Untangling Neo4j: Solving Dependency Graph Challenges
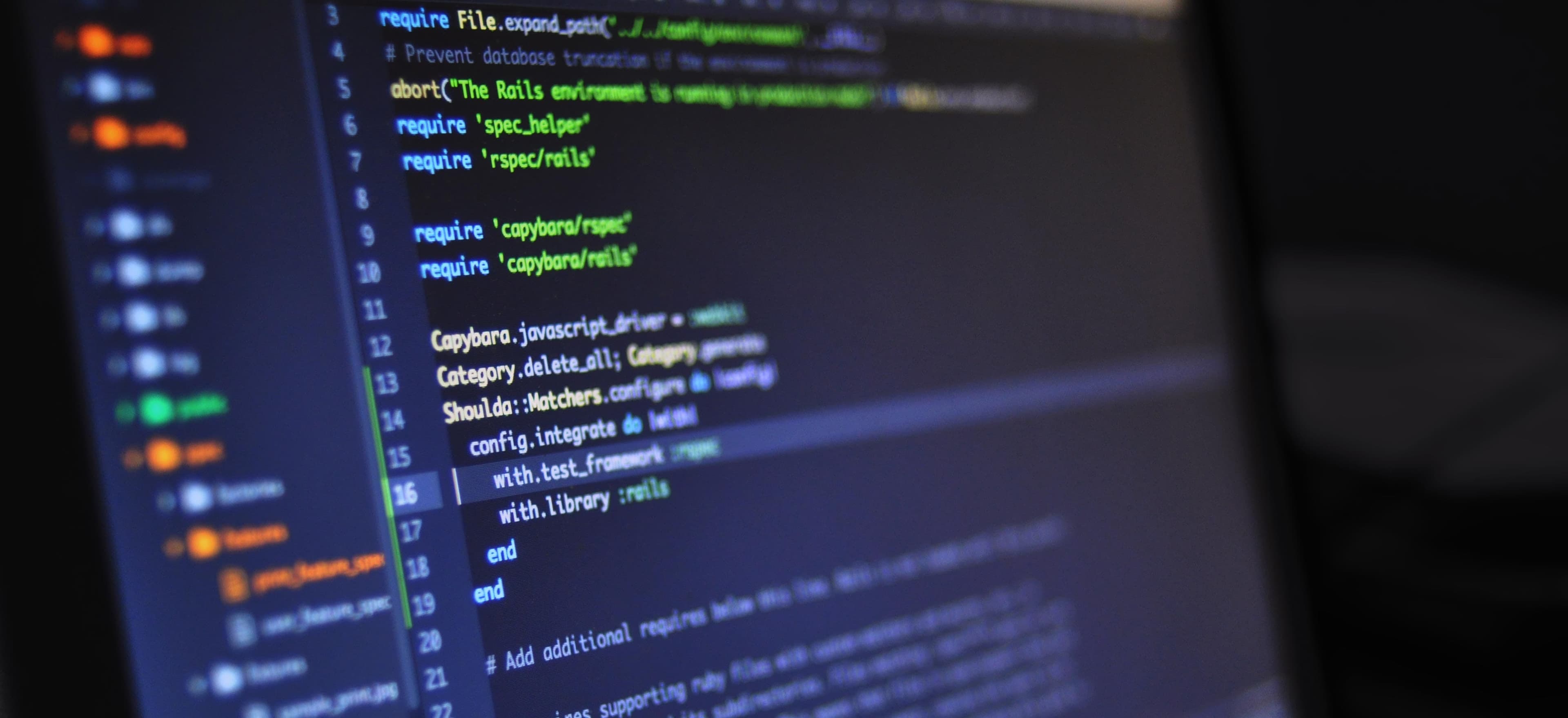
- Published on
Untangling Neo4j: Solving Dependency Graph Challenges
Managing dependencies in software development is crucial for ensuring smooth operations and efficient performance. One of the ways to visualize and analyze dependencies is by using a graph database like Neo4j. In this article, we will explore the challenges posed by dependency graphs and how Neo4j can be leveraged to address them effectively in Java applications.
