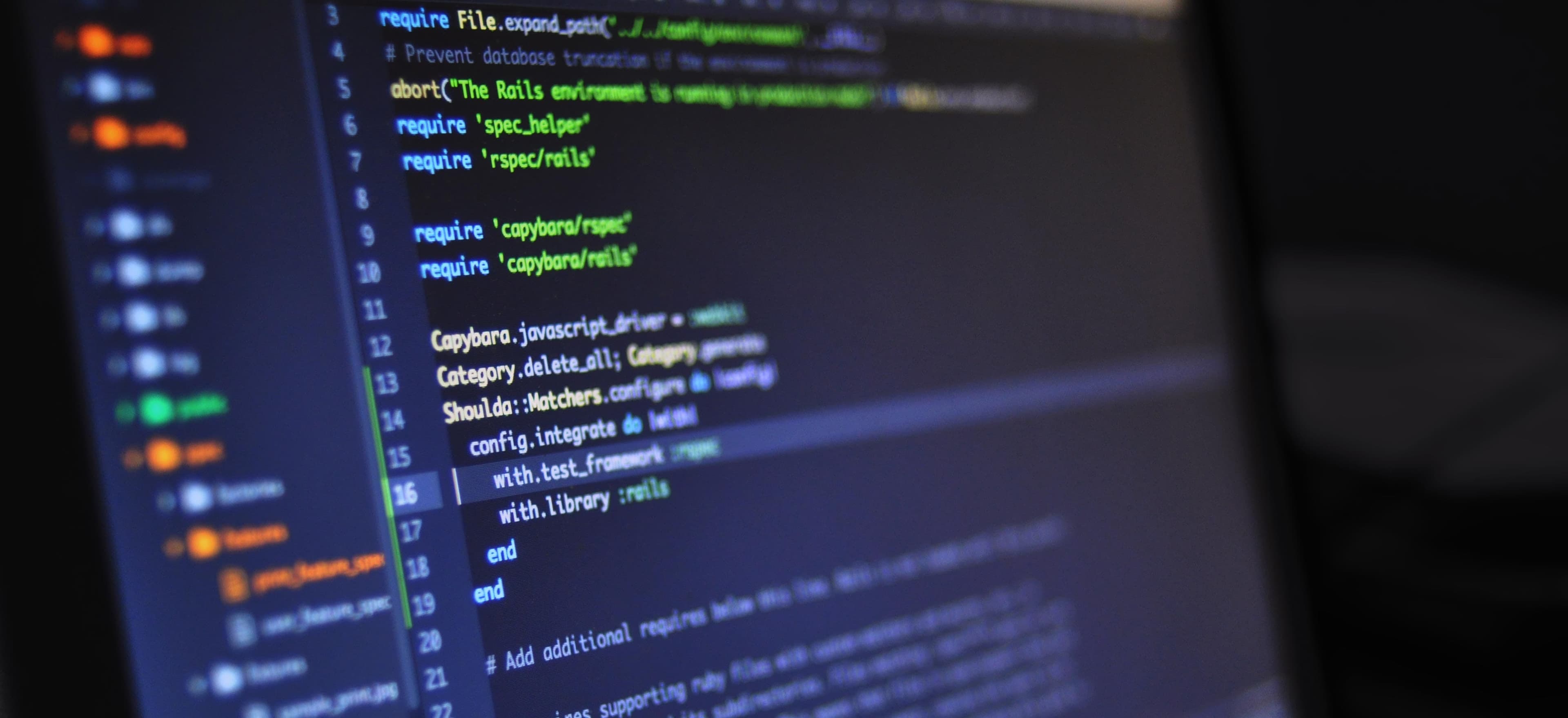
- Published on
Mastering Spring Security: Solving Simple Login Challenges
When it comes to creating secure and reliable web applications, Spring Security is a powerful and versatile tool in the Java ecosystem. In this post, we'll dive into the basics of Spring Security and solve some of the common challenges encountered when implementing a simple login system.
Understanding Spring Security
Spring Security is a framework that focuses on providing authentication, authorization, and protection against common security exploits for Java applications. It integrates seamlessly with the Spring Framework and offers a wide range of features for securing your application.
Setting Up a Simple Spring Boot Project
To get started, let's create a basic Spring Boot project and add the necessary dependencies for Spring Security.
// pom.xml
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
With these dependencies in place, we can move on to defining a simple Spring Security configuration.
Configuring Spring Security
Create a new class to configure Spring Security by extending WebSecurityConfigurerAdapter
and overriding the configure
method.
// SecurityConfig.java
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/public").permitAll()
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.permitAll()
.and()
.logout()
.permitAll();
}
}
In the above configuration, we define that requests to "/public" are permitted for all users, while any other request requires authentication. We also specify a custom login page and enable logout functionality.
Implementing a Simple User Service
Next, let's create a simple user service that provides a hardcoded user for demonstration purposes.
// UserService.java
@Service
public class UserService implements UserDetailsService {
@Override
public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException {
if ("user".equals(username)) {
return User.builder()
.username("user")
.password("{noop}password")
.roles("USER")
.build();
}
throw new UsernameNotFoundException("User not found");
}
}
In the loadUserByUsername
method, we return a user with the username "user" and password "password", along with the role "USER". It's worth noting that we're using the {noop}
prefix to indicate that the password is not encoded.
Creating a Login Page
Now, let's create a simple login page using Thymeleaf to demonstrate the authentication flow.
<!-- login.html -->
<!DOCTYPE html>
<html>
<head>
<title>Login</title>
</head>
<body>
<h2>Login</h2>
<form th:action="@{/login}" method="post">
<div>
<label for="username">Username:</label>
<input type="text" id="username" name="username"/>
</div>
<div>
<label for="password">Password:</label>
<input type="password" id="password" name="password"/>
</div>
<button type="submit">Login</button>
</form>
</body>
</html>
Putting It All Together
Finally, let's connect the pieces we've created by setting up a simple controller to handle the login page and a public endpoint for demonstration.
// HomeController.java
@Controller
public class HomeController {
@GetMapping("/")
public String index() {
return "index";
}
@GetMapping("/public")
public String publicPage() {
return "public";
}
@GetMapping("/login")
public String login() {
return "login";
}
}
In this HomeController
, we define mappings for the home page, the public page, and the login page. These mappings align with the configuration we set up in the SecurityConfig
.
Closing Remarks
In this post, we've covered the basics of setting up a simple login system using Spring Security in a Spring Boot application. While this example is straightforward, it demonstrates the fundamental aspects of authentication and authorization provided by Spring Security.
With a solid understanding of these foundational concepts, you can take your security implementation to the next level by exploring features such as custom authentication providers, OAuth integration, and more.
By mastering Spring Security, you can ensure that your Java web applications are well-protected and equipped to handle modern security challenges.
Now that you have a grasp on these concepts, you can dive deeper into Spring Security documentation and explore advanced topics to reinforce your understanding.
Happy coding!