Solving Common Pitfalls in Thymeleaf-Spring Integration
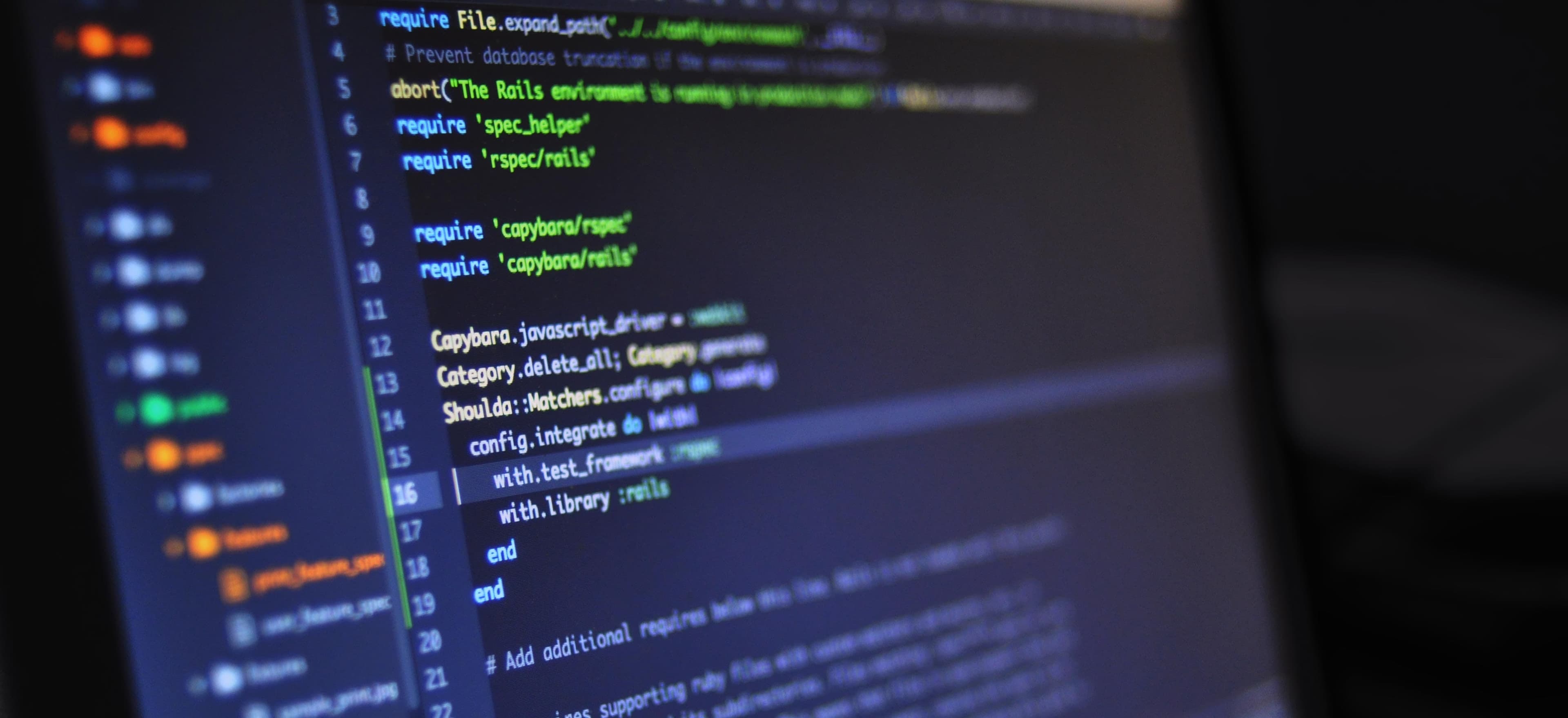
- Published on
Solving Common Pitfalls in Thymeleaf-Spring Integration
Thymeleaf is a popular Java template engine for web applications, while Spring is a widely-used application framework. Integrating Thymeleaf with Spring is a powerful way to create dynamic and robust web applications. However, like any technology stack, there are common pitfalls that developers may encounter when using these tools together. In this blog post, we will explore and provide solutions for some of the most common pitfalls in Thymeleaf-Spring integration, allowing you to harness the full potential of these tools while avoiding potential roadblocks.
1. Resource Handling Issues
One of the common pitfalls in Thymeleaf-Spring integration is resource handling. When using Thymeleaf templates with Spring, it's crucial to ensure that static resources like CSS, JavaScript, and images are properly handled and served by the application.
