Mastering Play 2: Crafting Your First Module!
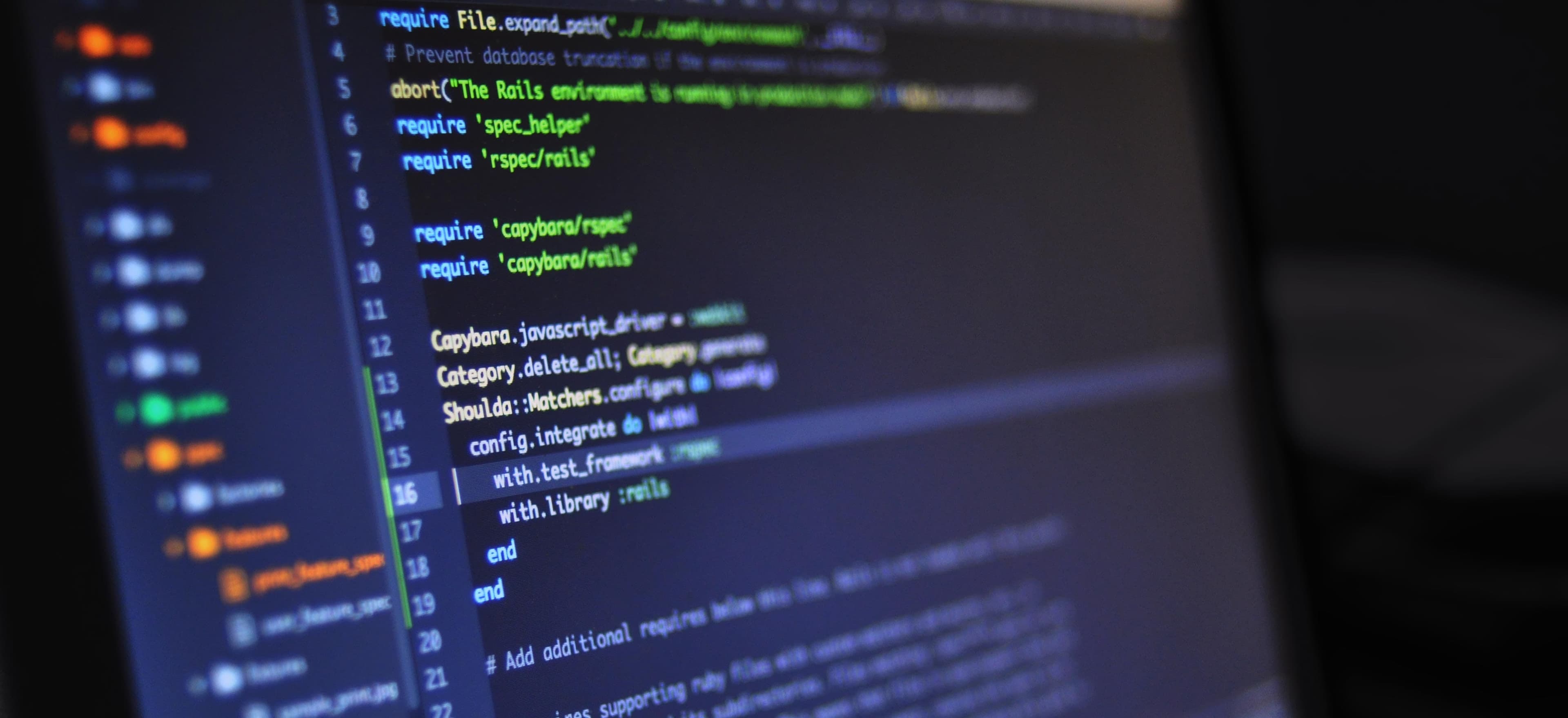
- Published on
Mastering Play 2: Crafting Your First Module
In the realm of Java web application development, Play Framework stands out as a versatile and powerful tool for crafting scalable, high-performance web applications. One of the key aspects of Play Framework is its modularity, which allows developers to create and utilize modules to enhance their projects' functionality. In this guide, we will delve into the process of creating your first Play 2 module using Java, empowering you to extend the capabilities of your Play applications with ease.
Understanding Play 2 Modules
Play 2 modules are self-contained packages that encapsulate a specific set of features or functionalities, enabling easy integration with Play applications. By creating custom modules, developers can efficiently reuse code across multiple projects, enhance code organization, and promote maintainability. Furthermore, modules can be shared with the Play community, contributing to the ecosystem of reusable components.
Setting the Stage
Before diving headfirst into crafting a Play 2 module, it's crucial to have a solid understanding of Play Framework's fundamental concepts, such as controllers, routes, and views. If you're new to Play Framework or need a refresher, it's recommended to familiarize yourself with its core components and request handling mechanisms to effectively build and integrate modules.
Creating a Play 2 Module
Let's embark on the journey of creating a custom Play 2 module step by step. For the purpose of this guide, we'll craft a simple module for generating random quotes.
-
Project Structure:
In your development environment, create a new Play 2 project or navigate to an existing Play project where you intend to integrate the module.
-
Module Creation:
Within the project, create a new directory named
quotes-module
to house the module's source code. -
Module Initialization:
Inside the
quotes-module
directory, initialize the module by creating aQuotesModule.java
file. This serves as the entry point of the module and extends theplay.api.Module
class.package quotes.module; import com.google.inject.AbstractModule; public class QuotesModule extends AbstractModule { @Override protected void configure() { // Module configuration } }
The
configure
method provides the foundation for configuring the bindings and dependencies within the module. -
Defining Module Functionality:
Create a class within the module to encapsulate the functionality of generating random quotes. Let's name the class
QuoteService
.package quotes.module; public class QuoteService { public String generateQuote() { // Logic to generate a random quote return "To be, or not to be, that is the question."; } }
Integrating the Module
With the module created, the next step is to integrate it into a Play 2 application.
-
Module Dependency:
Update the
build.sbt
file of the Play application to include the module as a dependency.lazy val root = (project in file(".")).enablePlugins(PlayJava) libraryDependencies += "quotes-module" % "quotes-module_2.12" % "1.0"
-
Utilizing the Module:
Within a controller of the Play application, inject an instance of the
QuoteService
from the module and utilize its functionality.package controllers; import play.mvc.Controller; import play.mvc.Result; import quotes.module.QuoteService; import javax.inject.Inject; public class HomeController extends Controller { private QuoteService quoteService; @Inject public HomeController(QuoteService quoteService) { this.quoteService = quoteService; } public Result index() { String randomQuote = quoteService.generateQuote(); return ok(randomQuote); } }
Lessons Learned
Congratulations! You've successfully crafted your first Play 2 module using Java and integrated it into a Play application. This newfound capability opens the doors to creating and leveraging reusable components across your projects, enhancing productivity and code maintainability. By grasping the essence of Play 2 modules, you pave the way for building modular, extensible, and efficient web applications in the Java ecosystem.
In this blog post, we covered the basics of creating a Play 2 module, integrating it into a Play application, and gaining an understanding of the benefits of modularization. As you continue your journey with Play Framework, delve deeper into advanced module development, explore community-contributed modules, and unlock the full potential of Play's modularity.
For additional insights into Play 2 and Java web development, refer to the official Play Framework documentation and Java web application development best practices.
Stay tuned for more guides and tutorials on mastering Play 2 and maximizing the potential of Java web development!