Overcoming Duplicate Natural ID Errors in Hibernate
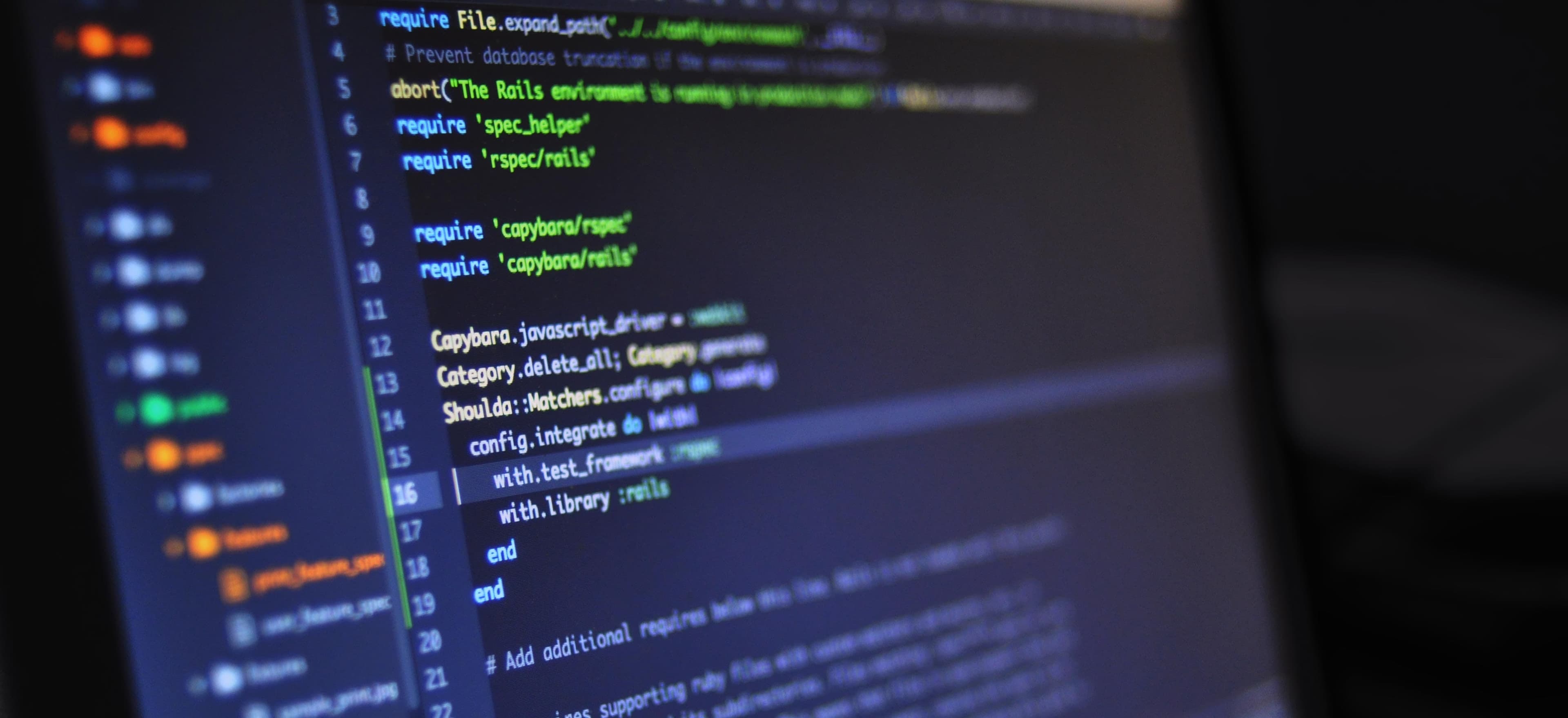
- Published on
Overcoming Duplicate Natural ID Errors in Hibernate
When working with Hibernate, it's common to encounter the issue of duplicate natural identifiers (natural IDs) for entities. This problem arises when you attempt to insert or update data that violates the uniqueness constraint of a natural ID in your database. Luckily, there are several ways to handle this issue effectively in Hibernate. In this blog post, we'll explore the concept of natural IDs, the causes of duplicate natural ID errors, and how to overcome them using different strategies.
