Mastering Java Sorting: One Common Pitfall to Avoid
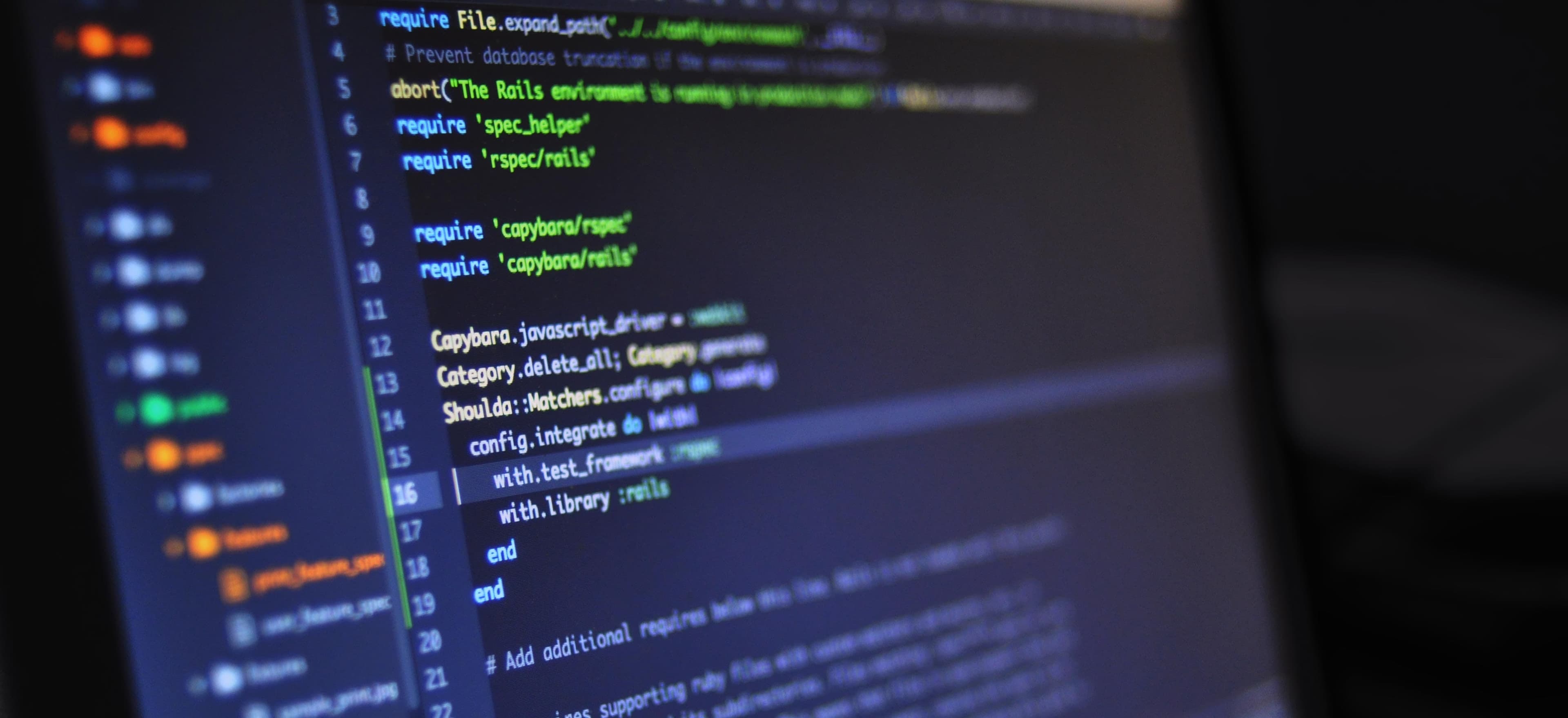
- Published on
Mastering Java Sorting: One Common Pitfall to Avoid
When it comes to mastering Java, sorting is an indispensable skill for any developer. Whether you are dealing with simple arrays or complex collections, efficient sorting algorithms are crucial for optimal performance. In this article, we will delve into the nuances of sorting in Java, highlight a common pitfall to avoid, and provide actionable tips to refine your sorting proficiency.
