devoxx 2015
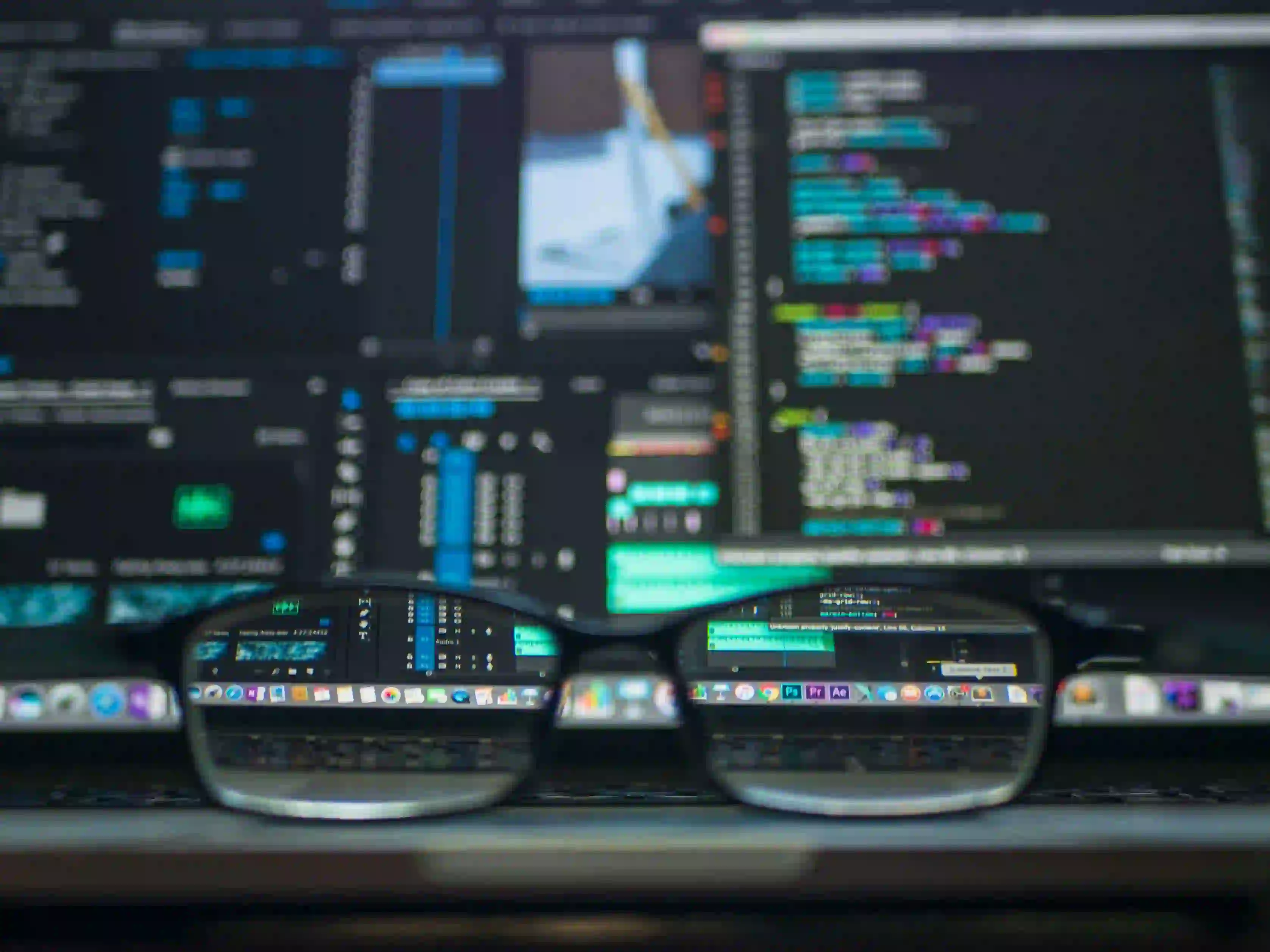
Mastering Microservices with Java, Docker, and Kubernetes
Microservices architecture has become increasingly popular in recent years due to its flexibility, scalability, and resilience. This approach allows developers to build and maintain large, complex applications by breaking them down into smaller, independent services. Java has been a popular language of choice for building microservices due to its robustness and scalability. In this blog post, we'll explore how to master microservices with Java, Docker, and Kubernetes.
