Boost Performance: Mastering Off-Heap Concurrent Counters!
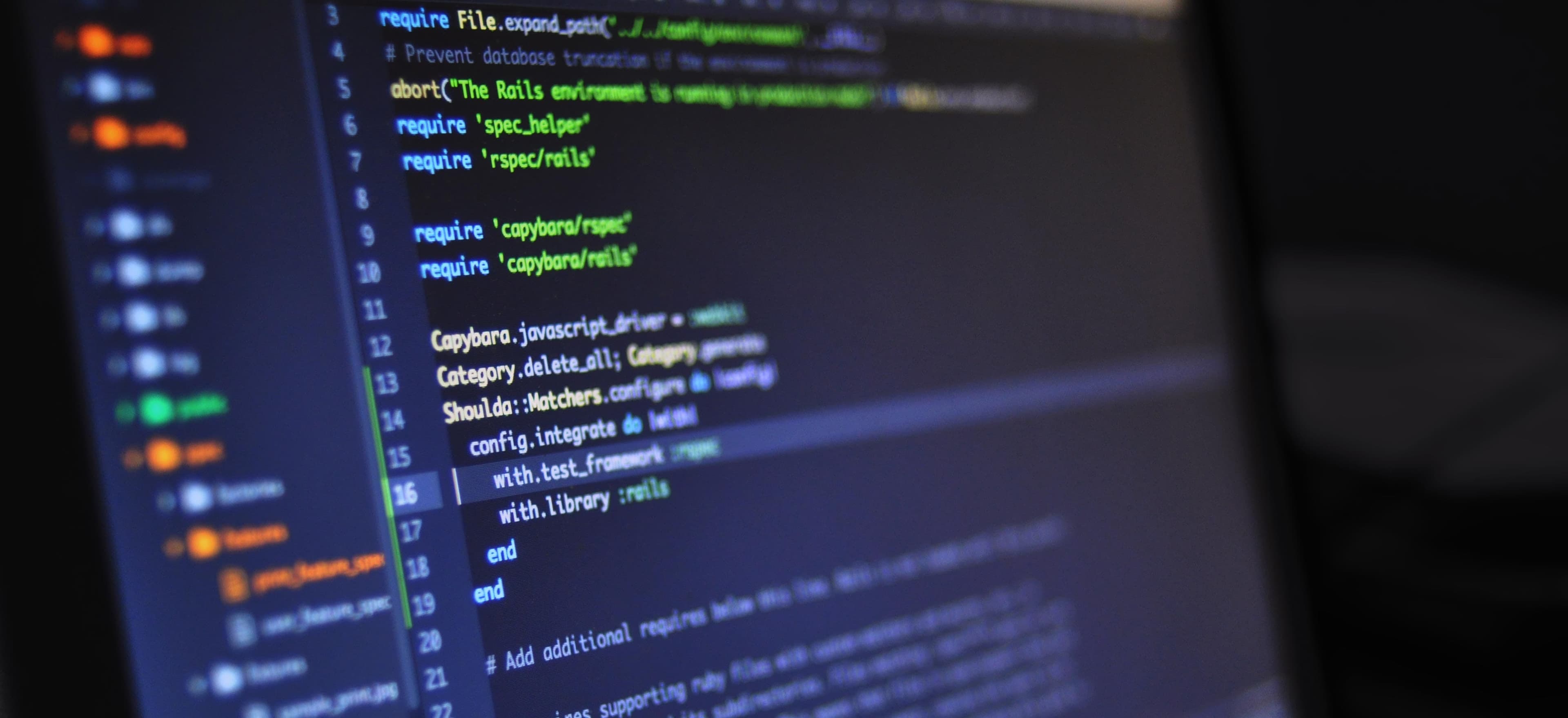
- Published on
Boost Performance: Mastering Off-Heap Concurrent Counters
In Java, managing concurrent access to shared data is a critical aspect of programming. Traditional approaches like using locks or the synchronized
keyword work well, but they come with performance overhead due to context switching and contention. Off-heap memory management offers a promising solution to this problem, allowing for faster and more efficient concurrent data access.
In this article, we'll explore the concept of off-heap memory and delve into the implementation of off-heap concurrent counters in Java. We'll discuss the benefits of off-heap memory, the challenges it poses, and how off-heap concurrent counters can significantly boost performance in Java applications.
Understanding Off-Heap Memory
In Java, the heap is the area of memory where objects are allocated. Accessing heap memory involves the Java Virtual Machine (JVM) and its garbage collection mechanism, which can introduce latency and overhead, especially in concurrent scenarios. Off-heap memory, on the other hand, refers to memory that is allocated outside the heap and is not managed by the JVM's garbage collector.
Off-heap memory is often leveraged for performance-critical data storage and access, as it bypasses the JVM's memory management, allowing for more direct and efficient memory operations. It is particularly useful for scenarios where low latency and predictable memory usage are crucial, such as in high-performance computing, real-time systems, and big data processing.
