Boost Your App UI: Mastering Overlay Icons on ImageViews
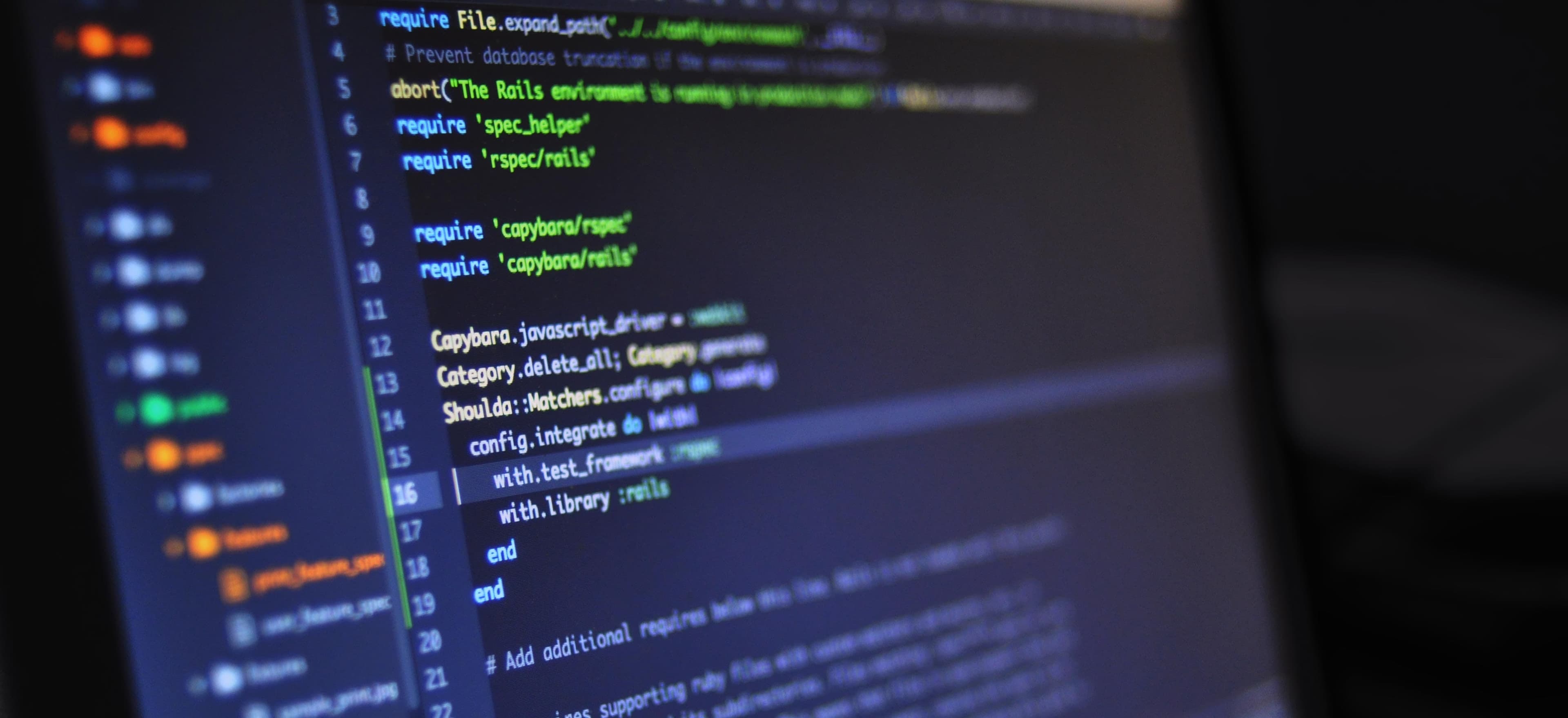
- Published on
Boost Your App UI: Mastering Overlay Icons on ImageViews
As developers, we all strive to create visually appealing user interfaces that are both intuitive and aesthetically pleasing. One way to achieve this is by mastering the art of overlaying icons on ImageViews
in your Android app. This simple yet effective technique can elevate the user experience and make your app stand out. In this blog post, we will delve into the process of adding overlay icons on ImageViews
in Java, offering insights and practical examples to help you master this essential UI skill.
Understanding the Need for Overlay Icons
Before we dive into the implementation, let's understand the significance of overlay icons in app UI. Overlay icons can be used to convey additional information or provide visual cues to users without overcrowding the UI. They are commonly employed to indicate status, offer quick actions, or simply enhance the visual appeal of an app. By adding overlay icons on ImageViews
, you can effectively communicate messages and functionality to users in a clear and concise manner.
Setting Up Your Project
To get started, create a new Android project in Android Studio or open an existing one where you want to implement overlay icons on ImageViews
. Ensure that you have the necessary resources, such as the images and icons you intend to overlay.
Adding Overlay Icons to ImageViews
Step 1: Adding the ImageView and Overlay Icon
First, let's add the ImageView
to your layout XML file. For this example, we will use a simple ImageView
with a background image.
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<ImageView
android:id="@+id/imageView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/background_image" />
</RelativeLayout>
Next, you need to add the overlay icon to the ImageView
. This can be achieved programmatically in your Java code.
Step 2: Programmatically Adding the Overlay Icon
Let's assume we want to add a checkmark icon as an overlay on the ImageView
.
ImageView imageView = findViewById(R.id.imageView);
Drawable overlayIcon = ContextCompat.getDrawable(this, R.drawable.checkmark_icon);
if (overlayIcon != null) {
int width = overlayIcon.getIntrinsicWidth();
int height = overlayIcon.getIntrinsicHeight();
overlayIcon.setBounds(0, 0, width, height);
// Adding the overlay icon
imageView.setCompoundDrawables(null, null, overlayIcon, null);
}
In the above code, we retrieve the ImageView
and the overlay icon as Drawable
objects. We then set the bounds for the overlay icon and use setCompoundDrawables()
to place the overlay on the right side of the ImageView
. This action adds the checkmark icon as an overlay on the ImageView
, effectively combining the background image and the overlay icon.
Customization and Best Practices
Customizing Overlay Icon Position
You can customize the position of the overlay icon by adjusting the bounds and parameters in the setCompoundDrawables()
method. This allows you to control whether the overlay is placed to the left, right, top, or bottom of the ImageView
.
Best Practices for Overlay Icons
When using overlay icons, ensure that they complement the background image and do not overshadow or clutter the UI. Use subtle and meaningful icons that provide value to the user and enhance the overall user experience of the app.
Final Considerations
Mastering the art of overlaying icons on ImageViews
can significantly elevate the user interface of your Android app. By effectively adding overlay icons, you can convey information, provide intuitive visual cues, and enhance the overall aesthetics of your app. With the insights and examples shared in this post, you can confidently integrate overlay icons into your app UI, boosting its appeal and usability.
Start implementing overlay icons on your ImageViews
today and take your app UI to the next level!
To explore more advanced UI techniques, you can delve into Android's official documentation and keep refining your app's user interface.
Checkout our other articles