What are Microservices?
Microservices are an architectural style that structures an application as a collection of loosely coupled services. Each service is highly maintainable and testable, and is organized around specific business capabilities. These services can be deployed independently, and communicate with each other through well-defined APIs. This approach enables organizations to break down the complexity of monolithic applications into smaller, manageable services.
Java for Microservices
Java has been a preferred language for building microservices due to its strong ecosystem, extensive libraries, and mature frameworks. Frameworks like Spring Boot, Dropwizard, and Micronaut provide powerful tools for developing and deploying microservices with Java.
// Example of a simple microservice using Spring Boot
@RestController
public class HelloController {
@GetMapping("/hello")
public String hello() {
return "Hello, Microservices!";
}
}
In the example above, we have a simple RESTful endpoint created using Spring Boot, a popular Java framework for building microservices. This simple service responds with "Hello, Microservices!" when accessed.
Docker for Microservices
Docker has revolutionized the way we build, ship, and run applications. It allows developers to package applications and their dependencies into containers, providing consistency across different environments. This is particularly useful in a microservices architecture where each service can be encapsulated within its own container.
# Example Dockerfile for a Java microservice
FROM openjdk:11-jre-slim
COPY target/my-application.jar /app/
CMD ["java", "-jar", "/app/my-application.jar"]
The Dockerfile above demonstrates how we can containerize a Java microservice. The openjdk
image is used as the base image, and the application JAR file is copied into the container. When the container is run, the application is executed using the java
command.
Kubernetes for Microservices Orchestration
Kubernetes is an open-source platform designed to automate deploying, scaling, and operating application containers. It provides powerful features for managing containerized applications in a clustered environment.
# Example Kubernetes Deployment for a Java microservice
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-application
spec:
replicas: 3
selector:
matchLabels:
app: my-application
template:
metadata:
labels:
app: my-application
spec:
containers:
- name: my-application
image: my-application:latest
ports:
- containerPort: 8080
In the Kubernetes Deployment configuration above, we define a deployment for our Java microservice. We specify the number of replicas, container image, and ports to expose. Kubernetes will ensure that the specified number of replicas are running and manage their lifecycle.
Mastering Microservices at Devoxx 2015
The Devoxx conference in 2015 featured insightful talks and workshops on mastering microservices with Java, Docker, and Kubernetes. Renowned experts in the field provided in-depth knowledge and practical guidance on building and deploying microservices in a production environment.
The sessions covered topics such as:
- Building resilient and scalable microservices with Java
- Containerizing Java applications with Docker
- Orchestration and scaling with Kubernetes
- Best practices for microservices architecture
The conference served as a platform for developers to dive deep into the world of microservices and gain valuable insights from industry leaders.
The Closing Argument
Mastering microservices with Java, Docker, and Kubernetes opens up a world of possibilities for building scalable and resilient applications. Java's robustness, Docker's containerization, and Kubernetes' orchestration capabilities make for a powerful combination in the world of microservices architecture. By adopting these technologies and best practices, developers can confidently navigate the complexities of modern application development.
Whether you're a seasoned developer or just starting out, mastering microservices with Java, Docker, and Kubernetes will empower you to architect and build sophisticated, scalable applications that meet the demands of today's dynamic business landscape.
So, what are you waiting for? Dive into the world of microservices and unleash the full potential of your Java applications.
For further reading and resources, consider exploring the following:
- Spring Boot - for building production-grade Spring-based applications
- Docker Documentation - for comprehensive guides and tutorials on Docker
- Kubernetes Official Documentation - for detailed information on Kubernetes features and concepts
Are you ready to master microservices with Java, Docker, and Kubernetes? Let's embark on this exciting journey together!