The Need for Off-Heap Concurrent Counters
Counters play a fundamental role in a wide range of applications, from monitoring system metrics to tracking user interactions. In a concurrent environment, maintaining accurate counters while ensuring efficient access patterns can be challenging. Traditional in-memory counters, especially those guarded by locks or synchronization, can become bottlenecks under high concurrency.
Off-heap concurrent counters address these challenges by providing a mechanism to increment and read counters without contending for locks or relying on expensive synchronization. This approach can significantly improve the scalability and performance of applications that heavily rely on counter operations.
Implementing Off-Heap Concurrent Counters
Let's dive into an example of implementing off-heap concurrent counters in Java using the Chronicle-Map library. Chronicle-Map offers a high-performance, off-heap key-value store with support for concurrent access and provides an excellent foundation for building off-heap concurrent counters.
Setting Up Chronicle-Map
First, we need to include the Chronicle-Map dependency in our project. We can do this by adding the following Maven dependency to our pom.xml
:
<dependency>
<groupId>net.openhft</groupId>
<artifactId>chronicle-map</artifactId>
<version>3.19.16</version>
</dependency>
Creating Off-Heap Concurrent Counters
Now, let's create a simple class for managing off-heap concurrent counters using Chronicle-Map:
import net.openhft.chronicle.map.ChronicleMap;
import java.io.File;
import java.io.IOException;
public class OffHeapCounterManager {
private ChronicleMap<String, Long> counters;
public OffHeapCounterManager(String filePath, long maxEntries) {
try {
counters = ChronicleMap
.of(String.class, Long.class)
.name("off-heap-counters")
.entries(maxEntries)
.createPersistedTo(new File(filePath));
} catch (IOException e) {
// Handle exception
}
}
public void incrementCounter(String key) {
counters.compute(key, (k, v) -> (v == null) ? 1 : v + 1);
}
public long getCounterValue(String key) {
return counters.getOrDefault(key, 0L);
}
public void close() {
counters.close();
}
}
In the OffHeapCounterManager
class, we use Chronicle-Map to create a persistent off-heap map that stores counters with keys of type String
and values of type Long
. We provide methods to increment a counter given a key, retrieve the value of a counter, and close the Chronicle-Map resource gracefully.
Understanding the Implementation
The incrementCounter
method utilizes the compute
function of Chronicle-Map to atomically increment the value associated with the given key. If the key does not exist, it initializes the counter to 1. This atomic update ensures thread safety without the need for explicit synchronization.
The getCounterValue
method simply retrieves the value associated with the specified key from the off-heap map. Similarly, the close
method is responsible for releasing the resources associated with the Chronicle-Map instance when it's no longer needed.
Benefits of Off-Heap Concurrent Counters
The use of off-heap concurrent counters offers several key benefits:
-
Improved Performance: Off-heap memory operations generally have lower latency and reduced contention compared to heap-based operations, leading to improved performance, especially in highly concurrent scenarios.
-
Scalability: Off-heap concurrent counters can efficiently handle a large number of concurrent operations without contention, making them highly scalable for applications with heavy concurrent workloads.
-
Predictable Memory Usage: By bypassing the JVM's garbage collection, off-heap memory helps in reducing memory allocation and deallocation overhead, resulting in more predictable memory usage patterns.
-
Persistent Storage: Off-heap data stored in Chronicle-Map persists across application restarts, making it suitable for scenarios where data durability is essential.
Considerations and Best Practices
While off-heap concurrent counters offer significant performance benefits, it's important to consider certain aspects and best practices:
-
Memory Management: Since off-heap memory is not managed by the JVM, it requires careful memory management to prevent memory leaks and fragmentation. Proper resource cleanup and lifecycle management are crucial.
-
Data Consistency: While off-heap concurrent counters provide efficient access, ensuring data consistency in highly concurrent environments is paramount. Carefully design access patterns and consider the implications of eventual consistency.
-
Tuning and Monitoring: Monitoring off-heap memory usage and performance is essential. Tuning parameters such as maximum entries, memory-mapped file configurations, and eviction policies can significantly impact the behavior of off-heap data structures.
The Bottom Line
In this article, we've explored the concept of off-heap memory in Java and delved into the implementation of off-heap concurrent counters using Chronicle-Map. By leveraging off-heap memory for managing concurrent counters, we can achieve significant performance improvements and scalability in applications with high concurrency requirements.
Off-heap memory management, when used judiciously, can be a powerful tool for optimizing performance-critical aspects of Java applications. However, it's essential to strike a balance between the benefits of off-heap memory and the additional complexity it introduces, considering factors such as data consistency, memory management, and monitoring.
By mastering the use of off-heap concurrent counters, Java developers can unlock the full potential of their applications in terms of performance, scalability, and efficient concurrent data access.
Remember, always measure the performance impact of off-heap solutions in your specific application context, and continually optimize based on empirical evidence.
Chronicle-Map GitHub repository: https://github.com/OpenHFT/Chronicle-Map
For further reading on off-heap memory management and Java performance optimization, check out this comprehensive guide on Understanding Java Off-Heap Memory: Understanding Java Off-Heap Memory
Happy coding and optimizing for performance!
Checkout our other articles