Tackling Common Pitfalls in Grails Spring Security
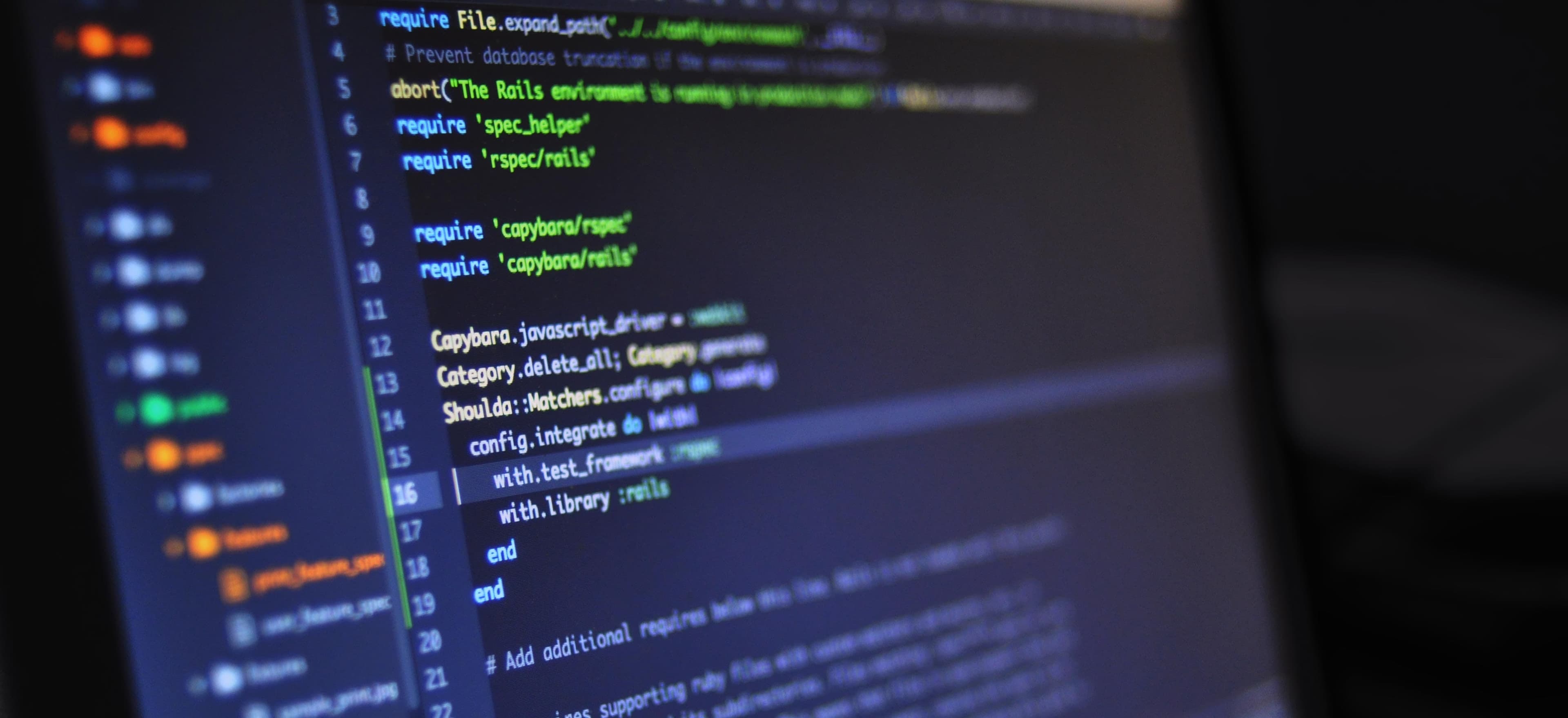
- Published on
Tackling Common Pitfalls in Grails Spring Security
Spring Security is a powerful framework that ensures the security aspects of your Grails applications. While offering robust authentication, authorization, and protection against common vulnerabilities, it can sometimes be daunting to implement correctly. Developers often face hurdles when integrating Spring Security with Grails, leading to configurations that are less secure than intended. In this post, we'll explore some common pitfalls in Grails Spring Security and how to avoid them, ensuring your application is both secure and efficient.
