Making Microservices Local: A Guide to First-Class Procedures
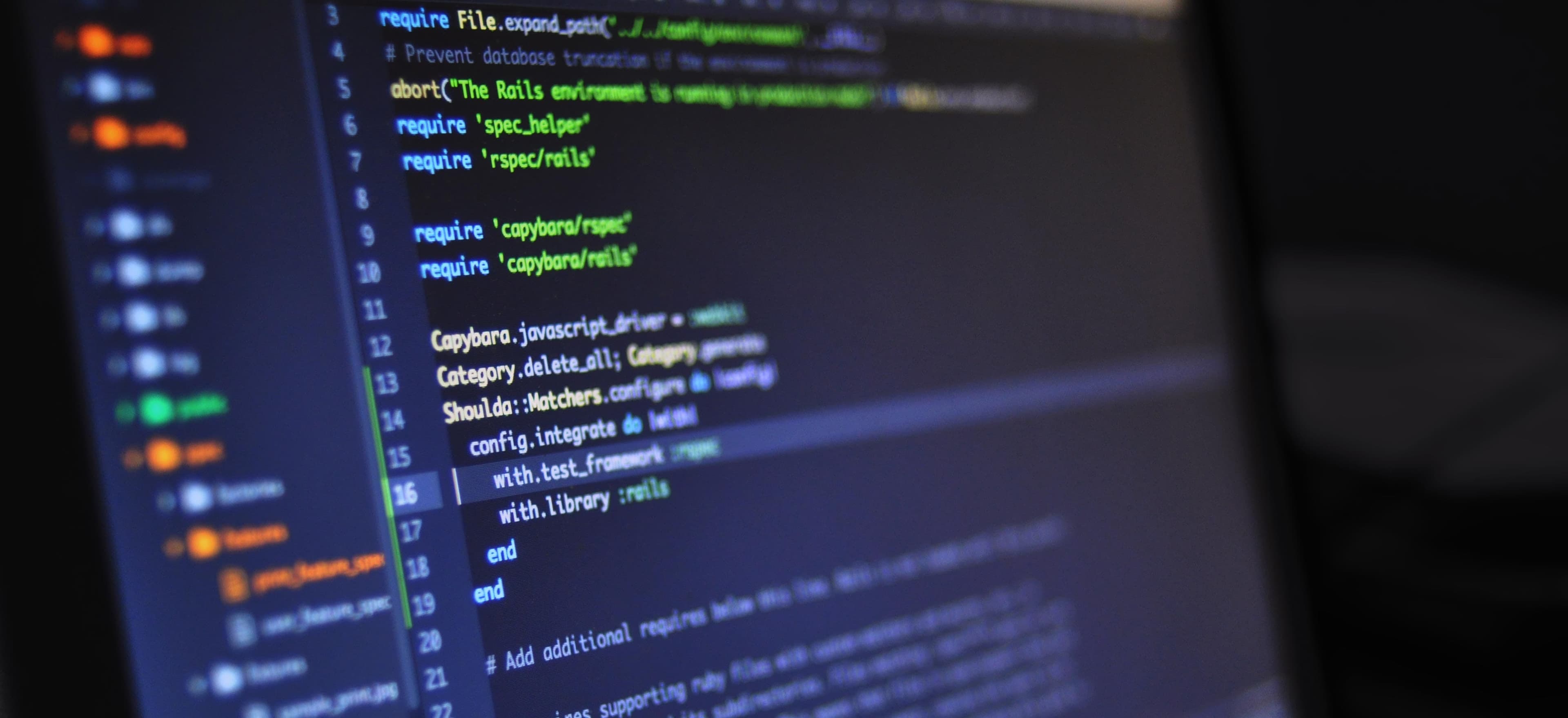
- Published on
Making Microservices Local: A Guide to First-Class Procedures
In the realm of modern software development, the concept of microservices has emerged as a game-changer. Microservices architecture allows developers to build and maintain large, complex applications with greater flexibility, scalability, and resilience. However, as with any innovative approach, implementing microservices requires careful consideration of various factors, including development, testing, and deployment. This post delves into the significance of making microservices local through the lens of Java, focusing on empowering developers to establish first-class procedures for building, testing, and running microservices effectively.