Solution:
In Spring, static resources are typically served from the /static
or /public
directory in the classpath. To ensure that Thymeleaf templates can access these resources correctly, we need to configure resource handling in the Spring configuration.
@Configuration
@EnableWebMvc
public class MvcConfig implements WebMvcConfigurer {
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry) {
registry.addResourceHandler("/resources/**")
.addResourceLocations("/static/", "classpath:/META-INF/resources/");
}
}
In the above code snippet, we configure the resource handler to serve static resources from the /static
directory. This ensures that when Thymeleaf templates reference static resources, Spring can correctly serve them.
2. Unresolved Variable Error in Thymeleaf Templates
Another common issue in Thymeleaf-Spring integration is encountering unresolved variable errors in Thymeleaf templates, especially when passing model attributes from Spring controllers to the templates.
Solution:
To resolve unresolved variable errors, ensure that the model attributes are correctly passed from the Spring controller to the Thymeleaf template. For example, in a Spring MVC controller, you can add attributes to the model using addAttribute
and then access them in the Thymeleaf template using the Thymeleaf expression language.
@Controller
public class MyController {
@GetMapping("/home")
public String homePage(Model model) {
model.addAttribute("message", "Welcome to the home page");
return "home";
}
}
In the corresponding Thymeleaf template:
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Home Page</title>
</head>
<body>
<h1 th:text="${message}"></h1>
</body>
</html>
In the above example, the message
attribute from the model is accessed in the Thymeleaf template using ${message}
.
3. Thymeleaf Layout Dialect Configuration
Integrating Thymeleaf layout dialect with Spring often leads to configuration issues, particularly when defining the layout structure and allowing different templates to be inserted into specific layout regions.
Solution:
To address Thymeleaf layout configuration issues, it's important to properly configure and use the Thymeleaf layout dialect. This dialect allows for defining a consistent layout structure across multiple pages.
First, ensure that the Thymeleaf layout dialect dependency is included in the project's configuration.
<dependency>
<groupId>nz.net.ultraq.thymeleaf</groupId>
<artifactId>thymeleaf-layout-dialect</artifactId>
<version>2.6.1</version>
</dependency>
After including the dependency, the layout dialect needs to be configured in the Thymeleaf template engine.
@Configuration
public class ThymeleafConfig implements ITemplateResolver {
@Bean
public LayoutDialect layoutDialect() {
return new LayoutDialect();
}
}
By using the Thymeleaf layout dialect, you can define a base layout and insert specific content from different templates into designated layout regions. This improves code reusability and ensures a consistent look and feel across the application.
4. Internationalization and Localization
In internationalized web applications, providing multilingual support is essential. However, when integrating Thymeleaf with Spring, developers may encounter challenges in managing internationalization and localization features.
Solution:
To enable internationalization and localization in a Thymeleaf-Spring application, use Spring's MessageSource
to resolve messages in the Thymeleaf templates.
First, define message properties for different languages, such as messages_en.properties
for English and messages_fr.properties
for French.
# messages_en.properties
greeting=Hello!
# messages_fr.properties
greeting=Bonjour!
Configure the message source bean in the Spring configuration:
@Configuration
public class AppConfig implements WebMvcConfigurer {
@Bean
public MessageSource messageSource() {
ResourceBundleMessageSource messageSource = new ResourceBundleMessageSource();
messageSource.setBasename("messages");
messageSource.setDefaultEncoding("UTF-8");
return messageSource;
}
// Other configuration...
}
In the Thymeleaf template, use the #{}
syntax to reference the messages:
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Internationalized Page</title>
</head>
<body>
<p th:text="#{greeting}"></p>
</body>
</html>
By following these steps, Thymeleaf can retrieve the appropriate message based on the user's locale, providing seamless internationalization and localization support.
Key Takeaways
In this blog post, we have explored and addressed some common pitfalls in Thymeleaf-Spring integration, providing solutions to ensure a smoother development experience. By effectively handling static resources, resolving variable errors, configuring the Thymeleaf layout dialect, and enabling internationalization and localization, developers can strengthen their Thymeleaf-Spring applications and deliver a more polished user experience.
By implementing the provided solutions, developers can harness the full capabilities of Thymeleaf and Spring, avoiding common pitfalls and building robust and dynamic web applications with ease.
Remember, thorough understanding and effective utilization of these solutions can contribute to the success of your Thymeleaf-Spring projects. Happy coding!
Resources:
- Thymeleaf Documentation
- Spring Framework Reference Documentation