Understanding Dependency Graphs
Dependency graphs are essential for representing complex relationships between elements in a system. In software development, these elements can range from packages, modules, or classes to external libraries and frameworks. Visualizing these dependencies as a graph helps in understanding the impact of changes, identifying cyclic dependencies, and optimizing system architecture.
Consider a scenario where a Java application depends on multiple libraries, and each of these libraries has its own set of dependencies. The interplay of these dependencies forms a complex web that demands careful management. Traditional relational databases might not be the best fit for representing and querying such intricate relationships, which is where graph databases come into play.
Neo4j as a Solution
Neo4j, a popular graph database, is well-suited for handling dependency graphs. Its native graph storage and traversal capabilities make it an ideal choice for modeling complex relationships. Using Cypher, Neo4j's query language, developers can leverage powerful graph algorithms to extract valuable insights from the dependency graph.
In Java applications, the Neo4j Java driver provides a robust interface for interacting with the Neo4j database. With its support for various types of queries and transactions, the Java driver empowers developers to seamlessly integrate Neo4j into their applications.
Challenges and Solutions
Challenge 1: Modeling Dependencies
Solution: Utilize Neo4j's node and relationship structure to model dependencies as nodes and edges in the graph. For instance, a Java package can be represented as a node, and its dependencies can be depicted as relationships between nodes.
// Example of creating a node representing a Java package
try (Session session = driver.session()) {
session.run("CREATE (pkg:Package {name: 'com.example.package'})");
}
Challenge 2: Querying Transitive Dependencies
Solution: Leverage Neo4j's graph traversal algorithms to efficiently query for transitive dependencies. By using algorithms like breadth-first search or shortest path, developers can retrieve all transitive dependencies for a given element in the graph.
// Example of querying transitive dependencies using Cypher
try (Session session = driver.session()) {
String packageName = "com.example.package";
Result result = session.run(
"MATCH (pkg:Package {name: $packageName})-[:DEPENDS_ON*]->(dependent:Package) RETURN dependent",
parameters("packageName", packageName)
);
}
Challenge 3: Detecting Cyclic Dependencies
Solution: Neo4j's graph algorithms can be employed to detect cycles within the dependency graph. By identifying cyclic dependencies, developers can refactor the codebase to remove such dependencies and improve the overall system stability.
// Example of using a cycle detection algorithm in Neo4j
try (Session session = driver.session()) {
Result result = session.run("CALL algo.cycles()");
}
Challenge 4: Analyzing Impact of Dependency Changes
Solution: With Neo4j, developers can traverse the graph to analyze the impact of modifying dependencies. This can help in understanding how a change in one part of the system ripples through the entire graph, enabling proactive decision-making.
// Example of analyzing the impact of dependency changes
try (Session session = driver.session()) {
String updatedDependency = "new.dependency";
Result result = session.run(
"MATCH (pkg:Package)-[:DEPENDS_ON]->(dependency:Package {name: $updatedDependency}) RETURN pkg",
parameters("updatedDependency", updatedDependency)
);
}
The Last Word
Neo4j provides a robust solution for addressing the challenges posed by dependency graphs in Java applications. By utilizing its graph modeling capabilities and powerful traversal algorithms, developers can gain valuable insights into their system's dependencies and make informed decisions. Integrating Neo4j into Java applications empowers developers to navigate through the intricacies of dependency management with ease, ultimately leading to more resilient and maintainable software systems.
In the ever-evolving landscape of software development, effective dependency management is a cornerstone for building robust and scalable applications. With Neo4j, developers can confidently tackle the complexities of dependency graphs and steer their projects towards success.
For further exploration, consider delving into the Neo4j documentation and the Neo4j Java driver documentation. These resources offer comprehensive insights into leveraging Neo4j for Java applications and harnessing the power of graph databases for efficient dependency management.