Understanding Microservices
Microservices are an architectural style in which a large application is constructed as a collection of small, independent services. Each service operates in its own process and communicates with other services through lightweight mechanisms, often an HTTP/REST API. This approach enables teams to work on different services concurrently, use the best technologies for each service, and scale individual services as needed.
Challenges with Microservices Development
While the advantages of microservices are significant, they also come with inherent challenges, particularly in the development phase. Coordinating the development of multiple microservices can be complex, as each service may have its own database or external dependencies. Additionally, ensuring that services interact seamlessly with one another requires rigorous testing and continuous integration. Consequently, setting up a robust development environment with proper testing procedures becomes crucial.
Local Development of Microservices with Java
Java has long been a stalwart in enterprise development, and its ecosystem offers a rich array of tools and libraries that are well-suited to building and testing microservices. By focusing on local development procedures using Java, teams can streamline the process of creating and testing microservices. Let's explore a comprehensive set of best practices and tools for local development of microservices in Java.
1. Utilizing Docker for Local Development
Docker provides a convenient way to package and distribute applications, along with their dependencies, as lightweight containers. Leveraging Docker for local development allows developers to replicate the production environment on their workstations, ensuring consistent behavior across different environments. By creating Docker containers for each microservice, developers can minimize the "it works on my machine" problem and guarantee a consistent development experience for the entire team.
Example of using Docker in local development:
// Dockerfile for a Java microservice
FROM openjdk:11-jre-slim
WORKDIR /app
COPY target/microservice.jar /app
CMD ["java", "-jar", "microservice.jar"]
2. Implementing Comprehensive Testing Automation
Testing microservices in isolation as well as in conjunction with other services is paramount. Utilizing testing frameworks such as JUnit and Mockito, along with tools like Postman for API testing, enables developers to ensure the stability and reliability of their services. Automated testing procedures also play a pivotal role in maintaining the integrity of microservices when changes or updates are introduced.
Example of a unit test using JUnit:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class UserServiceTest {
@Test
public void testGetUserById() {
UserService userService = new UserService();
User user = userService.getUserById(123);
assertEquals("John Doe", user.getName());
}
}
3. Embracing Continuous Integration and Delivery (CI/CD)
Incorporating CI/CD practices into the development workflow ensures that changes to microservices are validated and deployed swiftly. Tools such as Jenkins, Travis CI, or GitLab CI/CD can automate the build, test, and deployment processes. Continuous integration enables developers to merge their code frequently, triggering automated builds and tests to catch issues early. Meanwhile, continuous delivery facilitates the release of changes to production or staging environments with minimal human intervention.
Example of a Jenkinsfile for a simple CI/CD pipeline:
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn clean package'
}
}
stage('Test') {
steps {
sh 'mvn test'
}
}
stage('Deploy') {
steps {
sh 'kubectl apply -f deployment.yaml'
}
}
}
}
4. Monitoring and Logging for Local Development
Efficient local development also demands visibility into the behavior of microservices. Integrating tools like Prometheus for metrics and Grafana for visualization can empower developers to monitor the performance and health of their services. Furthermore, logging frameworks such as Log4j or Logback allow developers to capture and analyze relevant information during local development, helping to identify and troubleshoot issues effectively.
In Conclusion
Developing and testing microservices locally with Java entails a comprehensive approach that encompasses containerization, thorough testing, continuous integration and delivery, as well as robust monitoring and logging. By adopting these first-class procedures, teams can mitigate the complexities inherent in microservices development and achieve a smoother, more efficient workflow. Embracing the powerful tools and practices available within the Java ecosystem equips developers to tackle the intricacies of microservices with confidence, paving the way for the creation of robust, scalable, and resilient applications.
Get ready to excel in your microservice development journey with Java!
Remember, always brighten the coder in you!
The above content is intended for informational purposes only and should not be considered as professional advice. It is recommended to independently validate and verify the information presented in this article.
Checkout our other articles