Overlooking Default Settings
One of the most common mistakes is not thoroughly reviewing and understanding the default settings of Spring Security. It's crucial to remember that the defaults might not align with the security requirements of your specific application.
Example:
Consider the default password storage in Spring Security. Initially, it used a simple hashing technique, which is no longer recommended due to advancements in attack tools. Now, BCrypt is the default, which is a significant improvement. However, developers often override this with weaker options due to misunderstanding or legacy compatibility reasons.
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
@Bean
public BCryptPasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
Why? The above code snippet ensures you are using BCryptPasswordEncoder, which is a strong hashing function for storing passwords securely. It's essential for safeguarding user credentials against brute-force attacks.
Not Using HTTPS
A grave mistake is not enforcing HTTPS, especially for authentication pages and endpoints. HTTPS encrypts the data between the client and server, protecting it from man-in-the-middle attacks.
Example:
To enforce HTTPS in Grails Spring Security, you can configure the channel security in your application.groovy
or application.yml
.
grails:
plugin:
springsecurity:
portMapper:
httpPort: 8080
httpsPort: 8443
ssl:
requireSecureChannel: true
Why? This configuration ensures that any HTTP request is automatically redirected to HTTPS, securing data transmission. Particularly for authentication and sensitive operations, using HTTPS is non-negotiable.
Improper Session Management
Improper session management can lead to security vulnerabilities, such as session fixation or hijacking. It's vital to configure sessions correctly, utilizing Spring Security's capabilities.
Example:
To prevent session fixation attacks, you can configure Spring Security to migrate the session after login.
grails:
plugin:
springsecurity:
sessionFixationPrevention: migrateSession
Why? This setting ensures that a new session is created upon authentication, making it harder for attackers to hijack an existing session.
Neglecting CSRF Protection
Cross-Site Request Forgery (CSRF) is an attack where a malicious site causes a user's browser to perform an unwanted action on another site where the user is authenticated. Spring Security provides CSRF protection by default, but it's often mistakenly disabled.
Example:
Ensure CSRF protection is enabled by checking your configuration. It should be explicitly enabled if not already by default.
grails:
plugin:
springsecurity:
csrf:
enabled: true
Why? Keeping CSRF protection enabled protects your application from malicious sites that might attempt to perform actions on behalf of logged-in users.
Exposing Sensitive Information in Logs
Logs are invaluable for debugging, but they can inadvertently expose sensitive information if not handled carefully. Ensure that you're not logging sensitive user details, especially passwords or session IDs.
Example:
When configuring loggers, be mindful of the information that could be logged. Adjust the logging levels appropriately and avoid logging sensitive information directly.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class SecureLoggingExample {
private static final Logger log = LoggerFactory.getLogger(SecureLoggingExample.class);
public void logSecureMessage() {
// Log informational messages without exposing sensitive data
log.info("A secure log message.");
}
}
Why? This practice ensures that even if logs are accessed maliciously, the sensitive information is not exposed, maintaining your users' security.
A Final Look
Spring Security is a pivotal component of the Grails ecosystem, providing a comprehensive security framework for your applications. However, its effectiveness is only as good as its implementation. By understanding and avoiding these common pitfalls, developers can ensure that their applications are secure, robust, and resilient against common vulnerabilities.
Further reading and resources are crucial for staying up-to-date with security practices. The official Spring Security documentation is a great starting point. Also, consider exploring resources like the OWASP Cheat Sheet Series for general security best practices.
Remember, security is an ongoing process, not a one-time setup. Regularly review and update your security configurations to adapt to new threats and vulnerabilities. Happy coding, and stay secure!