Understanding Natural IDs in Hibernate
In Hibernate, a natural identifier (natural ID) is a property or combination of properties that uniquely identify an entity, without the need for an artificial identifier such as a primary key. Natural IDs are often used to represent business keys or unique attributes of an entity in the domain model. Hibernate provides support for defining and utilizing natural IDs through the @NaturalId
annotation, allowing you to enforce uniqueness constraints at the database level.
Causes of Duplicate Natural ID Errors
Duplicate natural ID errors typically occur when attempting to insert or update an entity with a natural ID that already exists in the database. This can happen due to various reasons, including concurrent transactions, improper handling of uniqueness constraints in the application code, or inconsistent session states. When Hibernate detects a duplicate natural ID violation, it throws a ConstraintViolationException
, indicating the failure to persist the entity due to the violation of the natural ID constraint.
Strategies for Overcoming Duplicate Natural ID Errors
1. Session merge()
method
One approach to handling duplicate natural ID errors is to use the merge()
method provided by the Hibernate Session
. When you encounter a ConstraintViolationException
while persisting an entity, you can catch the exception, perform a merge operation, and then re-attempt the transaction. The merge()
method reattaches a detached entity to the current persistence context, resolving any conflicts with existing persistent entities.
try {
// Attempt to persist the entity
session.save(entity);
} catch (ConstraintViolationException e) {
// Handle the duplicate natural ID error
entity = (Entity) session.merge(entity);
// Re-attempt the transaction
session.save(entity);
}
2. Optimistic Locking with Versioning
Another effective strategy involves utilizing optimistic locking with versioning. By adding a version attribute to your entity and enabling optimistic locking through the @Version
annotation, Hibernate can automatically detect and prevent concurrent updates to entities with the same natural ID. When a concurrent transaction attempts to update an entity with the same natural ID, Hibernate will throw an OptimisticLockException
, allowing you to handle the conflict gracefully.
@Entity
public class Entity {
@Id
@GeneratedValue
private Long id;
@NaturalId
private String naturalId;
@Version
private Long version;
// other entity properties and methods
}
3. Custom Error Handling and Retry Policies
To enhance resilience against duplicate natural ID errors, you can implement custom error handling and retry policies within your application. By encapsulating the persistence logic in a service layer, you can intercept ConstraintViolationException
instances and apply retry mechanisms such as exponential backoff, circuit breakers, or retry with increasing delays. This proactive approach can mitigate the impact of transient database conflicts and improve the overall reliability of your application.
@Service
public class EntityService {
@Transactional
public void saveEntityWithRetry(Entity entity) {
try {
// Attempt to persist the entity
entityManager.persist(entity);
} catch (ConstraintViolationException e) {
// Apply custom retry logic
retrySaveWithBackoff(entity);
}
}
private void retrySaveWithBackoff(Entity entity) {
// Exponential backoff and retry logic implementation
}
}
The Last Word
In conclusion, dealing with duplicate natural ID errors in Hibernate requires a proactive and strategic approach. By understanding the nature of natural IDs, identifying the causes of duplicate errors, and applying effective mitigation strategies such as session merging, optimistic locking, and custom error handling, you can ensure the integrity and reliability of your Hibernate-based applications.
By employing these techniques, you can effectively manage duplicate natural ID errors and maintain the consistency of your database while leveraging the power and flexibility of Hibernate.
To gain further insights into advanced Hibernate features and best practices, consider exploring the official Hibernate documentation and community resources.
Remember, mastering the handling of duplicate natural ID errors not only enhances your skills as a Java developer but also contributes to the robustness and stability of your applications.
Checkout our other articles