The Significance of Sorting Algorithms
Sorting algorithms play a pivotal role in organizing data in a meaningful and efficient manner. In Java, the Arrays.sort()
method and the Collections.sort()
method provide convenient ways to sort arrays and collections, respectively. These methods internally use the dual-pivot Quicksort algorithm, which offers excellent average-case performance and is well-suited for most scenarios.
The Pitfall: Not Implementing Comparable or Comparator
One common pitfall that developers encounter when sorting custom objects in Java is failing to implement the Comparable
interface or provide a custom Comparator
. When an object needs to be sorted, whether it's an array or a collection, Java requires the elements to have a natural ordering. This is achieved by implementing the Comparable
interface and overriding the compareTo()
method, or by providing a separate class that implements the Comparator
interface with a custom comparison logic.
Let's take a closer look at the Comparable
interface. Consider the following Person
class representing individuals with a name
and age
:
public class Person implements Comparable<Person> {
private String name;
private int age;
// Constructors, getters, setters
@Override
public int compareTo(Person otherPerson) {
return this.age - otherPerson.age;
}
}
In this example, the Person
class implements the Comparable
interface and provides a natural ordering based on the age
attribute. This enables sorting of Person
objects using the default sorting logic, such as in Arrays.sort()
or Collections.sort()
.
However, situations may arise where the default sorting behavior is not suitable. This is where a custom Comparator
comes into play. It allows for flexibility in defining multiple sorting strategies without modifying the target object. Here's a demonstration using a NameComparator
to sort Person
objects based on their names:
public class NameComparator implements Comparator<Person> {
@Override
public int compare(Person person1, Person person2) {
return person1.getName().compareTo(person2.getName());
}
}
By providing custom sorting logic through the Comparator
interface, developers can sort objects based on different attributes or even custom rules, offering a versatile approach to sorting in Java.
Best Practices for Effective Sorting
To ensure seamless and efficient sorting in Java, it is essential to adopt best practices that align with industry standards and optimize performance. Here are some key considerations to elevate your sorting proficiency:
1. Choose the Right Sorting Algorithm
While Java's built-in sorting methods leverage the Quicksort algorithm, it's important to evaluate the nature of the data being sorted and consider alternative algorithms when dealing with specific use cases. For instance, when sorting small arrays or nearly sorted collections, the Arrays.sort()
method can intelligently switch to a different algorithm, such as the Insertion sort, for improved efficiency.
2. Employ Comparator Chaining
In scenarios where multiple attributes need to be considered for sorting, employing comparator chaining can simplify the sorting process. By using the thenComparing()
method available in the Comparator
interface, developers can chain multiple comparators to establish a composite sorting order based on various attributes.
3. Leverage Parallel Sorting
With the evolution of multi-core processors, Java provides a parallel sorting capability through the Arrays.parallelSort()
method. This enables parallelization of the sorting process for large arrays, leveraging the available hardware resources to expedite the sorting procedure.
4. Optimize for Stability
Stability in sorting ensures that the relative order of equal elements remains unchanged after sorting. When using the built-in sorting methods, such as Arrays.sort()
or Collections.sort()
, it's important to be aware of their inherent stability to guarantee predictable outcomes, especially in scenarios where preserving the original order of equivalent elements is vital.
Conclusion
Mastering sorting in Java is fundamental for harnessing the full potential of the language's capabilities. By avoiding the common pitfall of overlooking the implementation of Comparable
or Comparator
interfaces, and incorporating best practices such as selecting the appropriate sorting algorithm and leveraging advanced sorting features, developers can elevate their proficiency in sorting data effectively and efficiently. With a robust understanding of sorting algorithms and the agility to tailor sorting behavior, Java developers can navigate complex data structures with ease, underpinning the foundation of reliable and high-performance applications.
We hope this article has provided valuable insights into the nuances of sorting in Java and equipped you with actionable strategies to refine your sorting techniques. Happy coding!
Ready to dive deeper into Java Sorting? Check out our comprehensive guide on Sorting in Java for a detailed exploration of sorting techniques and best practices.